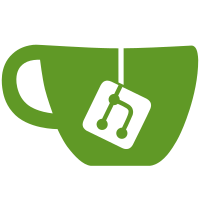
8 changed files with 711 additions and 722 deletions
@ -1,55 +1,56 @@ |
|||
using System; |
|||
using System.Collections.Generic; |
|||
using System.Text; |
|||
using System.Text.Json; |
|||
using System.Threading.Tasks; |
|||
using CoviDok.Api; |
|||
using CoviDok.Data.SessionProviders; |
|||
using NCuid; |
|||
|
|||
namespace CoviDok.BLL.Sessions |
|||
{ |
|||
class SessionHandler |
|||
{ |
|||
private readonly ISessionProvider SessionStore; |
|||
|
|||
public SessionHandler() |
|||
{ |
|||
SessionStore = new RedisProvider(); |
|||
} |
|||
|
|||
public async Task<Session> GetSession(string SessionId) |
|||
{ |
|||
string Candidate = SessionStore.Get(SessionId); |
|||
if (Candidate == null) return null; |
|||
Session session = null; |
|||
await Task.Run(() => { |
|||
session = JsonSerializer.Deserialize<Session>(Candidate); |
|||
session.LastAccess = DateTime.Now; |
|||
SessionStore.Set(SessionId, JsonSerializer.Serialize(session)); |
|||
}); |
|||
return session; |
|||
} |
|||
|
|||
public async Task<string> CreateSession(Role UserType, int UserId) |
|||
{ |
|||
string Id = null; |
|||
await Task.Run(() => { |
|||
Session session = new Session |
|||
{ |
|||
Id = UserId, |
|||
Type = UserType, |
|||
LastAccess = DateTime.Now |
|||
}; |
|||
Id = Cuid.Generate(); |
|||
SessionStore.Set(Id, JsonSerializer.Serialize(session)); |
|||
}); |
|||
return Id; |
|||
} |
|||
|
|||
public void DeleteSession(string SessionId) |
|||
{ |
|||
SessionStore.Del(SessionId); |
|||
} |
|||
} |
|||
} |
|||
using System; |
|||
using System.Collections.Generic; |
|||
using System.Text; |
|||
using System.Text.Json; |
|||
using System.Threading.Tasks; |
|||
using CoviDok.Api; |
|||
using CoviDok.Data.SessionProviders; |
|||
using NCuid; |
|||
|
|||
namespace CoviDok.BLL.Sessions |
|||
{ |
|||
class SessionHandler |
|||
{ |
|||
private readonly ISessionProvider SessionStore; |
|||
|
|||
public SessionHandler() |
|||
{ |
|||
SessionStore = new RedisProvider(); |
|||
} |
|||
|
|||
public async Task<Session> GetSession(string SessionId) |
|||
{ |
|||
if (string.IsNullOrEmpty(SessionId)) throw new UnauthorizedAccessException(); |
|||
string Candidate = SessionStore.Get(SessionId); |
|||
if (Candidate == null) throw new UnauthorizedAccessException(); |
|||
Session session = null; |
|||
await Task.Run(() => { |
|||
session = JsonSerializer.Deserialize<Session>(Candidate); |
|||
session.LastAccess = DateTime.Now; |
|||
SessionStore.Set(SessionId, JsonSerializer.Serialize(session)); |
|||
}); |
|||
return session; |
|||
} |
|||
|
|||
public async Task<string> CreateSession(Role UserType, int UserId) |
|||
{ |
|||
string Id = null; |
|||
await Task.Run(() => { |
|||
Session session = new Session |
|||
{ |
|||
Id = UserId, |
|||
Type = UserType, |
|||
LastAccess = DateTime.Now |
|||
}; |
|||
Id = Cuid.Generate(); |
|||
SessionStore.Set(Id, JsonSerializer.Serialize(session)); |
|||
}); |
|||
return Id; |
|||
} |
|||
|
|||
public void DeleteSession(string SessionId) |
|||
{ |
|||
SessionStore.Del(SessionId); |
|||
} |
|||
} |
|||
} |
|||
|
@ -1,61 +1,56 @@ |
|||
using System; |
|||
using System.Collections.Generic; |
|||
using System.Linq; |
|||
using System.Threading.Tasks; |
|||
using CoviDok.Api.Objects; |
|||
using CoviDok.BLL.Sessions; |
|||
using CoviDok.BLL.User.Managers; |
|||
using CoviDok.Data.MySQL; |
|||
using CoviDok.Data.SessionProviders; |
|||
using Microsoft.AspNetCore.Http; |
|||
using Microsoft.AspNetCore.Mvc; |
|||
|
|||
namespace CoviDok.Controllers |
|||
{ |
|||
[Route("api/[controller]")]
|
|||
[ApiController] |
|||
public class AssistantController : ControllerBase |
|||
{ |
|||
private readonly SessionHandler Handler = new SessionHandler(); |
|||
|
|||
private readonly AssistantManager mgr = new AssistantManager(); |
|||
|
|||
|
|||
[HttpGet("{id}")] |
|||
public async Task<ActionResult<PublicAssistant>> GetAssistant(int id) |
|||
{ |
|||
try |
|||
{ |
|||
return await mgr.GetAssistant(id); |
|||
} |
|||
catch (KeyNotFoundException) |
|||
{ |
|||
return NotFound(); |
|||
} |
|||
} |
|||
|
|||
[HttpPut("{id}")] |
|||
public async Task<IActionResult> PutAssistant(int id, PublicAssistant ast) |
|||
{ |
|||
Session s = await Handler.GetSession(ast.SessionId); |
|||
if (s == null) return Unauthorized(); |
|||
try |
|||
{ |
|||
await mgr.UpdateAssistant(s, id, ast); |
|||
return NoContent(); |
|||
} |
|||
catch (UnauthorizedAccessException) |
|||
{ |
|||
return Unauthorized(); |
|||
} |
|||
catch (KeyNotFoundException) |
|||
{ |
|||
return NotFound(); |
|||
} |
|||
catch (FormatException) |
|||
{ |
|||
return BadRequest(); |
|||
} |
|||
} |
|||
} |
|||
} |
|||
using System; |
|||
using System.Collections.Generic; |
|||
using System.Linq; |
|||
using System.Threading.Tasks; |
|||
using CoviDok.Api.Objects; |
|||
using CoviDok.BLL.Sessions; |
|||
using CoviDok.BLL.User.Managers; |
|||
using CoviDok.Data.MySQL; |
|||
using CoviDok.Data.SessionProviders; |
|||
using Microsoft.AspNetCore.Http; |
|||
using Microsoft.AspNetCore.Mvc; |
|||
|
|||
namespace CoviDok.Controllers |
|||
{ |
|||
[Route("api/[controller]")]
|
|||
[ApiController] |
|||
public class AssistantController : ControllerBase |
|||
{ |
|||
private readonly SessionHandler Handler = new SessionHandler(); |
|||
|
|||
private readonly AssistantManager mgr = new AssistantManager(); |
|||
|
|||
|
|||
[HttpGet("{id}")] |
|||
public async Task<ActionResult<PublicAssistant>> GetAssistant(int id) |
|||
{ |
|||
try |
|||
{ |
|||
return await mgr.GetAssistant(id); |
|||
} |
|||
catch (KeyNotFoundException) |
|||
{ |
|||
return NotFound(); |
|||
} |
|||
} |
|||
|
|||
[HttpPut("{id}")] |
|||
public async Task<IActionResult> PutAssistant(int id, PublicAssistant ast) |
|||
{ |
|||
try |
|||
{ |
|||
Session s = await Handler.GetSession(ast.SessionId); |
|||
await mgr.UpdateAssistant(s, id, ast); |
|||
return NoContent(); |
|||
} |
|||
catch (UnauthorizedAccessException) |
|||
{ |
|||
return Unauthorized(); |
|||
} |
|||
catch (KeyNotFoundException) |
|||
{ |
|||
return NotFound(); |
|||
} |
|||
} |
|||
} |
|||
} |
|||
|
@ -1,186 +1,175 @@ |
|||
using System; |
|||
using System.Collections; |
|||
using System.Collections.Generic; |
|||
using System.Linq; |
|||
using System.Threading.Tasks; |
|||
using CoviDok.Api; |
|||
using CoviDok.Api.Request; |
|||
using CoviDok.Api.Response; |
|||
using CoviDok.BLL; |
|||
using CoviDok.BLL.Sessions; |
|||
using CoviDok.BLL.User.Managers; |
|||
using CoviDok.Data.Model; |
|||
using CoviDok.Data.SessionProviders; |
|||
using Microsoft.AspNetCore.Http; |
|||
using Microsoft.AspNetCore.Mvc; |
|||
|
|||
namespace CoviDok.Controllers |
|||
{ |
|||
[Route("api/[controller]")]
|
|||
[ApiController] |
|||
public class CaseController : ControllerBase |
|||
{ |
|||
private readonly SessionHandler Handler = new SessionHandler(); |
|||
private readonly CaseManager mgr = new CaseManager(); |
|||
|
|||
// POST /api/Case/{id}
|
|||
[HttpPost("{id}")] |
|||
public async Task<ActionResult<Case>> PostGetCase(int id, string SessionId) |
|||
{ |
|||
Session s = await Handler.GetSession(SessionId); |
|||
if (s == null) return Unauthorized(); |
|||
|
|||
try { |
|||
return await mgr.GetCase(s, id); |
|||
} |
|||
catch (KeyNotFoundException) |
|||
{ |
|||
return NotFound(); |
|||
} |
|||
catch (UnauthorizedAccessException) |
|||
{ |
|||
return Unauthorized(); |
|||
} |
|||
} |
|||
|
|||
// POST /api/Case/{id}/update
|
|||
|
|||
[HttpPut("{id}/update")] |
|||
public async Task<IActionResult> PostUpdate(int id, CaseUpdate data) |
|||
{ |
|||
Session s = await Handler.GetSession(data.SessionId); |
|||
if (s == null) return Unauthorized(); |
|||
try |
|||
{ |
|||
await mgr.UpdateCase(s, id, data.UpdateMsg, data.Images); |
|||
return Ok(); |
|||
} |
|||
catch (UnauthorizedAccessException) |
|||
{ |
|||
return Unauthorized(); |
|||
} |
|||
catch (KeyNotFoundException) { |
|||
return NotFound(); |
|||
} |
|||
catch (InvalidOperationException) |
|||
{ |
|||
return BadRequest(); |
|||
} |
|||
|
|||
} |
|||
|
|||
[HttpPost] |
|||
public async Task<ActionResult<Case>> NewCase(CaseCreate data) |
|||
{ |
|||
Session s = await Handler.GetSession(data.SessionId); |
|||
if (s == null) return Unauthorized(); |
|||
|
|||
try |
|||
{ |
|||
Case c = await mgr.CreateCase(s, data.DoctorId, data.ChildId, data.Title, data.StartDate); |
|||
return CreatedAtAction("PostGetCase", new { id = c.Id }, c); |
|||
} |
|||
catch (UnauthorizedAccessException) |
|||
{ |
|||
return Unauthorized(); |
|||
} |
|||
} |
|||
|
|||
[HttpPost("{id}/updates")] |
|||
public async Task<ActionResult<List<Update>>> GetUpdatesForCase(int id, string SessionId) |
|||
{ |
|||
Session s = await Handler.GetSession(SessionId); |
|||
if (s == null) return Unauthorized(); |
|||
try { |
|||
return await mgr.GetUpdatesForCase(s, id); |
|||
} |
|||
catch (UnauthorizedAccessException) |
|||
{ |
|||
return Unauthorized(); |
|||
} |
|||
catch (KeyNotFoundException) |
|||
{ |
|||
return NotFound(); |
|||
} |
|||
} |
|||
|
|||
[HttpPost("updates/{id}")] |
|||
public async Task<ActionResult<Update>> GetUpdate(int id, string SessionId) |
|||
{ |
|||
Session s = await Handler.GetSession(SessionId); |
|||
if (s == null) return Unauthorized(); |
|||
try |
|||
{ |
|||
return await mgr.GetUpdate(s, id); |
|||
} |
|||
catch (UnauthorizedAccessException) |
|||
{ |
|||
return Unauthorized(); |
|||
} |
|||
catch (KeyNotFoundException) |
|||
{ |
|||
return NotFound(); |
|||
} |
|||
} |
|||
|
|||
// POST /api/Case/{id}/close
|
|||
[HttpPost("{id}/close")] |
|||
public async Task<IActionResult> PostClose(int id, string SessionId) |
|||
{ |
|||
Session s = await Handler.GetSession(SessionId); |
|||
if (s == null) return Unauthorized(); |
|||
try |
|||
{ |
|||
await mgr.SetCertified(s, id); |
|||
return Ok(); |
|||
} |
|||
catch (UnauthorizedAccessException) |
|||
{ |
|||
return Unauthorized(); |
|||
} |
|||
catch (KeyNotFoundException) |
|||
{ |
|||
return NotFound(); |
|||
} |
|||
} |
|||
// POST /api/Case/{id}/close
|
|||
[HttpPost("{id}/cure")] |
|||
public async Task<IActionResult> PostCured(int id, string SessionId) |
|||
{ |
|||
Session s = await Handler.GetSession(SessionId); |
|||
if (s == null) return Unauthorized(); |
|||
try |
|||
{ |
|||
await mgr.SetCured(s, id); |
|||
return Ok(); |
|||
} |
|||
catch (UnauthorizedAccessException) |
|||
{ |
|||
return Unauthorized(); |
|||
} |
|||
catch (KeyNotFoundException) |
|||
{ |
|||
return NotFound(); |
|||
} |
|||
} |
|||
|
|||
// POST /api/Case/filter
|
|||
[HttpPost("filter")] |
|||
public async Task<ActionResult<List<Case>>> Filter(CaseFilter filters) |
|||
{ |
|||
Session s = await Handler.GetSession(filters.SessionId); |
|||
if (s == null) return Unauthorized(); |
|||
|
|||
try |
|||
{ |
|||
return await mgr.FilterCases(s, filters); |
|||
} |
|||
catch (UnauthorizedAccessException) |
|||
{ |
|||
return Unauthorized(); |
|||
} |
|||
|
|||
} |
|||
|
|||
} |
|||
} |
|||
using System; |
|||
using System.Collections; |
|||
using System.Collections.Generic; |
|||
using System.Linq; |
|||
using System.Threading.Tasks; |
|||
using CoviDok.Api; |
|||
using CoviDok.Api.Request; |
|||
using CoviDok.Api.Response; |
|||
using CoviDok.BLL; |
|||
using CoviDok.BLL.Sessions; |
|||
using CoviDok.BLL.User.Managers; |
|||
using CoviDok.Data.Model; |
|||
using CoviDok.Data.SessionProviders; |
|||
using Microsoft.AspNetCore.Http; |
|||
using Microsoft.AspNetCore.Mvc; |
|||
|
|||
namespace CoviDok.Controllers |
|||
{ |
|||
[Route("api/[controller]")]
|
|||
[ApiController] |
|||
public class CaseController : ControllerBase |
|||
{ |
|||
private readonly SessionHandler Handler = new SessionHandler(); |
|||
private readonly CaseManager mgr = new CaseManager(); |
|||
|
|||
// POST /api/Case/{id}
|
|||
[HttpPost("{id}")] |
|||
public async Task<ActionResult<Case>> PostGetCase(int id, string SessionId) |
|||
{ |
|||
try { |
|||
Session s = await Handler.GetSession(SessionId); |
|||
return await mgr.GetCase(s, id); |
|||
} |
|||
catch (KeyNotFoundException) |
|||
{ |
|||
return NotFound(); |
|||
} |
|||
catch (UnauthorizedAccessException) |
|||
{ |
|||
return Unauthorized(); |
|||
} |
|||
} |
|||
|
|||
// POST /api/Case/{id}/update
|
|||
|
|||
[HttpPut("{id}/update")] |
|||
public async Task<IActionResult> PostUpdate(int id, CaseUpdate data) |
|||
{ |
|||
try |
|||
{ |
|||
Session s = await Handler.GetSession(data.SessionId); |
|||
await mgr.UpdateCase(s, id, data.UpdateMsg, data.Images); |
|||
return Ok(); |
|||
} |
|||
catch (UnauthorizedAccessException) |
|||
{ |
|||
return Unauthorized(); |
|||
} |
|||
catch (KeyNotFoundException) { |
|||
return NotFound(); |
|||
} |
|||
catch (InvalidOperationException) |
|||
{ |
|||
return BadRequest(); |
|||
} |
|||
|
|||
} |
|||
|
|||
[HttpPost] |
|||
public async Task<ActionResult<Case>> NewCase(CaseCreate data) |
|||
{ |
|||
try |
|||
{ |
|||
Session s = await Handler.GetSession(data.SessionId); |
|||
Case c = await mgr.CreateCase(s, data.DoctorId, data.ChildId, data.Title, data.StartDate); |
|||
return CreatedAtAction("PostGetCase", new { id = c.Id }, c); |
|||
} |
|||
catch (UnauthorizedAccessException) |
|||
{ |
|||
return Unauthorized(); |
|||
} |
|||
} |
|||
|
|||
[HttpPost("{id}/updates")] |
|||
public async Task<ActionResult<List<Update>>> GetUpdatesForCase(int id, string SessionId) |
|||
{ |
|||
try { |
|||
Session s = await Handler.GetSession(SessionId); |
|||
return await mgr.GetUpdatesForCase(s, id); |
|||
} |
|||
catch (UnauthorizedAccessException) |
|||
{ |
|||
return Unauthorized(); |
|||
} |
|||
catch (KeyNotFoundException) |
|||
{ |
|||
return NotFound(); |
|||
} |
|||
} |
|||
|
|||
[HttpPost("updates/{id}")] |
|||
public async Task<ActionResult<Update>> GetUpdate(int id, string SessionId) |
|||
{ |
|||
try |
|||
{ |
|||
Session s = await Handler.GetSession(SessionId); |
|||
return await mgr.GetUpdate(s, id); |
|||
} |
|||
catch (UnauthorizedAccessException) |
|||
{ |
|||
return Unauthorized(); |
|||
} |
|||
catch (KeyNotFoundException) |
|||
{ |
|||
return NotFound(); |
|||
} |
|||
} |
|||
|
|||
// POST /api/Case/{id}/close
|
|||
[HttpPost("{id}/close")] |
|||
public async Task<IActionResult> PostClose(int id, string SessionId) |
|||
{ |
|||
try |
|||
{ |
|||
Session s = await Handler.GetSession(SessionId); |
|||
await mgr.SetCertified(s, id); |
|||
return Ok(); |
|||
} |
|||
catch (UnauthorizedAccessException) |
|||
{ |
|||
return Unauthorized(); |
|||
} |
|||
catch (KeyNotFoundException) |
|||
{ |
|||
return NotFound(); |
|||
} |
|||
} |
|||
// POST /api/Case/{id}/close
|
|||
[HttpPost("{id}/cure")] |
|||
public async Task<IActionResult> PostCured(int id, string SessionId) |
|||
{ |
|||
try |
|||
{ |
|||
Session s = await Handler.GetSession(SessionId); |
|||
await mgr.SetCured(s, id); |
|||
return Ok(); |
|||
} |
|||
catch (UnauthorizedAccessException) |
|||
{ |
|||
return Unauthorized(); |
|||
} |
|||
catch (KeyNotFoundException) |
|||
{ |
|||
return NotFound(); |
|||
} |
|||
} |
|||
|
|||
// POST /api/Case/filter
|
|||
[HttpPost("filter")] |
|||
public async Task<ActionResult<List<Case>>> Filter(CaseFilter filters) |
|||
{ |
|||
try |
|||
{ |
|||
Session s = await Handler.GetSession(filters.SessionId); |
|||
return await mgr.FilterCases(s, filters); |
|||
} |
|||
catch (UnauthorizedAccessException) |
|||
{ |
|||
return Unauthorized(); |
|||
} |
|||
|
|||
} |
|||
|
|||
} |
|||
} |
|||
|
@ -1,95 +1,96 @@ |
|||
using System; |
|||
using System.Collections.Generic; |
|||
using System.Linq; |
|||
using System.Threading.Tasks; |
|||
using Microsoft.AspNetCore.Http; |
|||
using Microsoft.AspNetCore.Mvc; |
|||
using CoviDok.Api.Objects; |
|||
using CoviDok.Api.Request; |
|||
using CoviDok.BLL.User.Managers; |
|||
using CoviDok.BLL.Sessions; |
|||
using CoviDok.Data.SessionProviders; |
|||
using CoviDok.Data.MySQL; |
|||
|
|||
namespace CoviDok.Controllers |
|||
{ |
|||
[Route("api/[controller]")]
|
|||
[ApiController] |
|||
public class ChildController : ControllerBase |
|||
{ |
|||
private readonly SessionHandler Handler = new SessionHandler(); |
|||
|
|||
private readonly ChildManager ChildManager = new ChildManager(); |
|||
|
|||
// POST: api/Child/5
|
|||
[HttpPost("{id}")] |
|||
public async Task<ActionResult<PublicChild>> GetPublicChild(int id, string SessionId) |
|||
{ |
|||
Session s = await Handler.GetSession(SessionId); |
|||
if (s == null) return Unauthorized(); |
|||
|
|||
try |
|||
{ |
|||
return await ChildManager.GetChild(s, id); |
|||
} |
|||
catch (UnauthorizedAccessException) |
|||
{ |
|||
return Unauthorized(); |
|||
} |
|||
catch (KeyNotFoundException) |
|||
{ |
|||
return NotFound(); |
|||
} |
|||
} |
|||
|
|||
// PUT: api/Child/5
|
|||
// To protect from overposting attacks, enable the specific properties you want to bind to, for
|
|||
// more details, see https://go.microsoft.com/fwlink/?linkid=2123754.
|
|||
[HttpPut("{id}")] |
|||
public async Task<IActionResult> PutPublicChild(int id, PublicChild publicChild) |
|||
{ |
|||
Session s = await Handler.GetSession(publicChild.SessionId); |
|||
if (s == null) return Unauthorized(); |
|||
try { |
|||
await ChildManager.UpdateChild(s, id, publicChild); |
|||
return NoContent(); |
|||
} |
|||
catch (UnauthorizedAccessException) { |
|||
return Unauthorized(); |
|||
} |
|||
catch (KeyNotFoundException) { |
|||
return NotFound(); |
|||
} |
|||
catch (FormatException) |
|||
{ |
|||
return BadRequest(); |
|||
} |
|||
} |
|||
|
|||
[HttpPost("parent")] |
|||
public async Task<ActionResult<List<PublicChild>>> GetChildrenOfParent(string SessionId) |
|||
{ |
|||
Session s = await Handler.GetSession(SessionId); |
|||
if (s == null) return Unauthorized(); |
|||
return ChildManager.ChildrenOfParent(s.Id); |
|||
} |
|||
|
|||
// POST: api/Child
|
|||
// To protect from overposting attacks, enable the specific properties you want to bind to, for
|
|||
// more details, see https://go.microsoft.com/fwlink/?linkid=2123754.
|
|||
[HttpPost] |
|||
public async Task<ActionResult<PublicChild>> PostPublicChild(PublicChild publicChild) |
|||
{ |
|||
Session s = await Handler.GetSession(publicChild.SessionId); |
|||
if (s == null ) return Unauthorized(); |
|||
|
|||
try { |
|||
int Id = await ChildManager.AddChild(s, publicChild); |
|||
return CreatedAtAction("GetPublicChild", new { id = Id }, publicChild); |
|||
} |
|||
catch (UnauthorizedAccessException) { |
|||
return Unauthorized(); |
|||
} |
|||
} |
|||
} |
|||
} |
|||
using System; |
|||
using System.Collections.Generic; |
|||
using System.Linq; |
|||
using System.Threading.Tasks; |
|||
using Microsoft.AspNetCore.Http; |
|||
using Microsoft.AspNetCore.Mvc; |
|||
using CoviDok.Api.Objects; |
|||
using CoviDok.Api.Request; |
|||
using CoviDok.BLL.User.Managers; |
|||
using CoviDok.BLL.Sessions; |
|||
using CoviDok.Data.SessionProviders; |
|||
using CoviDok.Data.MySQL; |
|||
|
|||
namespace CoviDok.Controllers |
|||
{ |
|||
[Route("api/[controller]")]
|
|||
[ApiController] |
|||
public class ChildController : ControllerBase |
|||
{ |
|||
private readonly SessionHandler Handler = new SessionHandler(); |
|||
|
|||
private readonly ChildManager ChildManager = new ChildManager(); |
|||
|
|||
// POST: api/Child/5
|
|||
[HttpPost("{id}")] |
|||
public async Task<ActionResult<PublicChild>> GetPublicChild(int id, string SessionId) |
|||
{ |
|||
try |
|||
{ |
|||
Session s = await Handler.GetSession(SessionId); |
|||
return await ChildManager.GetChild(s, id); |
|||
} |
|||
catch (UnauthorizedAccessException) |
|||
{ |
|||
return Unauthorized(); |
|||
} |
|||
catch (KeyNotFoundException) |
|||
{ |
|||
return NotFound(); |
|||
} |
|||
} |
|||
|
|||
// PUT: api/Child/5
|
|||
// To protect from overposting attacks, enable the specific properties you want to bind to, for
|
|||
// more details, see https://go.microsoft.com/fwlink/?linkid=2123754.
|
|||
[HttpPut("{id}")] |
|||
public async Task<IActionResult> PutPublicChild(int id, PublicChild publicChild) |
|||
{ |
|||
try { |
|||
Session s = await Handler.GetSession(publicChild.SessionId); |
|||
await ChildManager.UpdateChild(s, id, publicChild); |
|||
return NoContent(); |
|||
} |
|||
catch (UnauthorizedAccessException) { |
|||
return Unauthorized(); |
|||
} |
|||
catch (KeyNotFoundException) { |
|||
return NotFound(); |
|||
} |
|||
catch (FormatException) |
|||
{ |
|||
return BadRequest(); |
|||
} |
|||
} |
|||
|
|||
[HttpPost("parent")] |
|||
public async Task<ActionResult<List<PublicChild>>> GetChildrenOfParent(string SessionId) |
|||
{ |
|||
try { |
|||
Session s = await Handler.GetSession(SessionId); |
|||
return ChildManager.ChildrenOfParent(s.Id); |
|||
} |
|||
catch (UnauthorizedAccessException) |
|||
{ |
|||
return Unauthorized(); |
|||
} |
|||
|
|||
} |
|||
|
|||
// POST: api/Child
|
|||
// To protect from overposting attacks, enable the specific properties you want to bind to, for
|
|||
// more details, see https://go.microsoft.com/fwlink/?linkid=2123754.
|
|||
[HttpPost] |
|||
public async Task<ActionResult<PublicChild>> PostPublicChild(PublicChild publicChild) |
|||
{ |
|||
try { |
|||
Session s = await Handler.GetSession(publicChild.SessionId); |
|||
int Id = await ChildManager.AddChild(s, publicChild); |
|||
return CreatedAtAction("GetPublicChild", new { id = Id }, publicChild); |
|||
} |
|||
catch (UnauthorizedAccessException) { |
|||
return Unauthorized(); |
|||
} |
|||
} |
|||
} |
|||
} |
|||
|
@ -1,117 +1,116 @@ |
|||
using System; |
|||
using System.Collections.Generic; |
|||
using System.Linq; |
|||
using System.Threading.Tasks; |
|||
using CoviDok.Api; |
|||
using CoviDok.Api.Objects; |
|||
using CoviDok.Api.Request; |
|||
using CoviDok.BLL.Sessions; |
|||
using CoviDok.BLL.User.Managers; |
|||
using CoviDok.Data.MySQL; |
|||
using CoviDok.Data.SessionProviders; |
|||
using Microsoft.AspNetCore.Http; |
|||
using Microsoft.AspNetCore.Mvc; |
|||
|
|||
namespace CoviDok.Controllers |
|||
{ |
|||
[Route("api/[controller]")]
|
|||
[ApiController] |
|||
public class DoctorController : ControllerBase |
|||
{ |
|||
private readonly SessionHandler Handler = new SessionHandler(); |
|||
|
|||
private readonly DoctorManager doctorHandler = new DoctorManager(); |
|||
// GET /api/Doc
|
|||
[HttpGet] |
|||
public async Task<ActionResult<ICollection<PublicDoctor>>> GetDoctors() |
|||
{ |
|||
return await doctorHandler.GetDoctors(); |
|||
} |
|||
|
|||
[HttpGet("byFirstName/{firstName}")] |
|||
public async Task<ActionResult<ICollection<PublicDoctor>>> GetDoctorsByFirstName(string firstName) |
|||
{ |
|||
return await doctorHandler.GetDoctors(firstName: firstName); |
|||
} |
|||
[HttpGet("byLastName/{lastName}")] |
|||
public async Task<ActionResult<ICollection<PublicDoctor>>> GetDoctorsByLastName(string lastName) |
|||
{ |
|||
return await doctorHandler.GetDoctors(lastName: lastName); |
|||
} |
|||
|
|||
[HttpGet("byName/{firstName}/{lastName}")] |
|||
public async Task<ActionResult<ICollection<PublicDoctor>>> GetDoctorByName(string firstName, string lastName) |
|||
{ |
|||
return await doctorHandler.GetDoctors(lastName: lastName, firstName: firstName); |
|||
} |
|||
|
|||
[HttpGet("{id}")] |
|||
public async Task<ActionResult<PublicDoctor>> GetDoctor(int id) |
|||
{ |
|||
try |
|||
{ |
|||
return await doctorHandler.GetDoctor(id); |
|||
} |
|||
catch (KeyNotFoundException) |
|||
{ |
|||
return NotFound(); |
|||
} |
|||
} |
|||
|
|||
[HttpPut("{id}")] |
|||
public async Task<IActionResult> PutDoctor(int id, PublicDoctor doctor) { |
|||
Session s = await Handler.GetSession(doctor.SessionId); |
|||
if (s == null) return Unauthorized(); |
|||
try |
|||
{ |
|||
await doctorHandler.UpdateDoctor(s, id, doctor); |
|||
return NoContent(); |
|||
} |
|||
catch (UnauthorizedAccessException) |
|||
{ |
|||
return Unauthorized(); |
|||
} |
|||
catch (KeyNotFoundException) |
|||
{ |
|||
return NotFound(); |
|||
} |
|||
catch (FormatException) |
|||
{ |
|||
return BadRequest(); |
|||
} |
|||
} |
|||
|
|||
// GET /api/Doc/{id}/assistants
|
|||
[HttpGet("{id}/assistants")] |
|||
public async Task<ActionResult<ICollection<PublicAssistant>>> GetAssistantsOfDoctor(int id) |
|||
{ |
|||
try |
|||
{ |
|||
return await doctorHandler.GetAssistants(id); |
|||
} |
|||
catch (KeyNotFoundException) |
|||
{ |
|||
return NotFound(); |
|||
} |
|||
} |
|||
|
|||
// GET /api/Doc/{id}/children
|
|||
[HttpPost("{id}/children")] |
|||
public async Task<ActionResult<ICollection<PublicChild>>> GetChildrenOfDoctor(int id, string SessionId) |
|||
{ |
|||
Session s = await Handler.GetSession(SessionId); |
|||
if (s == null) return Unauthorized(); |
|||
try |
|||
{ |
|||
return await doctorHandler.GetChildren(id); |
|||
} |
|||
catch (KeyNotFoundException) |
|||
{ |
|||
return NotFound(); |
|||
} |
|||
catch (UnauthorizedAccessException) { |
|||
return Unauthorized(); |
|||
} |
|||
} |
|||
} |
|||
} |
|||
using System; |
|||
using System.Collections.Generic; |
|||
using System.Linq; |
|||
using System.Threading.Tasks; |
|||
using CoviDok.Api; |
|||
using CoviDok.Api.Objects; |
|||
using CoviDok.Api.Request; |
|||
using CoviDok.BLL.Sessions; |
|||
using CoviDok.BLL.User.Managers; |
|||
using CoviDok.Data.MySQL; |
|||
using CoviDok.Data.SessionProviders; |
|||
using Microsoft.AspNetCore.Http; |
|||
using Microsoft.AspNetCore.Mvc; |
|||
|
|||
namespace CoviDok.Controllers |
|||
{ |
|||
[Route("api/[controller]")]
|
|||
[ApiController] |
|||
public class DoctorController : ControllerBase |
|||
{ |
|||
private readonly SessionHandler Handler = new SessionHandler(); |
|||
|
|||
private readonly DoctorManager doctorHandler = new DoctorManager(); |
|||
// GET /api/Doc
|
|||
[HttpGet] |
|||
public async Task<ActionResult<ICollection<PublicDoctor>>> GetDoctors() |
|||
{ |
|||
return await doctorHandler.GetDoctors(); |
|||
} |
|||
|
|||
[HttpGet("byFirstName/{firstName}")] |
|||
public async Task<ActionResult<ICollection<PublicDoctor>>> GetDoctorsByFirstName(string firstName) |
|||
{ |
|||
return await doctorHandler.GetDoctors(firstName: firstName); |
|||
} |
|||
[HttpGet("byLastName/{lastName}")] |
|||
public async Task<ActionResult<ICollection<PublicDoctor>>> GetDoctorsByLastName(string lastName) |
|||
{ |
|||
return await doctorHandler.GetDoctors(lastName: lastName); |
|||
} |
|||
|
|||
[HttpGet("byName/{firstName}/{lastName}")] |
|||
public async Task<ActionResult<ICollection<PublicDoctor>>> GetDoctorByName(string firstName, string lastName) |
|||
{ |
|||
return await doctorHandler.GetDoctors(lastName: lastName, firstName: firstName); |
|||
} |
|||
|
|||
[HttpGet("{id}")] |
|||
public async Task<ActionResult<PublicDoctor>> GetDoctor(int id) |
|||
{ |
|||
try |
|||
{ |
|||
return await doctorHandler.GetDoctor(id); |
|||
} |
|||
catch (KeyNotFoundException) |
|||
{ |
|||
return NotFound(); |
|||
} |
|||
} |
|||
|
|||
[HttpPut("{id}")] |
|||
public async Task<IActionResult> PutDoctor(int id, PublicDoctor doctor) { |
|||
Session s = await Handler.GetSession(doctor.SessionId); |
|||
if (s == null) return Unauthorized(); |
|||
try |
|||
{ |
|||
await doctorHandler.UpdateDoctor(s, id, doctor); |
|||
return NoContent(); |
|||
} |
|||
catch (UnauthorizedAccessException) |
|||
{ |
|||
return Unauthorized(); |
|||
} |
|||
catch (KeyNotFoundException) |
|||
{ |
|||
return NotFound(); |
|||
} |
|||
catch (FormatException) |
|||
{ |
|||
return BadRequest(); |
|||
} |
|||
} |
|||
|
|||
// GET /api/Doc/{id}/assistants
|
|||
[HttpGet("{id}/assistants")] |
|||
public async Task<ActionResult<ICollection<PublicAssistant>>> GetAssistantsOfDoctor(int id) |
|||
{ |
|||
try |
|||
{ |
|||
return await doctorHandler.GetAssistants(id); |
|||
} |
|||
catch (KeyNotFoundException) |
|||
{ |
|||
return NotFound(); |
|||
} |
|||
} |
|||
|
|||
// GET /api/Doc/{id}/children
|
|||
[HttpPost("{id}/children")] |
|||
public async Task<ActionResult<ICollection<PublicChild>>> GetChildrenOfDoctor(int id, string SessionId) |
|||
{ |
|||
try |
|||
{ |
|||
Session s = await Handler.GetSession(SessionId); |
|||
return await doctorHandler.GetChildren(id); |
|||
} |
|||
catch (KeyNotFoundException) |
|||
{ |
|||
return NotFound(); |
|||
} |
|||
catch (UnauthorizedAccessException) { |
|||
return Unauthorized(); |
|||
} |
|||
} |
|||
} |
|||
} |
|||
|
@ -1,88 +1,91 @@ |
|||
using System; |
|||
using System.Collections.Generic; |
|||
using System.IO; |
|||
using System.Linq; |
|||
using System.Security.Cryptography.X509Certificates; |
|||
using System.Threading.Tasks; |
|||
using CoviDok.Api; |
|||
using CoviDok.Api.Request; |
|||
using CoviDok.BLL; |
|||
using CoviDok.BLL.Sessions; |
|||
using CoviDok.BLL.Storage; |
|||
using CoviDok.Data.SessionProviders; |
|||
using CoviDok.Data.StorageProviders; |
|||
using Microsoft.AspNetCore.Http; |
|||
using Microsoft.AspNetCore.Mvc; |
|||
using Microsoft.Extensions.Configuration; |
|||
using Microsoft.Extensions.Options; |
|||
using NCuid; |
|||
|
|||
namespace CoviDok.Controllers |
|||
{ |
|||
[Route("api/[controller]")]
|
|||
[ApiController] |
|||
public class ImagesController : ControllerBase |
|||
{ |
|||
private StorageHandler MinioHandler = new StorageHandler(new MinioProvider()); |
|||
private readonly string BucketName = "test1"; |
|||
|
|||
private readonly SessionHandler Handler = new SessionHandler(); |
|||
|
|||
private static Stream MakeStream(string s) |
|||
{ |
|||
var stream = new MemoryStream(); |
|||
var writer = new StreamWriter(stream); |
|||
writer.Write(s); |
|||
writer.Flush(); |
|||
stream.Position = 0; |
|||
return stream; |
|||
} |
|||
|
|||
[HttpPost("Upload")] |
|||
public async Task<ActionResult<GenericResponse>> OnPostImage(ImagePost post) |
|||
{ |
|||
GenericResponse response = new GenericResponse(); |
|||
Session s = await Handler.GetSession(post.SessionId); |
|||
if (s == null) |
|||
{ |
|||
response.Status = Status.Error; |
|||
response.Body["reason"] = "unauthorized"; |
|||
return response; |
|||
} |
|||
StorageResult Result = await MinioHandler.UploadImage(BucketName, MakeStream(post.File), post.File.Length, Cuid.Generate()); |
|||
if (!Result.Success) response.Status = Status.Error; |
|||
response.Body["reason"] = Result.Data; |
|||
|
|||
return response; |
|||
} |
|||
|
|||
[HttpPost("Download")] |
|||
public async Task<GenericResponse> OnGetImage(ImageGet imageGet) |
|||
{ |
|||
GenericResponse response = new GenericResponse(); |
|||
Session s = await Handler.GetSession(imageGet.SessionId); |
|||
if (s == null) |
|||
{ |
|||
response.Status = Status.Error; |
|||
response.Body["reason"] = "unauthorized"; |
|||
return response; |
|||
} |
|||
try { |
|||
string res = null; |
|||
await MinioHandler.GetImage(BucketName, imageGet.ImageId, (stream) => { |
|||
StreamReader reader = new StreamReader(stream); |
|||
res = reader.ReadToEnd(); |
|||
}); |
|||
|
|||
response.Body["image"] = res; |
|||
return response; |
|||
} |
|||
catch (KeyNotFoundException) |
|||
{ |
|||
response.Status = Status.Error; |
|||
response.Body["reason"] = "Image not found!"; |
|||
return response; |
|||
} |
|||
} |
|||
} |
|||
} |
|||
using System; |
|||
using System.Collections.Generic; |
|||
using System.IO; |
|||
using System.Linq; |
|||
using System.Security.Cryptography.X509Certificates; |
|||
using System.Threading.Tasks; |
|||
using CoviDok.Api; |
|||
using CoviDok.Api.Request; |
|||
using CoviDok.BLL; |
|||
using CoviDok.BLL.Sessions; |
|||
using CoviDok.BLL.Storage; |
|||
using CoviDok.Data.SessionProviders; |
|||
using CoviDok.Data.StorageProviders; |
|||
using Microsoft.AspNetCore.Http; |
|||
using Microsoft.AspNetCore.Mvc; |
|||
using Microsoft.Extensions.Configuration; |
|||
using Microsoft.Extensions.Options; |
|||
using NCuid; |
|||
|
|||
namespace CoviDok.Controllers |
|||
{ |
|||
[Route("api/[controller]")]
|
|||
[ApiController] |
|||
public class ImagesController : ControllerBase |
|||
{ |
|||
private StorageHandler MinioHandler = new StorageHandler(new MinioProvider()); |
|||
private readonly string BucketName = "test1"; |
|||
|
|||
private readonly SessionHandler Handler = new SessionHandler(); |
|||
|
|||
private static Stream MakeStream(string s) |
|||
{ |
|||
var stream = new MemoryStream(); |
|||
var writer = new StreamWriter(stream); |
|||
writer.Write(s); |
|||
writer.Flush(); |
|||
stream.Position = 0; |
|||
return stream; |
|||
} |
|||
|
|||
[HttpPost("Upload")] |
|||
public async Task<ActionResult<GenericResponse>> OnPostImage(ImagePost post) |
|||
{ |
|||
GenericResponse response = new GenericResponse(); |
|||
Session s = await Handler.GetSession(post.SessionId); |
|||
if (s == null) |
|||
{ |
|||
response.Status = Status.Error; |
|||
response.Body["reason"] = "unauthorized"; |
|||
return response; |
|||
} |
|||
StorageResult Result = await MinioHandler.UploadImage(BucketName, MakeStream(post.File), post.File.Length, Cuid.Generate()); |
|||
if (!Result.Success) response.Status = Status.Error; |
|||
response.Body["reason"] = Result.Data; |
|||
|
|||
return response; |
|||
} |
|||
|
|||
[HttpPost("Download")] |
|||
public async Task<GenericResponse> OnGetImage(ImageGet imageGet) |
|||
{ |
|||
GenericResponse response = new GenericResponse(); |
|||
try |
|||
{ |
|||
Session s = await Handler.GetSession(imageGet.SessionId); |
|||
string res = null; |
|||
await MinioHandler.GetImage(BucketName, imageGet.ImageId, (stream) => |
|||
{ |
|||
StreamReader reader = new StreamReader(stream); |
|||
res = reader.ReadToEnd(); |
|||
}); |
|||
|
|||
response.Body["image"] = res; |
|||
return response; |
|||
} |
|||
catch (UnauthorizedAccessException) |
|||
{ |
|||
response.Status = Status.Error; |
|||
response.Body["reason"] = "unauthorized"; |
|||
return response; |
|||
} |
|||
|
|||
catch (KeyNotFoundException) |
|||
{ |
|||
response.Status = Status.Error; |
|||
response.Body["reason"] = "Image not found!"; |
|||
return response; |
|||
} |
|||
} |
|||
} |
|||
} |
|||
|
@ -1,79 +1,80 @@ |
|||
using System; |
|||
using System.Collections.Generic; |
|||
using System.Linq; |
|||
using System.Threading.Tasks; |
|||
using CoviDok.Api.Objects; |
|||
using CoviDok.Api.Request; |
|||
using CoviDok.BLL.Sessions; |
|||
using CoviDok.BLL.User.Managers; |
|||
using CoviDok.Data.MySQL; |
|||
using CoviDok.Data.SessionProviders; |
|||
using Microsoft.AspNetCore.Http; |
|||
using Microsoft.AspNetCore.Mvc; |
|||
|
|||
namespace CoviDok.Controllers |
|||
{ |
|||
[Route("api/[controller]")]
|
|||
[ApiController] |
|||
public class ParentController : ControllerBase |
|||
{ |
|||
private readonly SessionHandler sessionHandler = new SessionHandler(); |
|||
|
|||
private readonly ParentManager parentManager = new ParentManager(); |
|||
|
|||
[HttpPost("{id}")] |
|||
public async Task<ActionResult<PublicParent>> GetParent(int id, string SessionId) |
|||
{ |
|||
Session s = await sessionHandler.GetSession(SessionId); |
|||
if (s == null) return Unauthorized(); |
|||
try { |
|||
return await parentManager.GetParent(id); |
|||
} |
|||
catch (KeyNotFoundException) |
|||
{ |
|||
return NotFound(); |
|||
} |
|||
} |
|||
|
|||
[HttpPut("{id}")] |
|||
public async Task<IActionResult> PutParent(int id, PublicParent parent) |
|||
{ |
|||
Session s = await sessionHandler.GetSession(parent.SessionId); |
|||
if (s == null) return Unauthorized(); |
|||
try { |
|||
await parentManager.UpdateParent(s, id, parent); |
|||
return NoContent(); |
|||
} |
|||
catch (UnauthorizedAccessException) |
|||
{ |
|||
return Unauthorized(); |
|||
} |
|||
catch (KeyNotFoundException) |
|||
{ |
|||
return NotFound(); |
|||
} |
|||
catch (FormatException) |
|||
{ |
|||
return BadRequest(); |
|||
} |
|||
} |
|||
[HttpPost("{id}/children")] |
|||
public async Task<ActionResult<List<PublicChild>>> GetChildrenOfParent(int id, string SessionId) |
|||
{ |
|||
Session s = await sessionHandler.GetSession(SessionId); |
|||
if (s == null) return Unauthorized(); |
|||
try |
|||
{ |
|||
return await parentManager.GetChildren(id); |
|||
} |
|||
catch (KeyNotFoundException) |
|||
{ |
|||
return NotFound(); |
|||
} |
|||
catch (UnauthorizedAccessException) |
|||
{ |
|||
return Unauthorized(); |
|||
} |
|||
} |
|||
} |
|||
} |
|||
using System; |
|||
using System.Collections.Generic; |
|||
using System.Linq; |
|||
using System.Threading.Tasks; |
|||
using CoviDok.Api.Objects; |
|||
using CoviDok.Api.Request; |
|||
using CoviDok.BLL.Sessions; |
|||
using CoviDok.BLL.User.Managers; |
|||
using CoviDok.Data.MySQL; |
|||
using CoviDok.Data.SessionProviders; |
|||
using Microsoft.AspNetCore.Http; |
|||
using Microsoft.AspNetCore.Mvc; |
|||
|
|||
namespace CoviDok.Controllers |
|||
{ |
|||
[Route("api/[controller]")]
|
|||
[ApiController] |
|||
public class ParentController : ControllerBase |
|||
{ |
|||
private readonly SessionHandler sessionHandler = new SessionHandler(); |
|||
|
|||
private readonly ParentManager parentManager = new ParentManager(); |
|||
|
|||
[HttpPost("{id}")] |
|||
public async Task<ActionResult<PublicParent>> GetParent(int id, string SessionId) |
|||
{ |
|||
try { |
|||
Session s = await sessionHandler.GetSession(SessionId); |
|||
return await parentManager.GetParent(id); |
|||
} |
|||
catch (UnauthorizedAccessException) |
|||
{ |
|||
return Unauthorized(); |
|||
} |
|||
catch (KeyNotFoundException) |
|||
{ |
|||
return NotFound(); |
|||
} |
|||
} |
|||
|
|||
[HttpPut("{id}")] |
|||
public async Task<IActionResult> PutParent(int id, PublicParent parent) |
|||
{ |
|||
try { |
|||
Session s = await sessionHandler.GetSession(parent.SessionId); |
|||
await parentManager.UpdateParent(s, id, parent); |
|||
return NoContent(); |
|||
} |
|||
catch (UnauthorizedAccessException) |
|||
{ |
|||
return Unauthorized(); |
|||
} |
|||
catch (KeyNotFoundException) |
|||
{ |
|||
return NotFound(); |
|||
} |
|||
catch (FormatException) |
|||
{ |
|||
return BadRequest(); |
|||
} |
|||
} |
|||
[HttpPost("{id}/children")] |
|||
public async Task<ActionResult<List<PublicChild>>> GetChildrenOfParent(int id, string SessionId) |
|||
{ |
|||
try |
|||
{ |
|||
Session s = await sessionHandler.GetSession(SessionId); |
|||
return await parentManager.GetChildren(id); |
|||
} |
|||
catch (KeyNotFoundException) |
|||
{ |
|||
return NotFound(); |
|||
} |
|||
catch (UnauthorizedAccessException) |
|||
{ |
|||
return Unauthorized(); |
|||
} |
|||
} |
|||
} |
|||
} |
|||
|
@ -1,41 +1,41 @@ |
|||
# Default values for covidok. |
|||
# This is a YAML-formatted file. |
|||
# Declare variables to be passed into your templates. |
|||
|
|||
images: |
|||
covidok: |
|||
name: docker.local/bme/covidok |
|||
tag: latest |
|||
mysql: |
|||
name: mysql |
|||
tag: 8.0 |
|||
minio: |
|||
name: minio/minio |
|||
tag: latest |
|||
redis: |
|||
name: redis |
|||
tag: 6 |
|||
pullPolicy: Always |
|||
# Overrides the image tag whose default is the chart appVersion. |
|||
|
|||
config: |
|||
minio: |
|||
accesskey: "accesskey" |
|||
secretkey: "secretkey" |
|||
mysql: |
|||
database: "covidok" |
|||
user: "covdiok" |
|||
password: "covidok" |
|||
redis: |
|||
port: "6379" |
|||
|
|||
|
|||
imagePullSecrets: [] |
|||
nameOverride: "" |
|||
fullnameOverride: "" |
|||
|
|||
podAnnotations: {} |
|||
|
|||
service: |
|||
type: ClusterIP |
|||
port: 80 |
|||
# Default values for covidok. |
|||
# This is a YAML-formatted file. |
|||
# Declare variables to be passed into your templates. |
|||
|
|||
images: |
|||
covidok: |
|||
name: docker.local/bme/covidok |
|||
tag: latest |
|||
mysql: |
|||
name: mysql |
|||
tag: 8.0 |
|||
minio: |
|||
name: minio/minio |
|||
tag: latest |
|||
redis: |
|||
name: redis |
|||
tag: 6 |
|||
pullPolicy: Always |
|||
# Overrides the image tag whose default is the chart appVersion. |
|||
|
|||
config: |
|||
minio: |
|||
accesskey: "accesskey" |
|||
secretkey: "secretkey" |
|||
mysql: |
|||
database: "covidok" |
|||
user: "covidok" |
|||
password: "covidok" |
|||
redis: |
|||
port: "6379" |
|||
|
|||
|
|||
imagePullSecrets: [] |
|||
nameOverride: "" |
|||
fullnameOverride: "" |
|||
|
|||
podAnnotations: {} |
|||
|
|||
service: |
|||
type: ClusterIP |
|||
port: 80 |
|||
|
Loading…
Reference in new issue