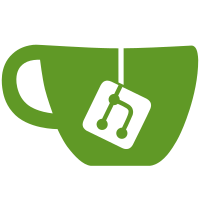
6 changed files with 210 additions and 0 deletions
@ -0,0 +1,67 @@ |
|||
using Minio; |
|||
using Minio.Exceptions; |
|||
using System; |
|||
using System.Collections.Generic; |
|||
using System.Linq; |
|||
using System.Threading.Tasks; |
|||
|
|||
namespace CoviDok.BLL |
|||
{ |
|||
class MinioHandler |
|||
{ |
|||
private MinioClient Client = null; |
|||
|
|||
public class MinioResult |
|||
{ |
|||
bool Success; |
|||
string Data; |
|||
|
|||
public MinioResult(bool Success, string Data) |
|||
{ |
|||
this.Success = Success; |
|||
this.Data = Data; |
|||
} |
|||
} |
|||
|
|||
public MinioHandler() |
|||
{ |
|||
Client = new MinioClient( |
|||
"192.168.0.160:9000", |
|||
"secretaccesskey", |
|||
"secretsecretkey"); |
|||
} |
|||
|
|||
public async Task<MinioResult> Upload(string BucketName, string FilePath, string ObjectName) |
|||
{ |
|||
try |
|||
{ |
|||
// Make a bucket on the server, if not already present.
|
|||
bool found = await Client.BucketExistsAsync(BucketName); |
|||
if (!found) |
|||
{ |
|||
await Client.MakeBucketAsync(BucketName); |
|||
} |
|||
// Upload a file to bucket.
|
|||
await Client.PutObjectAsync(BucketName, ObjectName, FilePath); |
|||
return new MinioResult(true, BucketName + ":" + ObjectName); |
|||
} |
|||
catch (MinioException e) |
|||
{ |
|||
return new MinioResult(false, e.Message); |
|||
} |
|||
} |
|||
|
|||
public async Task<MinioResult> GetImage(string BucketName, string FilePath, string ObjectName) |
|||
{ |
|||
try |
|||
{ |
|||
await Client.GetObjectAsync(BucketName, ObjectName, FilePath); |
|||
return new MinioResult(true, FilePath); |
|||
} |
|||
catch (MinioException e) |
|||
{ |
|||
return new MinioResult(false, e.Message); |
|||
} |
|||
} |
|||
} |
|||
} |
@ -0,0 +1,38 @@ |
|||
using System; |
|||
using System.Collections.Generic; |
|||
using System.Text; |
|||
|
|||
namespace CoviDok.BLL |
|||
{ |
|||
class DummyProvider : ISessionProvider |
|||
{ |
|||
private readonly Dictionary<string, string> dict; |
|||
public DummyProvider() |
|||
{ |
|||
dict = new Dictionary<string, string>(); |
|||
} |
|||
|
|||
public void Del(string key) |
|||
{ |
|||
dict.Remove(key); |
|||
} |
|||
|
|||
public string Get(string key) |
|||
{ |
|||
try |
|||
{ |
|||
return dict[key]; |
|||
} |
|||
catch (KeyNotFoundException) |
|||
{ |
|||
return null; |
|||
} |
|||
|
|||
} |
|||
|
|||
public void Set(string key, string value) |
|||
{ |
|||
dict[key] = value; |
|||
} |
|||
} |
|||
} |
@ -0,0 +1,16 @@ |
|||
using System; |
|||
using System.Collections.Generic; |
|||
using System.Text; |
|||
|
|||
namespace CoviDok.BLL |
|||
{ |
|||
interface ISessionProvider |
|||
{ |
|||
public void Set(string key, string value); |
|||
|
|||
// Returns null if not found
|
|||
public string Get(string key); |
|||
|
|||
public void Del(string key); |
|||
} |
|||
} |
@ -0,0 +1,30 @@ |
|||
using StackExchange.Redis; |
|||
using System; |
|||
using System.Collections.Generic; |
|||
using System.Text; |
|||
|
|||
namespace CoviDok.BLL |
|||
{ |
|||
class RedisProvider : ISessionProvider |
|||
{ |
|||
private readonly IDatabase conn; |
|||
public RedisProvider(string host) |
|||
{ |
|||
conn = ConnectionMultiplexer.Connect(host).GetDatabase(); |
|||
} |
|||
public void Del(string key) |
|||
{ |
|||
conn.KeyDelete(key); |
|||
} |
|||
|
|||
public string Get(string key) |
|||
{ |
|||
return conn.StringGet(key); |
|||
} |
|||
|
|||
public void Set(string key, string value) |
|||
{ |
|||
conn.StringSet(key, value); |
|||
} |
|||
} |
|||
} |
@ -0,0 +1,13 @@ |
|||
using System; |
|||
using System.Collections.Generic; |
|||
using System.Text; |
|||
|
|||
namespace CoviDok.BLL |
|||
{ |
|||
public class Session |
|||
{ |
|||
public string ID { get; set; } |
|||
public string Type { get; set; } |
|||
public DateTime LastAccess { get; set; } |
|||
} |
|||
} |
@ -0,0 +1,46 @@ |
|||
using System; |
|||
using System.Collections.Generic; |
|||
using System.Text; |
|||
using System.Text.Json; |
|||
using NCuid; |
|||
|
|||
namespace CoviDok.BLL |
|||
{ |
|||
class SessionHandler |
|||
{ |
|||
private readonly ISessionProvider SessionStore; |
|||
|
|||
public SessionHandler(ISessionProvider Provider) |
|||
{ |
|||
SessionStore = Provider; |
|||
} |
|||
|
|||
public Session GetSession(string SessionID) |
|||
{ |
|||
string Candidate = SessionStore.Get(SessionID); |
|||
if (Candidate == null) return null; |
|||
Session session = JsonSerializer.Deserialize<Session>(Candidate); |
|||
session.LastAccess = DateTime.Now; |
|||
SessionStore.Set(SessionID, JsonSerializer.Serialize(session)); |
|||
return session; |
|||
} |
|||
|
|||
public string CreateSession(string UserType, string UserID) |
|||
{ |
|||
Session session = new Session |
|||
{ |
|||
ID = UserID, |
|||
Type = UserType, |
|||
LastAccess = DateTime.Now |
|||
}; |
|||
string ID = Cuid.Generate(); |
|||
SessionStore.Set(ID, JsonSerializer.Serialize(session)); |
|||
return ID; |
|||
} |
|||
|
|||
public void DeleteSession(string SessionID) |
|||
{ |
|||
SessionStore.Del(SessionID); |
|||
} |
|||
} |
|||
} |
Loading…
Reference in new issue