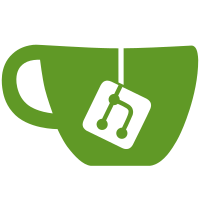
1 changed files with 68 additions and 67 deletions
@ -1,67 +1,68 @@ |
|||||
using System; |
using System; |
||||
using System.Collections.Generic; |
using System.Collections.Generic; |
||||
using System.Linq; |
using System.Linq; |
||||
using System.Threading.Tasks; |
using System.Threading.Tasks; |
||||
using CoviDok.Data.MySQL; |
using CoviDok.Data.MySQL; |
||||
using CoviDok.Data.SessionProviders; |
using CoviDok.Data.SessionProviders; |
||||
using CoviDok.Data.StorageProviders; |
using CoviDok.Data.StorageProviders; |
||||
using Microsoft.AspNetCore.Builder; |
using Microsoft.AspNetCore.Builder; |
||||
using Microsoft.AspNetCore.Hosting; |
using Microsoft.AspNetCore.Hosting; |
||||
using Microsoft.AspNetCore.Http; |
using Microsoft.AspNetCore.Http; |
||||
using Microsoft.AspNetCore.Mvc; |
using Microsoft.AspNetCore.Mvc; |
||||
using Microsoft.Extensions.Configuration; |
using Microsoft.Extensions.Configuration; |
||||
using Microsoft.Extensions.DependencyInjection; |
using Microsoft.Extensions.DependencyInjection; |
||||
using Microsoft.Extensions.Hosting; |
using Microsoft.Extensions.Hosting; |
||||
|
|
||||
namespace CoviDok |
namespace CoviDok |
||||
{ |
{ |
||||
public class Startup |
public class Startup |
||||
{ |
{ |
||||
// This method gets called by the runtime. Use this method to add services to the container.
|
// This method gets called by the runtime. Use this method to add services to the container.
|
||||
// For more information on how to configure your application, visit https://go.microsoft.com/fwlink/?LinkID=398940
|
// For more information on how to configure your application, visit https://go.microsoft.com/fwlink/?LinkID=398940
|
||||
private readonly IConfiguration _configuration; |
private readonly IConfiguration _configuration; |
||||
|
|
||||
public Startup(IConfiguration configuration) |
public Startup(IConfiguration configuration) |
||||
{ |
{ |
||||
_configuration = configuration; |
_configuration = configuration; |
||||
} |
} |
||||
|
|
||||
public void ConfigureServices(IServiceCollection services) |
public void ConfigureServices(IServiceCollection services) |
||||
{ |
{ |
||||
services.AddMvc(options => options.EnableEndpointRouting = false).SetCompatibilityVersion(CompatibilityVersion.Version_3_0); |
services.AddMvc(options => options.EnableEndpointRouting = false).SetCompatibilityVersion(CompatibilityVersion.Version_3_0); |
||||
services.AddSingleton<IConfiguration>(_configuration); |
services.AddSingleton<IConfiguration>(_configuration); |
||||
MySqlContext.MySqlString = _configuration.GetConnectionString("MySQLDatabase"); |
MySqlContext.MySqlString = _configuration.GetConnectionString("MySQLDatabase"); |
||||
RedisProvider.Host = _configuration.GetConnectionString("RedisHost"); |
RedisProvider.Host = _configuration.GetConnectionString("RedisHost"); |
||||
MinioProvider._settings = new MinioSettings { |
MinioProvider._settings = new MinioSettings { |
||||
HostName = _configuration["MinioSettings:HostName"], |
HostName = _configuration["MinioSettings:HostName"], |
||||
AccessKey = _configuration["MinioSettings:AccessKey"], |
AccessKey = _configuration["MinioSettings:AccessKey"], |
||||
SecretKey = _configuration["MinioSettings:SecretKey"] |
SecretKey = _configuration["MinioSettings:SecretKey"] |
||||
}; |
}; |
||||
} |
Console.WriteLine("Minio host is" + MinioProvider._settings.HostName); |
||||
|
} |
||||
// This method gets called by the runtime. Use this method to configure the HTTP request pipeline.
|
|
||||
public void Configure(IApplicationBuilder app, IWebHostEnvironment env) |
// This method gets called by the runtime. Use this method to configure the HTTP request pipeline.
|
||||
{ |
public void Configure(IApplicationBuilder app, IWebHostEnvironment env) |
||||
if (env.IsDevelopment()) |
{ |
||||
{ |
if (env.IsDevelopment()) |
||||
app.UseDeveloperExceptionPage(); |
{ |
||||
} |
app.UseDeveloperExceptionPage(); |
||||
|
} |
||||
//app.UseRouting();
|
|
||||
|
//app.UseRouting();
|
||||
app.UseMvc(); |
|
||||
|
app.UseMvc(); |
||||
|
|
||||
MySqlContext ctx = new MySqlContext(); |
|
||||
ctx.Database.EnsureCreated(); |
MySqlContext ctx = new MySqlContext(); |
||||
|
ctx.Database.EnsureCreated(); |
||||
//app.UseEndpoints(endpoints =>
|
|
||||
//{
|
//app.UseEndpoints(endpoints =>
|
||||
// endpoints.MapGet("/", async context =>
|
//{
|
||||
// {
|
// endpoints.MapGet("/", async context =>
|
||||
// await context.Response.WriteAsync("Hello World!");
|
// {
|
||||
// });
|
// await context.Response.WriteAsync("Hello World!");
|
||||
//});
|
// });
|
||||
} |
//});
|
||||
} |
} |
||||
} |
} |
||||
|
} |
||||
|
Loading…
Reference in new issue