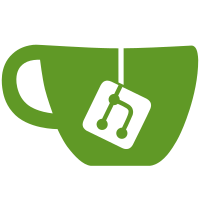
3 changed files with 134 additions and 123 deletions
@ -1,18 +1,19 @@ |
|||||
using CoviDok.Api.Objects; |
using CoviDok.Api.Objects; |
||||
using CoviDok.BLL.Sessions; |
using CoviDok.BLL.Sessions; |
||||
using CoviDok.Data.Model; |
using CoviDok.Data.Model; |
||||
using System; |
using System; |
||||
using System.Collections.Generic; |
using System.Collections.Generic; |
||||
using System.Linq; |
using System.Linq; |
||||
using System.Threading.Tasks; |
using System.Threading.Tasks; |
||||
|
|
||||
namespace CoviDok.BLL.User.Managers |
namespace CoviDok.BLL.User.Managers |
||||
{ |
{ |
||||
interface IChildHandler |
interface IChildHandler |
||||
{ |
{ |
||||
public Task<Child> GetChild(int id); |
public Task<Child> GetChild(int id); |
||||
public Task UpdateChild(int id, Child newData); |
public Task UpdateChild(int id, Child newData); |
||||
public Task<int> AddChild(Child newChild); |
public Task<int> AddChild(Child newChild); |
||||
public List<Child> GetChildren(int parentId); |
public List<Child> GetChildren(int parentId); |
||||
} |
public bool IsAuthorized(Session s, Child c); |
||||
} |
} |
||||
|
} |
||||
|
@ -1,66 +1,66 @@ |
|||||
using CoviDok.Api.Objects; |
using CoviDok.Api.Objects; |
||||
using CoviDok.BLL.Sessions; |
using CoviDok.BLL.Sessions; |
||||
using CoviDok.BLL.User.Managers; |
using CoviDok.BLL.User.Managers; |
||||
using CoviDok.Data.Model; |
using CoviDok.Data.Model; |
||||
using CoviDok.Data.MySQL; |
using CoviDok.Data.MySQL; |
||||
using Microsoft.EntityFrameworkCore; |
using Microsoft.EntityFrameworkCore; |
||||
using System; |
using System; |
||||
using System.Collections.Generic; |
using System.Collections.Generic; |
||||
using System.Linq; |
using System.Linq; |
||||
using System.Threading.Tasks; |
using System.Threading.Tasks; |
||||
|
|
||||
namespace CoviDok.Data.MySQL |
namespace CoviDok.Data.MySQL |
||||
{ |
{ |
||||
public class ChildManager |
public class ChildManager |
||||
{ |
{ |
||||
private readonly IChildHandler handler = new MySqlChildHandler(); |
private readonly IChildHandler handler = new MySqlChildHandler(); |
||||
public async Task<PublicChild> GetChild(Session s, int id) |
public async Task<PublicChild> GetChild(Session s, int id) |
||||
{ |
{ |
||||
Child child = await handler.GetChild(id); |
Child child = await handler.GetChild(id); |
||||
if (child == null) throw new KeyNotFoundException(); |
if (child == null) throw new KeyNotFoundException(); |
||||
if (child.DoctorId == s.Id || child.ParentId == s.Id) |
if (handler.IsAuthorized(s, child)) |
||||
{ |
{ |
||||
return child.ToPublic(); |
return child.ToPublic(); |
||||
} |
} |
||||
else { |
else { |
||||
throw new UnauthorizedAccessException(); |
throw new UnauthorizedAccessException(); |
||||
} |
} |
||||
} |
} |
||||
|
|
||||
public List<PublicChild> ChildrenOfParent(int parentId) |
public List<PublicChild> ChildrenOfParent(int parentId) |
||||
{ |
{ |
||||
List<PublicChild> ret = new List<PublicChild>(); |
List<PublicChild> ret = new List<PublicChild>(); |
||||
foreach (Child child in handler.GetChildren(parentId)) |
foreach (Child child in handler.GetChildren(parentId)) |
||||
{ |
{ |
||||
ret.Add(child.ToPublic()); |
ret.Add(child.ToPublic()); |
||||
} |
} |
||||
return ret; |
return ret; |
||||
} |
} |
||||
|
|
||||
public async Task UpdateChild(Session s, int id, PublicChild newData) |
public async Task UpdateChild(Session s, int id, PublicChild newData) |
||||
{ |
{ |
||||
if (id != newData.Id) throw new FormatException(); |
if (id != newData.Id) throw new FormatException(); |
||||
|
|
||||
Child child = await handler.GetChild(id); |
Child child = await handler.GetChild(id); |
||||
if (child == null) throw new KeyNotFoundException(); |
if (child == null) throw new KeyNotFoundException(); |
||||
if (child.ParentId == s.Id || child.DoctorId == s.Id) |
if (handler.IsAuthorized(s, child)) |
||||
{ |
{ |
||||
child.UpdateSelf(newData); |
child.UpdateSelf(newData); |
||||
await handler.UpdateChild(id, child); |
await handler.UpdateChild(id, child); |
||||
} |
} |
||||
else |
else |
||||
{ |
{ |
||||
throw new UnauthorizedAccessException(); |
throw new UnauthorizedAccessException(); |
||||
} |
} |
||||
} |
} |
||||
|
|
||||
public async Task<int> AddChild(Session s, PublicChild newChild) |
public async Task<int> AddChild(Session s, PublicChild newChild) |
||||
{ |
{ |
||||
if (s.Id != newChild.ParentId) throw new UnauthorizedAccessException(); |
if (s.Id != newChild.ParentId) throw new UnauthorizedAccessException(); |
||||
Child child = new Child(); |
Child child = new Child(); |
||||
child.UpdateSelf(newChild); |
child.UpdateSelf(newChild); |
||||
child.RegistrationDate = DateTime.Now; |
child.RegistrationDate = DateTime.Now; |
||||
return await handler.AddChild(child); |
return await handler.AddChild(child); |
||||
} |
} |
||||
} |
} |
||||
} |
} |
||||
|
@ -1,39 +1,49 @@ |
|||||
using CoviDok.BLL; |
using CoviDok.BLL; |
||||
using CoviDok.BLL.User.Managers; |
using CoviDok.BLL.Sessions; |
||||
using CoviDok.Data.Model; |
using CoviDok.BLL.User.Managers; |
||||
using System; |
using CoviDok.Data.Model; |
||||
using System.Collections.Generic; |
using System; |
||||
using System.Linq; |
using System.Collections.Generic; |
||||
using System.Threading.Tasks; |
using System.Linq; |
||||
|
using System.Threading.Tasks; |
||||
namespace CoviDok.Data.MySQL |
|
||||
{ |
namespace CoviDok.Data.MySQL |
||||
public class MySqlChildHandler : IChildHandler |
{ |
||||
{ |
public class MySqlChildHandler : IChildHandler |
||||
private readonly MySqlContext context = new MySqlContext(); |
{ |
||||
public async Task<int> AddChild(Child child) |
private readonly MySqlContext context = new MySqlContext(); |
||||
{ |
public async Task<int> AddChild(Child child) |
||||
context.Children.Add(child); |
{ |
||||
await context.SaveChangesAsync(); |
context.Children.Add(child); |
||||
return child.Id; |
await context.SaveChangesAsync(); |
||||
} |
return child.Id; |
||||
|
} |
||||
public async Task<Child> GetChild(int id) |
|
||||
{ |
public async Task<Child> GetChild(int id) |
||||
return await context.Children.FindAsync(id); |
{ |
||||
} |
return await context.Children.FindAsync(id); |
||||
|
} |
||||
public List<Child> GetChildren(int parentId) |
|
||||
{ |
public List<Child> GetChildren(int parentId) |
||||
return (from c in context.Children where c.ParentId == parentId select c).ToList(); |
{ |
||||
} |
return (from c in context.Children where c.ParentId == parentId select c).ToList(); |
||||
|
} |
||||
public async Task UpdateChild(int id, Child newData) |
|
||||
{ |
public async Task UpdateChild(int id, Child newData) |
||||
Child child = await context.Children.FindAsync(id); |
{ |
||||
context.Entry(child).State = Microsoft.EntityFrameworkCore.EntityState.Modified; |
Child child = await context.Children.FindAsync(id); |
||||
PropertyCopier<Child>.Copy(newData, child); |
context.Entry(child).State = Microsoft.EntityFrameworkCore.EntityState.Modified; |
||||
await context.SaveChangesAsync(); |
PropertyCopier<Child>.Copy(newData, child); |
||||
} |
await context.SaveChangesAsync(); |
||||
} |
} |
||||
} |
|
||||
|
public bool IsAuthorized(Session s, Child c) |
||||
|
{ |
||||
|
if (s.Id == c.DoctorId || s.Id == c.ParentId) return true; |
||||
|
// Ha van olyan Asszisztens, akinek;
|
||||
|
// - a dokija egyezik az ügy dokijával
|
||||
|
// - azonosítója a bejelentezett user azonosítója
|
||||
|
return (context.Assistants.Any(a => a.Id == s.Id && a.DoctorId == c.DoctorId)); |
||||
|
} |
||||
|
} |
||||
|
} |
||||
|
Loading…
Reference in new issue