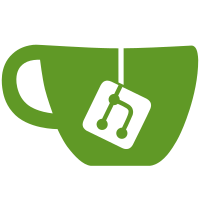
8 changed files with 711 additions and 722 deletions
@ -1,55 +1,56 @@ |
|||||
using System; |
using System; |
||||
using System.Collections.Generic; |
using System.Collections.Generic; |
||||
using System.Text; |
using System.Text; |
||||
using System.Text.Json; |
using System.Text.Json; |
||||
using System.Threading.Tasks; |
using System.Threading.Tasks; |
||||
using CoviDok.Api; |
using CoviDok.Api; |
||||
using CoviDok.Data.SessionProviders; |
using CoviDok.Data.SessionProviders; |
||||
using NCuid; |
using NCuid; |
||||
|
|
||||
namespace CoviDok.BLL.Sessions |
namespace CoviDok.BLL.Sessions |
||||
{ |
{ |
||||
class SessionHandler |
class SessionHandler |
||||
{ |
{ |
||||
private readonly ISessionProvider SessionStore; |
private readonly ISessionProvider SessionStore; |
||||
|
|
||||
public SessionHandler() |
public SessionHandler() |
||||
{ |
{ |
||||
SessionStore = new RedisProvider(); |
SessionStore = new RedisProvider(); |
||||
} |
} |
||||
|
|
||||
public async Task<Session> GetSession(string SessionId) |
public async Task<Session> GetSession(string SessionId) |
||||
{ |
{ |
||||
string Candidate = SessionStore.Get(SessionId); |
if (string.IsNullOrEmpty(SessionId)) throw new UnauthorizedAccessException(); |
||||
if (Candidate == null) return null; |
string Candidate = SessionStore.Get(SessionId); |
||||
Session session = null; |
if (Candidate == null) throw new UnauthorizedAccessException(); |
||||
await Task.Run(() => { |
Session session = null; |
||||
session = JsonSerializer.Deserialize<Session>(Candidate); |
await Task.Run(() => { |
||||
session.LastAccess = DateTime.Now; |
session = JsonSerializer.Deserialize<Session>(Candidate); |
||||
SessionStore.Set(SessionId, JsonSerializer.Serialize(session)); |
session.LastAccess = DateTime.Now; |
||||
}); |
SessionStore.Set(SessionId, JsonSerializer.Serialize(session)); |
||||
return session; |
}); |
||||
} |
return session; |
||||
|
} |
||||
public async Task<string> CreateSession(Role UserType, int UserId) |
|
||||
{ |
public async Task<string> CreateSession(Role UserType, int UserId) |
||||
string Id = null; |
{ |
||||
await Task.Run(() => { |
string Id = null; |
||||
Session session = new Session |
await Task.Run(() => { |
||||
{ |
Session session = new Session |
||||
Id = UserId, |
{ |
||||
Type = UserType, |
Id = UserId, |
||||
LastAccess = DateTime.Now |
Type = UserType, |
||||
}; |
LastAccess = DateTime.Now |
||||
Id = Cuid.Generate(); |
}; |
||||
SessionStore.Set(Id, JsonSerializer.Serialize(session)); |
Id = Cuid.Generate(); |
||||
}); |
SessionStore.Set(Id, JsonSerializer.Serialize(session)); |
||||
return Id; |
}); |
||||
} |
return Id; |
||||
|
} |
||||
public void DeleteSession(string SessionId) |
|
||||
{ |
public void DeleteSession(string SessionId) |
||||
SessionStore.Del(SessionId); |
{ |
||||
} |
SessionStore.Del(SessionId); |
||||
} |
} |
||||
} |
} |
||||
|
} |
||||
|
@ -1,61 +1,56 @@ |
|||||
using System; |
using System; |
||||
using System.Collections.Generic; |
using System.Collections.Generic; |
||||
using System.Linq; |
using System.Linq; |
||||
using System.Threading.Tasks; |
using System.Threading.Tasks; |
||||
using CoviDok.Api.Objects; |
using CoviDok.Api.Objects; |
||||
using CoviDok.BLL.Sessions; |
using CoviDok.BLL.Sessions; |
||||
using CoviDok.BLL.User.Managers; |
using CoviDok.BLL.User.Managers; |
||||
using CoviDok.Data.MySQL; |
using CoviDok.Data.MySQL; |
||||
using CoviDok.Data.SessionProviders; |
using CoviDok.Data.SessionProviders; |
||||
using Microsoft.AspNetCore.Http; |
using Microsoft.AspNetCore.Http; |
||||
using Microsoft.AspNetCore.Mvc; |
using Microsoft.AspNetCore.Mvc; |
||||
|
|
||||
namespace CoviDok.Controllers |
namespace CoviDok.Controllers |
||||
{ |
{ |
||||
[Route("api/[controller]")]
|
[Route("api/[controller]")]
|
||||
[ApiController] |
[ApiController] |
||||
public class AssistantController : ControllerBase |
public class AssistantController : ControllerBase |
||||
{ |
{ |
||||
private readonly SessionHandler Handler = new SessionHandler(); |
private readonly SessionHandler Handler = new SessionHandler(); |
||||
|
|
||||
private readonly AssistantManager mgr = new AssistantManager(); |
private readonly AssistantManager mgr = new AssistantManager(); |
||||
|
|
||||
|
|
||||
[HttpGet("{id}")] |
[HttpGet("{id}")] |
||||
public async Task<ActionResult<PublicAssistant>> GetAssistant(int id) |
public async Task<ActionResult<PublicAssistant>> GetAssistant(int id) |
||||
{ |
{ |
||||
try |
try |
||||
{ |
{ |
||||
return await mgr.GetAssistant(id); |
return await mgr.GetAssistant(id); |
||||
} |
} |
||||
catch (KeyNotFoundException) |
catch (KeyNotFoundException) |
||||
{ |
{ |
||||
return NotFound(); |
return NotFound(); |
||||
} |
} |
||||
} |
} |
||||
|
|
||||
[HttpPut("{id}")] |
[HttpPut("{id}")] |
||||
public async Task<IActionResult> PutAssistant(int id, PublicAssistant ast) |
public async Task<IActionResult> PutAssistant(int id, PublicAssistant ast) |
||||
{ |
{ |
||||
Session s = await Handler.GetSession(ast.SessionId); |
try |
||||
if (s == null) return Unauthorized(); |
{ |
||||
try |
Session s = await Handler.GetSession(ast.SessionId); |
||||
{ |
await mgr.UpdateAssistant(s, id, ast); |
||||
await mgr.UpdateAssistant(s, id, ast); |
return NoContent(); |
||||
return NoContent(); |
} |
||||
} |
catch (UnauthorizedAccessException) |
||||
catch (UnauthorizedAccessException) |
{ |
||||
{ |
return Unauthorized(); |
||||
return Unauthorized(); |
} |
||||
} |
catch (KeyNotFoundException) |
||||
catch (KeyNotFoundException) |
{ |
||||
{ |
return NotFound(); |
||||
return NotFound(); |
} |
||||
} |
} |
||||
catch (FormatException) |
} |
||||
{ |
} |
||||
return BadRequest(); |
|
||||
} |
|
||||
} |
|
||||
} |
|
||||
} |
|
||||
|
@ -1,186 +1,175 @@ |
|||||
using System; |
using System; |
||||
using System.Collections; |
using System.Collections; |
||||
using System.Collections.Generic; |
using System.Collections.Generic; |
||||
using System.Linq; |
using System.Linq; |
||||
using System.Threading.Tasks; |
using System.Threading.Tasks; |
||||
using CoviDok.Api; |
using CoviDok.Api; |
||||
using CoviDok.Api.Request; |
using CoviDok.Api.Request; |
||||
using CoviDok.Api.Response; |
using CoviDok.Api.Response; |
||||
using CoviDok.BLL; |
using CoviDok.BLL; |
||||
using CoviDok.BLL.Sessions; |
using CoviDok.BLL.Sessions; |
||||
using CoviDok.BLL.User.Managers; |
using CoviDok.BLL.User.Managers; |
||||
using CoviDok.Data.Model; |
using CoviDok.Data.Model; |
||||
using CoviDok.Data.SessionProviders; |
using CoviDok.Data.SessionProviders; |
||||
using Microsoft.AspNetCore.Http; |
using Microsoft.AspNetCore.Http; |
||||
using Microsoft.AspNetCore.Mvc; |
using Microsoft.AspNetCore.Mvc; |
||||
|
|
||||
namespace CoviDok.Controllers |
namespace CoviDok.Controllers |
||||
{ |
{ |
||||
[Route("api/[controller]")]
|
[Route("api/[controller]")]
|
||||
[ApiController] |
[ApiController] |
||||
public class CaseController : ControllerBase |
public class CaseController : ControllerBase |
||||
{ |
{ |
||||
private readonly SessionHandler Handler = new SessionHandler(); |
private readonly SessionHandler Handler = new SessionHandler(); |
||||
private readonly CaseManager mgr = new CaseManager(); |
private readonly CaseManager mgr = new CaseManager(); |
||||
|
|
||||
// POST /api/Case/{id}
|
// POST /api/Case/{id}
|
||||
[HttpPost("{id}")] |
[HttpPost("{id}")] |
||||
public async Task<ActionResult<Case>> PostGetCase(int id, string SessionId) |
public async Task<ActionResult<Case>> PostGetCase(int id, string SessionId) |
||||
{ |
{ |
||||
Session s = await Handler.GetSession(SessionId); |
try { |
||||
if (s == null) return Unauthorized(); |
Session s = await Handler.GetSession(SessionId); |
||||
|
return await mgr.GetCase(s, id); |
||||
try { |
} |
||||
return await mgr.GetCase(s, id); |
catch (KeyNotFoundException) |
||||
} |
{ |
||||
catch (KeyNotFoundException) |
return NotFound(); |
||||
{ |
} |
||||
return NotFound(); |
catch (UnauthorizedAccessException) |
||||
} |
{ |
||||
catch (UnauthorizedAccessException) |
return Unauthorized(); |
||||
{ |
} |
||||
return Unauthorized(); |
} |
||||
} |
|
||||
} |
// POST /api/Case/{id}/update
|
||||
|
|
||||
// POST /api/Case/{id}/update
|
[HttpPut("{id}/update")] |
||||
|
public async Task<IActionResult> PostUpdate(int id, CaseUpdate data) |
||||
[HttpPut("{id}/update")] |
{ |
||||
public async Task<IActionResult> PostUpdate(int id, CaseUpdate data) |
try |
||||
{ |
{ |
||||
Session s = await Handler.GetSession(data.SessionId); |
Session s = await Handler.GetSession(data.SessionId); |
||||
if (s == null) return Unauthorized(); |
await mgr.UpdateCase(s, id, data.UpdateMsg, data.Images); |
||||
try |
return Ok(); |
||||
{ |
} |
||||
await mgr.UpdateCase(s, id, data.UpdateMsg, data.Images); |
catch (UnauthorizedAccessException) |
||||
return Ok(); |
{ |
||||
} |
return Unauthorized(); |
||||
catch (UnauthorizedAccessException) |
} |
||||
{ |
catch (KeyNotFoundException) { |
||||
return Unauthorized(); |
return NotFound(); |
||||
} |
} |
||||
catch (KeyNotFoundException) { |
catch (InvalidOperationException) |
||||
return NotFound(); |
{ |
||||
} |
return BadRequest(); |
||||
catch (InvalidOperationException) |
} |
||||
{ |
|
||||
return BadRequest(); |
} |
||||
} |
|
||||
|
[HttpPost] |
||||
} |
public async Task<ActionResult<Case>> NewCase(CaseCreate data) |
||||
|
{ |
||||
[HttpPost] |
try |
||||
public async Task<ActionResult<Case>> NewCase(CaseCreate data) |
{ |
||||
{ |
Session s = await Handler.GetSession(data.SessionId); |
||||
Session s = await Handler.GetSession(data.SessionId); |
Case c = await mgr.CreateCase(s, data.DoctorId, data.ChildId, data.Title, data.StartDate); |
||||
if (s == null) return Unauthorized(); |
return CreatedAtAction("PostGetCase", new { id = c.Id }, c); |
||||
|
} |
||||
try |
catch (UnauthorizedAccessException) |
||||
{ |
{ |
||||
Case c = await mgr.CreateCase(s, data.DoctorId, data.ChildId, data.Title, data.StartDate); |
return Unauthorized(); |
||||
return CreatedAtAction("PostGetCase", new { id = c.Id }, c); |
} |
||||
} |
} |
||||
catch (UnauthorizedAccessException) |
|
||||
{ |
[HttpPost("{id}/updates")] |
||||
return Unauthorized(); |
public async Task<ActionResult<List<Update>>> GetUpdatesForCase(int id, string SessionId) |
||||
} |
{ |
||||
} |
try { |
||||
|
Session s = await Handler.GetSession(SessionId); |
||||
[HttpPost("{id}/updates")] |
return await mgr.GetUpdatesForCase(s, id); |
||||
public async Task<ActionResult<List<Update>>> GetUpdatesForCase(int id, string SessionId) |
} |
||||
{ |
catch (UnauthorizedAccessException) |
||||
Session s = await Handler.GetSession(SessionId); |
{ |
||||
if (s == null) return Unauthorized(); |
return Unauthorized(); |
||||
try { |
} |
||||
return await mgr.GetUpdatesForCase(s, id); |
catch (KeyNotFoundException) |
||||
} |
{ |
||||
catch (UnauthorizedAccessException) |
return NotFound(); |
||||
{ |
} |
||||
return Unauthorized(); |
} |
||||
} |
|
||||
catch (KeyNotFoundException) |
[HttpPost("updates/{id}")] |
||||
{ |
public async Task<ActionResult<Update>> GetUpdate(int id, string SessionId) |
||||
return NotFound(); |
{ |
||||
} |
try |
||||
} |
{ |
||||
|
Session s = await Handler.GetSession(SessionId); |
||||
[HttpPost("updates/{id}")] |
return await mgr.GetUpdate(s, id); |
||||
public async Task<ActionResult<Update>> GetUpdate(int id, string SessionId) |
} |
||||
{ |
catch (UnauthorizedAccessException) |
||||
Session s = await Handler.GetSession(SessionId); |
{ |
||||
if (s == null) return Unauthorized(); |
return Unauthorized(); |
||||
try |
} |
||||
{ |
catch (KeyNotFoundException) |
||||
return await mgr.GetUpdate(s, id); |
{ |
||||
} |
return NotFound(); |
||||
catch (UnauthorizedAccessException) |
} |
||||
{ |
} |
||||
return Unauthorized(); |
|
||||
} |
// POST /api/Case/{id}/close
|
||||
catch (KeyNotFoundException) |
[HttpPost("{id}/close")] |
||||
{ |
public async Task<IActionResult> PostClose(int id, string SessionId) |
||||
return NotFound(); |
{ |
||||
} |
try |
||||
} |
{ |
||||
|
Session s = await Handler.GetSession(SessionId); |
||||
// POST /api/Case/{id}/close
|
await mgr.SetCertified(s, id); |
||||
[HttpPost("{id}/close")] |
return Ok(); |
||||
public async Task<IActionResult> PostClose(int id, string SessionId) |
} |
||||
{ |
catch (UnauthorizedAccessException) |
||||
Session s = await Handler.GetSession(SessionId); |
{ |
||||
if (s == null) return Unauthorized(); |
return Unauthorized(); |
||||
try |
} |
||||
{ |
catch (KeyNotFoundException) |
||||
await mgr.SetCertified(s, id); |
{ |
||||
return Ok(); |
return NotFound(); |
||||
} |
} |
||||
catch (UnauthorizedAccessException) |
} |
||||
{ |
// POST /api/Case/{id}/close
|
||||
return Unauthorized(); |
[HttpPost("{id}/cure")] |
||||
} |
public async Task<IActionResult> PostCured(int id, string SessionId) |
||||
catch (KeyNotFoundException) |
{ |
||||
{ |
try |
||||
return NotFound(); |
{ |
||||
} |
Session s = await Handler.GetSession(SessionId); |
||||
} |
await mgr.SetCured(s, id); |
||||
// POST /api/Case/{id}/close
|
return Ok(); |
||||
[HttpPost("{id}/cure")] |
} |
||||
public async Task<IActionResult> PostCured(int id, string SessionId) |
catch (UnauthorizedAccessException) |
||||
{ |
{ |
||||
Session s = await Handler.GetSession(SessionId); |
return Unauthorized(); |
||||
if (s == null) return Unauthorized(); |
} |
||||
try |
catch (KeyNotFoundException) |
||||
{ |
{ |
||||
await mgr.SetCured(s, id); |
return NotFound(); |
||||
return Ok(); |
} |
||||
} |
} |
||||
catch (UnauthorizedAccessException) |
|
||||
{ |
// POST /api/Case/filter
|
||||
return Unauthorized(); |
[HttpPost("filter")] |
||||
} |
public async Task<ActionResult<List<Case>>> Filter(CaseFilter filters) |
||||
catch (KeyNotFoundException) |
{ |
||||
{ |
try |
||||
return NotFound(); |
{ |
||||
} |
Session s = await Handler.GetSession(filters.SessionId); |
||||
} |
return await mgr.FilterCases(s, filters); |
||||
|
} |
||||
// POST /api/Case/filter
|
catch (UnauthorizedAccessException) |
||||
[HttpPost("filter")] |
{ |
||||
public async Task<ActionResult<List<Case>>> Filter(CaseFilter filters) |
return Unauthorized(); |
||||
{ |
} |
||||
Session s = await Handler.GetSession(filters.SessionId); |
|
||||
if (s == null) return Unauthorized(); |
} |
||||
|
|
||||
try |
} |
||||
{ |
} |
||||
return await mgr.FilterCases(s, filters); |
|
||||
} |
|
||||
catch (UnauthorizedAccessException) |
|
||||
{ |
|
||||
return Unauthorized(); |
|
||||
} |
|
||||
|
|
||||
} |
|
||||
|
|
||||
} |
|
||||
} |
|
||||
|
@ -1,95 +1,96 @@ |
|||||
using System; |
using System; |
||||
using System.Collections.Generic; |
using System.Collections.Generic; |
||||
using System.Linq; |
using System.Linq; |
||||
using System.Threading.Tasks; |
using System.Threading.Tasks; |
||||
using Microsoft.AspNetCore.Http; |
using Microsoft.AspNetCore.Http; |
||||
using Microsoft.AspNetCore.Mvc; |
using Microsoft.AspNetCore.Mvc; |
||||
using CoviDok.Api.Objects; |
using CoviDok.Api.Objects; |
||||
using CoviDok.Api.Request; |
using CoviDok.Api.Request; |
||||
using CoviDok.BLL.User.Managers; |
using CoviDok.BLL.User.Managers; |
||||
using CoviDok.BLL.Sessions; |
using CoviDok.BLL.Sessions; |
||||
using CoviDok.Data.SessionProviders; |
using CoviDok.Data.SessionProviders; |
||||
using CoviDok.Data.MySQL; |
using CoviDok.Data.MySQL; |
||||
|
|
||||
namespace CoviDok.Controllers |
namespace CoviDok.Controllers |
||||
{ |
{ |
||||
[Route("api/[controller]")]
|
[Route("api/[controller]")]
|
||||
[ApiController] |
[ApiController] |
||||
public class ChildController : ControllerBase |
public class ChildController : ControllerBase |
||||
{ |
{ |
||||
private readonly SessionHandler Handler = new SessionHandler(); |
private readonly SessionHandler Handler = new SessionHandler(); |
||||
|
|
||||
private readonly ChildManager ChildManager = new ChildManager(); |
private readonly ChildManager ChildManager = new ChildManager(); |
||||
|
|
||||
// POST: api/Child/5
|
// POST: api/Child/5
|
||||
[HttpPost("{id}")] |
[HttpPost("{id}")] |
||||
public async Task<ActionResult<PublicChild>> GetPublicChild(int id, string SessionId) |
public async Task<ActionResult<PublicChild>> GetPublicChild(int id, string SessionId) |
||||
{ |
{ |
||||
Session s = await Handler.GetSession(SessionId); |
try |
||||
if (s == null) return Unauthorized(); |
{ |
||||
|
Session s = await Handler.GetSession(SessionId); |
||||
try |
return await ChildManager.GetChild(s, id); |
||||
{ |
} |
||||
return await ChildManager.GetChild(s, id); |
catch (UnauthorizedAccessException) |
||||
} |
{ |
||||
catch (UnauthorizedAccessException) |
return Unauthorized(); |
||||
{ |
} |
||||
return Unauthorized(); |
catch (KeyNotFoundException) |
||||
} |
{ |
||||
catch (KeyNotFoundException) |
return NotFound(); |
||||
{ |
} |
||||
return NotFound(); |
} |
||||
} |
|
||||
} |
// PUT: api/Child/5
|
||||
|
// To protect from overposting attacks, enable the specific properties you want to bind to, for
|
||||
// PUT: api/Child/5
|
// more details, see https://go.microsoft.com/fwlink/?linkid=2123754.
|
||||
// To protect from overposting attacks, enable the specific properties you want to bind to, for
|
[HttpPut("{id}")] |
||||
// more details, see https://go.microsoft.com/fwlink/?linkid=2123754.
|
public async Task<IActionResult> PutPublicChild(int id, PublicChild publicChild) |
||||
[HttpPut("{id}")] |
{ |
||||
public async Task<IActionResult> PutPublicChild(int id, PublicChild publicChild) |
try { |
||||
{ |
Session s = await Handler.GetSession(publicChild.SessionId); |
||||
Session s = await Handler.GetSession(publicChild.SessionId); |
await ChildManager.UpdateChild(s, id, publicChild); |
||||
if (s == null) return Unauthorized(); |
return NoContent(); |
||||
try { |
} |
||||
await ChildManager.UpdateChild(s, id, publicChild); |
catch (UnauthorizedAccessException) { |
||||
return NoContent(); |
return Unauthorized(); |
||||
} |
} |
||||
catch (UnauthorizedAccessException) { |
catch (KeyNotFoundException) { |
||||
return Unauthorized(); |
return NotFound(); |
||||
} |
} |
||||
catch (KeyNotFoundException) { |
catch (FormatException) |
||||
return NotFound(); |
{ |
||||
} |
return BadRequest(); |
||||
catch (FormatException) |
} |
||||
{ |
} |
||||
return BadRequest(); |
|
||||
} |
[HttpPost("parent")] |
||||
} |
public async Task<ActionResult<List<PublicChild>>> GetChildrenOfParent(string SessionId) |
||||
|
{ |
||||
[HttpPost("parent")] |
try { |
||||
public async Task<ActionResult<List<PublicChild>>> GetChildrenOfParent(string SessionId) |
Session s = await Handler.GetSession(SessionId); |
||||
{ |
return ChildManager.ChildrenOfParent(s.Id); |
||||
Session s = await Handler.GetSession(SessionId); |
} |
||||
if (s == null) return Unauthorized(); |
catch (UnauthorizedAccessException) |
||||
return ChildManager.ChildrenOfParent(s.Id); |
{ |
||||
} |
return Unauthorized(); |
||||
|
} |
||||
// POST: api/Child
|
|
||||
// To protect from overposting attacks, enable the specific properties you want to bind to, for
|
} |
||||
// more details, see https://go.microsoft.com/fwlink/?linkid=2123754.
|
|
||||
[HttpPost] |
// POST: api/Child
|
||||
public async Task<ActionResult<PublicChild>> PostPublicChild(PublicChild publicChild) |
// To protect from overposting attacks, enable the specific properties you want to bind to, for
|
||||
{ |
// more details, see https://go.microsoft.com/fwlink/?linkid=2123754.
|
||||
Session s = await Handler.GetSession(publicChild.SessionId); |
[HttpPost] |
||||
if (s == null ) return Unauthorized(); |
public async Task<ActionResult<PublicChild>> PostPublicChild(PublicChild publicChild) |
||||
|
{ |
||||
try { |
try { |
||||
int Id = await ChildManager.AddChild(s, publicChild); |
Session s = await Handler.GetSession(publicChild.SessionId); |
||||
return CreatedAtAction("GetPublicChild", new { id = Id }, publicChild); |
int Id = await ChildManager.AddChild(s, publicChild); |
||||
} |
return CreatedAtAction("GetPublicChild", new { id = Id }, publicChild); |
||||
catch (UnauthorizedAccessException) { |
} |
||||
return Unauthorized(); |
catch (UnauthorizedAccessException) { |
||||
} |
return Unauthorized(); |
||||
} |
} |
||||
} |
} |
||||
} |
} |
||||
|
} |
||||
|
@ -1,117 +1,116 @@ |
|||||
using System; |
using System; |
||||
using System.Collections.Generic; |
using System.Collections.Generic; |
||||
using System.Linq; |
using System.Linq; |
||||
using System.Threading.Tasks; |
using System.Threading.Tasks; |
||||
using CoviDok.Api; |
using CoviDok.Api; |
||||
using CoviDok.Api.Objects; |
using CoviDok.Api.Objects; |
||||
using CoviDok.Api.Request; |
using CoviDok.Api.Request; |
||||
using CoviDok.BLL.Sessions; |
using CoviDok.BLL.Sessions; |
||||
using CoviDok.BLL.User.Managers; |
using CoviDok.BLL.User.Managers; |
||||
using CoviDok.Data.MySQL; |
using CoviDok.Data.MySQL; |
||||
using CoviDok.Data.SessionProviders; |
using CoviDok.Data.SessionProviders; |
||||
using Microsoft.AspNetCore.Http; |
using Microsoft.AspNetCore.Http; |
||||
using Microsoft.AspNetCore.Mvc; |
using Microsoft.AspNetCore.Mvc; |
||||
|
|
||||
namespace CoviDok.Controllers |
namespace CoviDok.Controllers |
||||
{ |
{ |
||||
[Route("api/[controller]")]
|
[Route("api/[controller]")]
|
||||
[ApiController] |
[ApiController] |
||||
public class DoctorController : ControllerBase |
public class DoctorController : ControllerBase |
||||
{ |
{ |
||||
private readonly SessionHandler Handler = new SessionHandler(); |
private readonly SessionHandler Handler = new SessionHandler(); |
||||
|
|
||||
private readonly DoctorManager doctorHandler = new DoctorManager(); |
private readonly DoctorManager doctorHandler = new DoctorManager(); |
||||
// GET /api/Doc
|
// GET /api/Doc
|
||||
[HttpGet] |
[HttpGet] |
||||
public async Task<ActionResult<ICollection<PublicDoctor>>> GetDoctors() |
public async Task<ActionResult<ICollection<PublicDoctor>>> GetDoctors() |
||||
{ |
{ |
||||
return await doctorHandler.GetDoctors(); |
return await doctorHandler.GetDoctors(); |
||||
} |
} |
||||
|
|
||||
[HttpGet("byFirstName/{firstName}")] |
[HttpGet("byFirstName/{firstName}")] |
||||
public async Task<ActionResult<ICollection<PublicDoctor>>> GetDoctorsByFirstName(string firstName) |
public async Task<ActionResult<ICollection<PublicDoctor>>> GetDoctorsByFirstName(string firstName) |
||||
{ |
{ |
||||
return await doctorHandler.GetDoctors(firstName: firstName); |
return await doctorHandler.GetDoctors(firstName: firstName); |
||||
} |
} |
||||
[HttpGet("byLastName/{lastName}")] |
[HttpGet("byLastName/{lastName}")] |
||||
public async Task<ActionResult<ICollection<PublicDoctor>>> GetDoctorsByLastName(string lastName) |
public async Task<ActionResult<ICollection<PublicDoctor>>> GetDoctorsByLastName(string lastName) |
||||
{ |
{ |
||||
return await doctorHandler.GetDoctors(lastName: lastName); |
return await doctorHandler.GetDoctors(lastName: lastName); |
||||
} |
} |
||||
|
|
||||
[HttpGet("byName/{firstName}/{lastName}")] |
[HttpGet("byName/{firstName}/{lastName}")] |
||||
public async Task<ActionResult<ICollection<PublicDoctor>>> GetDoctorByName(string firstName, string lastName) |
public async Task<ActionResult<ICollection<PublicDoctor>>> GetDoctorByName(string firstName, string lastName) |
||||
{ |
{ |
||||
return await doctorHandler.GetDoctors(lastName: lastName, firstName: firstName); |
return await doctorHandler.GetDoctors(lastName: lastName, firstName: firstName); |
||||
} |
} |
||||
|
|
||||
[HttpGet("{id}")] |
[HttpGet("{id}")] |
||||
public async Task<ActionResult<PublicDoctor>> GetDoctor(int id) |
public async Task<ActionResult<PublicDoctor>> GetDoctor(int id) |
||||
{ |
{ |
||||
try |
try |
||||
{ |
{ |
||||
return await doctorHandler.GetDoctor(id); |
return await doctorHandler.GetDoctor(id); |
||||
} |
} |
||||
catch (KeyNotFoundException) |
catch (KeyNotFoundException) |
||||
{ |
{ |
||||
return NotFound(); |
return NotFound(); |
||||
} |
} |
||||
} |
} |
||||
|
|
||||
[HttpPut("{id}")] |
[HttpPut("{id}")] |
||||
public async Task<IActionResult> PutDoctor(int id, PublicDoctor doctor) { |
public async Task<IActionResult> PutDoctor(int id, PublicDoctor doctor) { |
||||
Session s = await Handler.GetSession(doctor.SessionId); |
Session s = await Handler.GetSession(doctor.SessionId); |
||||
if (s == null) return Unauthorized(); |
if (s == null) return Unauthorized(); |
||||
try |
try |
||||
{ |
{ |
||||
await doctorHandler.UpdateDoctor(s, id, doctor); |
await doctorHandler.UpdateDoctor(s, id, doctor); |
||||
return NoContent(); |
return NoContent(); |
||||
} |
} |
||||
catch (UnauthorizedAccessException) |
catch (UnauthorizedAccessException) |
||||
{ |
{ |
||||
return Unauthorized(); |
return Unauthorized(); |
||||
} |
} |
||||
catch (KeyNotFoundException) |
catch (KeyNotFoundException) |
||||
{ |
{ |
||||
return NotFound(); |
return NotFound(); |
||||
} |
} |
||||
catch (FormatException) |
catch (FormatException) |
||||
{ |
{ |
||||
return BadRequest(); |
return BadRequest(); |
||||
} |
} |
||||
} |
} |
||||
|
|
||||
// GET /api/Doc/{id}/assistants
|
// GET /api/Doc/{id}/assistants
|
||||
[HttpGet("{id}/assistants")] |
[HttpGet("{id}/assistants")] |
||||
public async Task<ActionResult<ICollection<PublicAssistant>>> GetAssistantsOfDoctor(int id) |
public async Task<ActionResult<ICollection<PublicAssistant>>> GetAssistantsOfDoctor(int id) |
||||
{ |
{ |
||||
try |
try |
||||
{ |
{ |
||||
return await doctorHandler.GetAssistants(id); |
return await doctorHandler.GetAssistants(id); |
||||
} |
} |
||||
catch (KeyNotFoundException) |
catch (KeyNotFoundException) |
||||
{ |
{ |
||||
return NotFound(); |
return NotFound(); |
||||
} |
} |
||||
} |
} |
||||
|
|
||||
// GET /api/Doc/{id}/children
|
// GET /api/Doc/{id}/children
|
||||
[HttpPost("{id}/children")] |
[HttpPost("{id}/children")] |
||||
public async Task<ActionResult<ICollection<PublicChild>>> GetChildrenOfDoctor(int id, string SessionId) |
public async Task<ActionResult<ICollection<PublicChild>>> GetChildrenOfDoctor(int id, string SessionId) |
||||
{ |
{ |
||||
Session s = await Handler.GetSession(SessionId); |
try |
||||
if (s == null) return Unauthorized(); |
{ |
||||
try |
Session s = await Handler.GetSession(SessionId); |
||||
{ |
return await doctorHandler.GetChildren(id); |
||||
return await doctorHandler.GetChildren(id); |
} |
||||
} |
catch (KeyNotFoundException) |
||||
catch (KeyNotFoundException) |
{ |
||||
{ |
return NotFound(); |
||||
return NotFound(); |
} |
||||
} |
catch (UnauthorizedAccessException) { |
||||
catch (UnauthorizedAccessException) { |
return Unauthorized(); |
||||
return Unauthorized(); |
} |
||||
} |
} |
||||
} |
} |
||||
} |
} |
||||
} |
|
||||
|
@ -1,88 +1,91 @@ |
|||||
using System; |
using System; |
||||
using System.Collections.Generic; |
using System.Collections.Generic; |
||||
using System.IO; |
using System.IO; |
||||
using System.Linq; |
using System.Linq; |
||||
using System.Security.Cryptography.X509Certificates; |
using System.Security.Cryptography.X509Certificates; |
||||
using System.Threading.Tasks; |
using System.Threading.Tasks; |
||||
using CoviDok.Api; |
using CoviDok.Api; |
||||
using CoviDok.Api.Request; |
using CoviDok.Api.Request; |
||||
using CoviDok.BLL; |
using CoviDok.BLL; |
||||
using CoviDok.BLL.Sessions; |
using CoviDok.BLL.Sessions; |
||||
using CoviDok.BLL.Storage; |
using CoviDok.BLL.Storage; |
||||
using CoviDok.Data.SessionProviders; |
using CoviDok.Data.SessionProviders; |
||||
using CoviDok.Data.StorageProviders; |
using CoviDok.Data.StorageProviders; |
||||
using Microsoft.AspNetCore.Http; |
using Microsoft.AspNetCore.Http; |
||||
using Microsoft.AspNetCore.Mvc; |
using Microsoft.AspNetCore.Mvc; |
||||
using Microsoft.Extensions.Configuration; |
using Microsoft.Extensions.Configuration; |
||||
using Microsoft.Extensions.Options; |
using Microsoft.Extensions.Options; |
||||
using NCuid; |
using NCuid; |
||||
|
|
||||
namespace CoviDok.Controllers |
namespace CoviDok.Controllers |
||||
{ |
{ |
||||
[Route("api/[controller]")]
|
[Route("api/[controller]")]
|
||||
[ApiController] |
[ApiController] |
||||
public class ImagesController : ControllerBase |
public class ImagesController : ControllerBase |
||||
{ |
{ |
||||
private StorageHandler MinioHandler = new StorageHandler(new MinioProvider()); |
private StorageHandler MinioHandler = new StorageHandler(new MinioProvider()); |
||||
private readonly string BucketName = "test1"; |
private readonly string BucketName = "test1"; |
||||
|
|
||||
private readonly SessionHandler Handler = new SessionHandler(); |
private readonly SessionHandler Handler = new SessionHandler(); |
||||
|
|
||||
private static Stream MakeStream(string s) |
private static Stream MakeStream(string s) |
||||
{ |
{ |
||||
var stream = new MemoryStream(); |
var stream = new MemoryStream(); |
||||
var writer = new StreamWriter(stream); |
var writer = new StreamWriter(stream); |
||||
writer.Write(s); |
writer.Write(s); |
||||
writer.Flush(); |
writer.Flush(); |
||||
stream.Position = 0; |
stream.Position = 0; |
||||
return stream; |
return stream; |
||||
} |
} |
||||
|
|
||||
[HttpPost("Upload")] |
[HttpPost("Upload")] |
||||
public async Task<ActionResult<GenericResponse>> OnPostImage(ImagePost post) |
public async Task<ActionResult<GenericResponse>> OnPostImage(ImagePost post) |
||||
{ |
{ |
||||
GenericResponse response = new GenericResponse(); |
GenericResponse response = new GenericResponse(); |
||||
Session s = await Handler.GetSession(post.SessionId); |
Session s = await Handler.GetSession(post.SessionId); |
||||
if (s == null) |
if (s == null) |
||||
{ |
{ |
||||
response.Status = Status.Error; |
response.Status = Status.Error; |
||||
response.Body["reason"] = "unauthorized"; |
response.Body["reason"] = "unauthorized"; |
||||
return response; |
return response; |
||||
} |
} |
||||
StorageResult Result = await MinioHandler.UploadImage(BucketName, MakeStream(post.File), post.File.Length, Cuid.Generate()); |
StorageResult Result = await MinioHandler.UploadImage(BucketName, MakeStream(post.File), post.File.Length, Cuid.Generate()); |
||||
if (!Result.Success) response.Status = Status.Error; |
if (!Result.Success) response.Status = Status.Error; |
||||
response.Body["reason"] = Result.Data; |
response.Body["reason"] = Result.Data; |
||||
|
|
||||
return response; |
return response; |
||||
} |
} |
||||
|
|
||||
[HttpPost("Download")] |
[HttpPost("Download")] |
||||
public async Task<GenericResponse> OnGetImage(ImageGet imageGet) |
public async Task<GenericResponse> OnGetImage(ImageGet imageGet) |
||||
{ |
{ |
||||
GenericResponse response = new GenericResponse(); |
GenericResponse response = new GenericResponse(); |
||||
Session s = await Handler.GetSession(imageGet.SessionId); |
try |
||||
if (s == null) |
{ |
||||
{ |
Session s = await Handler.GetSession(imageGet.SessionId); |
||||
response.Status = Status.Error; |
string res = null; |
||||
response.Body["reason"] = "unauthorized"; |
await MinioHandler.GetImage(BucketName, imageGet.ImageId, (stream) => |
||||
return response; |
{ |
||||
} |
StreamReader reader = new StreamReader(stream); |
||||
try { |
res = reader.ReadToEnd(); |
||||
string res = null; |
}); |
||||
await MinioHandler.GetImage(BucketName, imageGet.ImageId, (stream) => { |
|
||||
StreamReader reader = new StreamReader(stream); |
response.Body["image"] = res; |
||||
res = reader.ReadToEnd(); |
return response; |
||||
}); |
} |
||||
|
catch (UnauthorizedAccessException) |
||||
response.Body["image"] = res; |
{ |
||||
return response; |
response.Status = Status.Error; |
||||
} |
response.Body["reason"] = "unauthorized"; |
||||
catch (KeyNotFoundException) |
return response; |
||||
{ |
} |
||||
response.Status = Status.Error; |
|
||||
response.Body["reason"] = "Image not found!"; |
catch (KeyNotFoundException) |
||||
return response; |
{ |
||||
} |
response.Status = Status.Error; |
||||
} |
response.Body["reason"] = "Image not found!"; |
||||
} |
return response; |
||||
} |
} |
||||
|
} |
||||
|
} |
||||
|
} |
||||
|
@ -1,79 +1,80 @@ |
|||||
using System; |
using System; |
||||
using System.Collections.Generic; |
using System.Collections.Generic; |
||||
using System.Linq; |
using System.Linq; |
||||
using System.Threading.Tasks; |
using System.Threading.Tasks; |
||||
using CoviDok.Api.Objects; |
using CoviDok.Api.Objects; |
||||
using CoviDok.Api.Request; |
using CoviDok.Api.Request; |
||||
using CoviDok.BLL.Sessions; |
using CoviDok.BLL.Sessions; |
||||
using CoviDok.BLL.User.Managers; |
using CoviDok.BLL.User.Managers; |
||||
using CoviDok.Data.MySQL; |
using CoviDok.Data.MySQL; |
||||
using CoviDok.Data.SessionProviders; |
using CoviDok.Data.SessionProviders; |
||||
using Microsoft.AspNetCore.Http; |
using Microsoft.AspNetCore.Http; |
||||
using Microsoft.AspNetCore.Mvc; |
using Microsoft.AspNetCore.Mvc; |
||||
|
|
||||
namespace CoviDok.Controllers |
namespace CoviDok.Controllers |
||||
{ |
{ |
||||
[Route("api/[controller]")]
|
[Route("api/[controller]")]
|
||||
[ApiController] |
[ApiController] |
||||
public class ParentController : ControllerBase |
public class ParentController : ControllerBase |
||||
{ |
{ |
||||
private readonly SessionHandler sessionHandler = new SessionHandler(); |
private readonly SessionHandler sessionHandler = new SessionHandler(); |
||||
|
|
||||
private readonly ParentManager parentManager = new ParentManager(); |
private readonly ParentManager parentManager = new ParentManager(); |
||||
|
|
||||
[HttpPost("{id}")] |
[HttpPost("{id}")] |
||||
public async Task<ActionResult<PublicParent>> GetParent(int id, string SessionId) |
public async Task<ActionResult<PublicParent>> GetParent(int id, string SessionId) |
||||
{ |
{ |
||||
Session s = await sessionHandler.GetSession(SessionId); |
try { |
||||
if (s == null) return Unauthorized(); |
Session s = await sessionHandler.GetSession(SessionId); |
||||
try { |
return await parentManager.GetParent(id); |
||||
return await parentManager.GetParent(id); |
} |
||||
} |
catch (UnauthorizedAccessException) |
||||
catch (KeyNotFoundException) |
{ |
||||
{ |
return Unauthorized(); |
||||
return NotFound(); |
} |
||||
} |
catch (KeyNotFoundException) |
||||
} |
{ |
||||
|
return NotFound(); |
||||
[HttpPut("{id}")] |
} |
||||
public async Task<IActionResult> PutParent(int id, PublicParent parent) |
} |
||||
{ |
|
||||
Session s = await sessionHandler.GetSession(parent.SessionId); |
[HttpPut("{id}")] |
||||
if (s == null) return Unauthorized(); |
public async Task<IActionResult> PutParent(int id, PublicParent parent) |
||||
try { |
{ |
||||
await parentManager.UpdateParent(s, id, parent); |
try { |
||||
return NoContent(); |
Session s = await sessionHandler.GetSession(parent.SessionId); |
||||
} |
await parentManager.UpdateParent(s, id, parent); |
||||
catch (UnauthorizedAccessException) |
return NoContent(); |
||||
{ |
} |
||||
return Unauthorized(); |
catch (UnauthorizedAccessException) |
||||
} |
{ |
||||
catch (KeyNotFoundException) |
return Unauthorized(); |
||||
{ |
} |
||||
return NotFound(); |
catch (KeyNotFoundException) |
||||
} |
{ |
||||
catch (FormatException) |
return NotFound(); |
||||
{ |
} |
||||
return BadRequest(); |
catch (FormatException) |
||||
} |
{ |
||||
} |
return BadRequest(); |
||||
[HttpPost("{id}/children")] |
} |
||||
public async Task<ActionResult<List<PublicChild>>> GetChildrenOfParent(int id, string SessionId) |
} |
||||
{ |
[HttpPost("{id}/children")] |
||||
Session s = await sessionHandler.GetSession(SessionId); |
public async Task<ActionResult<List<PublicChild>>> GetChildrenOfParent(int id, string SessionId) |
||||
if (s == null) return Unauthorized(); |
{ |
||||
try |
try |
||||
{ |
{ |
||||
return await parentManager.GetChildren(id); |
Session s = await sessionHandler.GetSession(SessionId); |
||||
} |
return await parentManager.GetChildren(id); |
||||
catch (KeyNotFoundException) |
} |
||||
{ |
catch (KeyNotFoundException) |
||||
return NotFound(); |
{ |
||||
} |
return NotFound(); |
||||
catch (UnauthorizedAccessException) |
} |
||||
{ |
catch (UnauthorizedAccessException) |
||||
return Unauthorized(); |
{ |
||||
} |
return Unauthorized(); |
||||
} |
} |
||||
} |
} |
||||
} |
} |
||||
|
} |
||||
|
@ -1,41 +1,41 @@ |
|||||
# Default values for covidok. |
# Default values for covidok. |
||||
# This is a YAML-formatted file. |
# This is a YAML-formatted file. |
||||
# Declare variables to be passed into your templates. |
# Declare variables to be passed into your templates. |
||||
|
|
||||
images: |
images: |
||||
covidok: |
covidok: |
||||
name: docker.local/bme/covidok |
name: docker.local/bme/covidok |
||||
tag: latest |
tag: latest |
||||
mysql: |
mysql: |
||||
name: mysql |
name: mysql |
||||
tag: 8.0 |
tag: 8.0 |
||||
minio: |
minio: |
||||
name: minio/minio |
name: minio/minio |
||||
tag: latest |
tag: latest |
||||
redis: |
redis: |
||||
name: redis |
name: redis |
||||
tag: 6 |
tag: 6 |
||||
pullPolicy: Always |
pullPolicy: Always |
||||
# Overrides the image tag whose default is the chart appVersion. |
# Overrides the image tag whose default is the chart appVersion. |
||||
|
|
||||
config: |
config: |
||||
minio: |
minio: |
||||
accesskey: "accesskey" |
accesskey: "accesskey" |
||||
secretkey: "secretkey" |
secretkey: "secretkey" |
||||
mysql: |
mysql: |
||||
database: "covidok" |
database: "covidok" |
||||
user: "covdiok" |
user: "covidok" |
||||
password: "covidok" |
password: "covidok" |
||||
redis: |
redis: |
||||
port: "6379" |
port: "6379" |
||||
|
|
||||
|
|
||||
imagePullSecrets: [] |
imagePullSecrets: [] |
||||
nameOverride: "" |
nameOverride: "" |
||||
fullnameOverride: "" |
fullnameOverride: "" |
||||
|
|
||||
podAnnotations: {} |
podAnnotations: {} |
||||
|
|
||||
service: |
service: |
||||
type: ClusterIP |
type: ClusterIP |
||||
port: 80 |
port: 80 |
||||
|
Loading…
Reference in new issue