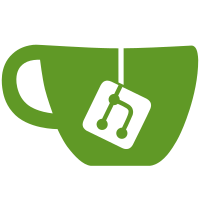
7 changed files with 168 additions and 9 deletions
@ -0,0 +1,123 @@ |
|||
using System; |
|||
using System.Collections.Generic; |
|||
using System.Linq; |
|||
using System.Threading.Tasks; |
|||
using Microsoft.AspNetCore.Http; |
|||
using Microsoft.AspNetCore.Mvc; |
|||
using Microsoft.EntityFrameworkCore; |
|||
using CoviDok.data; |
|||
|
|||
namespace CoviDok.Controllers |
|||
{ |
|||
[Route("api/[controller]")]
|
|||
[ApiController] |
|||
public class DoctorsController : ControllerBase |
|||
{ |
|||
private readonly MySQLContext _context; |
|||
|
|||
public DoctorsController(MySQLContext context) |
|||
{ |
|||
_context = context; |
|||
} |
|||
|
|||
// GET: api/Doctors
|
|||
[HttpGet] |
|||
public async Task<ActionResult<IEnumerable<Doctor>>> GetDoctors() |
|||
{ |
|||
return await _context.Doctors.ToListAsync(); |
|||
} |
|||
|
|||
// GET: api/Doctors/5
|
|||
[HttpGet("{id}")] |
|||
public async Task<ActionResult<Doctor>> GetDoctor(string id) |
|||
{ |
|||
var doctor = await _context.Doctors.FindAsync(id); |
|||
|
|||
if (doctor == null) |
|||
{ |
|||
return NotFound(); |
|||
} |
|||
|
|||
return doctor; |
|||
} |
|||
|
|||
// PUT: api/Doctors/5
|
|||
// To protect from overposting attacks, enable the specific properties you want to bind to, for
|
|||
// more details, see https://go.microsoft.com/fwlink/?linkid=2123754.
|
|||
[HttpPut("{id}")] |
|||
public async Task<IActionResult> PutDoctor(string id, Doctor doctor) |
|||
{ |
|||
if (id != doctor.Id) |
|||
{ |
|||
return BadRequest(); |
|||
} |
|||
|
|||
_context.Entry(doctor).State = EntityState.Modified; |
|||
|
|||
try |
|||
{ |
|||
await _context.SaveChangesAsync(); |
|||
} |
|||
catch (DbUpdateConcurrencyException) |
|||
{ |
|||
if (!DoctorExists(id)) |
|||
{ |
|||
return NotFound(); |
|||
} |
|||
else |
|||
{ |
|||
throw; |
|||
} |
|||
} |
|||
|
|||
return NoContent(); |
|||
} |
|||
|
|||
// POST: api/Doctors
|
|||
// To protect from overposting attacks, enable the specific properties you want to bind to, for
|
|||
// more details, see https://go.microsoft.com/fwlink/?linkid=2123754.
|
|||
[HttpPost] |
|||
public async Task<ActionResult<Doctor>> PostDoctor(Doctor doctor) |
|||
{ |
|||
_context.Doctors.Add(doctor); |
|||
try |
|||
{ |
|||
await _context.SaveChangesAsync(); |
|||
} |
|||
catch (DbUpdateException) |
|||
{ |
|||
if (DoctorExists(doctor.Id)) |
|||
{ |
|||
return Conflict(); |
|||
} |
|||
else |
|||
{ |
|||
throw; |
|||
} |
|||
} |
|||
|
|||
return CreatedAtAction("GetDoctor", new { id = doctor.Id }, doctor); |
|||
} |
|||
|
|||
// DELETE: api/Doctors/5
|
|||
[HttpDelete("{id}")] |
|||
public async Task<ActionResult<Doctor>> DeleteDoctor(string id) |
|||
{ |
|||
var doctor = await _context.Doctors.FindAsync(id); |
|||
if (doctor == null) |
|||
{ |
|||
return NotFound(); |
|||
} |
|||
|
|||
_context.Doctors.Remove(doctor); |
|||
await _context.SaveChangesAsync(); |
|||
|
|||
return doctor; |
|||
} |
|||
|
|||
private bool DoctorExists(string id) |
|||
{ |
|||
return _context.Doctors.Any(e => e.Id == id); |
|||
} |
|||
} |
|||
} |
@ -0,0 +1,23 @@ |
|||
using Microsoft.EntityFrameworkCore; |
|||
using System; |
|||
using System.Collections.Generic; |
|||
using System.Linq; |
|||
using System.Security.Cryptography.X509Certificates; |
|||
using System.Threading.Tasks; |
|||
|
|||
namespace CoviDok.data |
|||
{ |
|||
public class MySQLContext : DbContext |
|||
{ |
|||
public DbSet<Case> Cases { get; set; } |
|||
public DbSet<Child> Children { get; set; } |
|||
public DbSet<Doctor> Doctors { get; set; } |
|||
public DbSet<Image> Images { get; set; } |
|||
public DbSet<Parent> Parents { get; set; } |
|||
public DbSet<Update> Updates { get; set; } |
|||
protected override void OnConfiguring(DbContextOptionsBuilder optionsBuilder) |
|||
{ |
|||
optionsBuilder.UseMySQL("server=localhost;database=library;user=user;password=password"); |
|||
} |
|||
} |
|||
} |
Loading…
Reference in new issue