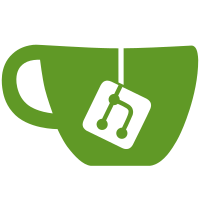
8 changed files with 141 additions and 0 deletions
@ -0,0 +1,24 @@ |
|||
using System; |
|||
using System.Collections.Generic; |
|||
using System.Linq; |
|||
using System.Threading.Tasks; |
|||
|
|||
namespace CoviDok.data |
|||
{ |
|||
public class Assistant |
|||
{ |
|||
public string Id { get; set; } |
|||
|
|||
public string Email { get; set; } |
|||
|
|||
public string Password { get; set; } |
|||
|
|||
public string FirstName { get; set; } |
|||
|
|||
public string LastName { get; set; } |
|||
|
|||
public DateTime RegistrationDate { get; set; } |
|||
|
|||
public string DoctorId { get; set; } |
|||
} |
|||
} |
@ -0,0 +1,20 @@ |
|||
using System; |
|||
using System.Collections.Generic; |
|||
using System.Linq; |
|||
using System.Threading.Tasks; |
|||
|
|||
namespace CoviDok.data |
|||
{ |
|||
public class Case |
|||
{ |
|||
public string DoctorID { get; set; } |
|||
public string ParentID { get; set; } |
|||
public string ChildID { get; set; } |
|||
|
|||
public ICollection<Update> updates = new List<Update>(); |
|||
|
|||
public string Assignee { get; set; } |
|||
|
|||
|
|||
} |
|||
} |
@ -0,0 +1,17 @@ |
|||
using System; |
|||
using System.Collections.Generic; |
|||
using System.Linq; |
|||
using System.Threading.Tasks; |
|||
|
|||
namespace CoviDok.data |
|||
{ |
|||
public class Child |
|||
{ |
|||
public string Id { get; set; } |
|||
public string FirstName { get; set; } |
|||
public string LastName { get; set; } |
|||
public string DoctorId { get; set; } |
|||
public string ParentId { get; set; } |
|||
public ICollection<Case> MedicalHistory { get; } = new List<Case>(); |
|||
} |
|||
} |
@ -0,0 +1,26 @@ |
|||
using System; |
|||
using System.Collections.Generic; |
|||
using System.Linq; |
|||
using System.Threading.Tasks; |
|||
|
|||
namespace CoviDok.data |
|||
{ |
|||
public class Doctor |
|||
{ |
|||
public string Id { get; set; } |
|||
|
|||
public string Email { get; set; } |
|||
|
|||
public string Password { get; set; } |
|||
|
|||
public string FirstName { get; set; } |
|||
|
|||
public string LastName { get; set; } |
|||
|
|||
public DateTime RegistrationDate { get; set; } |
|||
|
|||
public ICollection<Child> Children { get; } = new List<Child>(); |
|||
|
|||
public ICollection<Assistant> Assistants { get; } = new List<Assistant>(); |
|||
} |
|||
} |
@ -0,0 +1,14 @@ |
|||
using System; |
|||
using System.Collections.Generic; |
|||
using System.Linq; |
|||
using System.Threading.Tasks; |
|||
|
|||
namespace CoviDok.data |
|||
{ |
|||
//Store Image ID, get actual content from MinIO backend.
|
|||
public class Image |
|||
{ |
|||
public string ImageID { get; set; } |
|||
|
|||
} |
|||
} |
@ -0,0 +1,24 @@ |
|||
using System; |
|||
using System.Collections.Generic; |
|||
using System.Linq; |
|||
using System.Threading.Tasks; |
|||
|
|||
namespace CoviDok.data |
|||
{ |
|||
public class Parent |
|||
{ |
|||
public string Id { get; set; } |
|||
|
|||
public string Email { get; set; } |
|||
|
|||
public string Password { get; set; } |
|||
|
|||
public string FirstName { get; set; } |
|||
|
|||
public string LastName { get; set; } |
|||
|
|||
public DateTime RegistrationDate { get; set; } |
|||
|
|||
public ICollection<Child> Children { get; } = new List<Child>(); |
|||
} |
|||
} |
@ -0,0 +1,15 @@ |
|||
using System; |
|||
using System.Collections.Generic; |
|||
using System.Linq; |
|||
using System.Threading.Tasks; |
|||
|
|||
namespace CoviDok.data |
|||
{ |
|||
public class Update |
|||
{ |
|||
public string Sender { get; set; } |
|||
public string Content { get; set; } |
|||
|
|||
public ICollection<Image> Images = new List<Image>(); |
|||
} |
|||
} |
Loading…
Reference in new issue