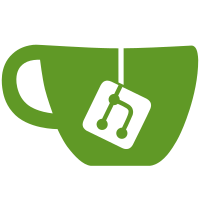
5 changed files with 106 additions and 29 deletions
@ -0,0 +1,13 @@ |
|||
using System; |
|||
using System.Collections.Generic; |
|||
using System.Linq; |
|||
using System.Threading.Tasks; |
|||
|
|||
namespace CoviDok.Api.Request |
|||
{ |
|||
public class ImageGet |
|||
{ |
|||
public string SessionID { get; set; } |
|||
public string ImageID { get; set; } |
|||
} |
|||
} |
@ -0,0 +1,14 @@ |
|||
using Microsoft.AspNetCore.Http; |
|||
using System; |
|||
using System.Collections.Generic; |
|||
using System.Linq; |
|||
using System.Threading.Tasks; |
|||
|
|||
namespace CoviDok.Api.Request |
|||
{ |
|||
public class ImagePost |
|||
{ |
|||
public string SessionID { get; set; } |
|||
public IFormFile File { get; set; } |
|||
} |
|||
} |
@ -0,0 +1,14 @@ |
|||
namespace CoviDok.BLL |
|||
{ |
|||
public class MinioResult |
|||
{ |
|||
public readonly bool Success; |
|||
public readonly string Data; |
|||
|
|||
public MinioResult(bool Success, string Data) |
|||
{ |
|||
this.Success = Success; |
|||
this.Data = Data; |
|||
} |
|||
} |
|||
} |
@ -0,0 +1,53 @@ |
|||
using System; |
|||
using System.Collections.Generic; |
|||
using System.Linq; |
|||
using System.Threading.Tasks; |
|||
using CoviDok.Api; |
|||
using CoviDok.Api.Request; |
|||
using CoviDok.BLL; |
|||
using Microsoft.AspNetCore.Http; |
|||
using Microsoft.AspNetCore.Mvc; |
|||
|
|||
namespace CoviDok.Controllers |
|||
{ |
|||
[Route("api/[controller]")]
|
|||
[ApiController] |
|||
public class ImagesController : ControllerBase |
|||
{ |
|||
private MinioHandler MinioHandler = new MinioHandler( |
|||
"192.168.0.160:9000", |
|||
"secretaccesskey", |
|||
"secretsecretkey"); |
|||
private readonly string BucketName = "test1"; |
|||
|
|||
[HttpPost] |
|||
public async Task<GenericResponse> OnPostImage(ImagePost post) |
|||
{ |
|||
GenericResponse response = new GenericResponse(); |
|||
if (post.SessionID != "a") |
|||
{ |
|||
response.Status = Status.Error; |
|||
response.Body["reason"] = "unauthorized"; |
|||
return response; |
|||
} |
|||
|
|||
MinioResult Result = await MinioHandler.UploadImage(BucketName, post.File.OpenReadStream(), post.File.Length, post.File.FileName); |
|||
if (!Result.Success) response.Status = Status.Error; |
|||
response.Body["reason"] = Result.Data; |
|||
|
|||
return response; |
|||
} |
|||
|
|||
public async Task<IActionResult> OnGetImage(ImageGet imageGet) |
|||
{ |
|||
string[] attrs = imageGet.ImageID.Split(":"); |
|||
if (attrs.Length != 2) return BadRequest(); |
|||
|
|||
FileStreamResult res = null; ; |
|||
await MinioHandler.GetImage(imageGet.ImageID, (stream) => { |
|||
res = File(stream, attrs[1]); |
|||
}); |
|||
return res; |
|||
} |
|||
} |
|||
} |
Loading…
Reference in new issue