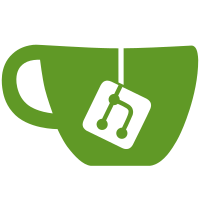
63 changed files with 1748 additions and 630 deletions
@ -0,0 +1,17 @@ |
|||
using System; |
|||
using System.Collections.Generic; |
|||
using System.Linq; |
|||
using System.Threading.Tasks; |
|||
|
|||
namespace CoviDok.Api |
|||
{ |
|||
public enum Errors |
|||
{ |
|||
Uauthorized, |
|||
PasswordNotComplex, |
|||
EmailTaken, |
|||
EmailNotValid, |
|||
SSNExists, |
|||
BadImageID |
|||
} |
|||
} |
@ -0,0 +1,20 @@ |
|||
using System; |
|||
using System.Collections.Generic; |
|||
using System.Linq; |
|||
using System.Threading.Tasks; |
|||
|
|||
namespace CoviDok.Api.Objects |
|||
{ |
|||
public class PublicChild |
|||
{ |
|||
public string SessionID { get; set; } |
|||
public int ID { get; set; } |
|||
public string FirstName { get; set; } |
|||
public string LastName { get; set; } |
|||
public int DoctorId { get; set; } |
|||
public int ParentId { get; set; } |
|||
public string SSN { get; set; } |
|||
public DateTime BirthDate { get; set; } |
|||
public string PictureId { get; set; } |
|||
} |
|||
} |
@ -0,0 +1,21 @@ |
|||
using System; |
|||
using System.Collections.Generic; |
|||
using System.Linq; |
|||
using System.Threading.Tasks; |
|||
|
|||
namespace CoviDok.Api.Objects |
|||
{ |
|||
public class PublicDoctor |
|||
{ |
|||
public string SessionID { get; set; } |
|||
public int ID { get; set; } |
|||
public string FirstName { get; set; } |
|||
public string LastName { get; set; } |
|||
|
|||
public string Email { get; set; } |
|||
public ICollection<int> Children { get; set; } = new List<int>(); |
|||
|
|||
public ICollection<int> Assistants { get; set; } = new List<int>(); |
|||
} |
|||
|
|||
} |
@ -0,0 +1,17 @@ |
|||
using System; |
|||
using System.Collections.Generic; |
|||
using System.Linq; |
|||
using System.Threading.Tasks; |
|||
|
|||
namespace CoviDok.Api.Objects |
|||
{ |
|||
public class PublicParent |
|||
{ |
|||
public string SessionID { get; set; } |
|||
public int ID { get; set; } |
|||
public string FirstName { get; set; } |
|||
public string LastName { get; set; } |
|||
public string Email { get; set; } |
|||
public ICollection<PublicChild> Children { get; set; } = new List<PublicChild>(); |
|||
} |
|||
} |
@ -0,0 +1,16 @@ |
|||
using System; |
|||
using System.Collections.Generic; |
|||
using System.Linq; |
|||
using System.Threading.Tasks; |
|||
|
|||
namespace CoviDok.Api.Request |
|||
{ |
|||
public class CaseCreate |
|||
{ |
|||
public string SessionID { get; set; } |
|||
public int DoctorID { get; set; } |
|||
public int ChildID { get; set; } |
|||
public DateTime StartDate { get; set; } |
|||
public string Title { get; set; } |
|||
} |
|||
} |
@ -1,17 +0,0 @@ |
|||
using CoviDok.data; |
|||
using System; |
|||
using System.Collections.Generic; |
|||
using System.Linq; |
|||
using System.Threading.Tasks; |
|||
|
|||
namespace CoviDok.Api.Response |
|||
{ |
|||
public class FilteredCases |
|||
{ |
|||
public ICollection<Case> Cases { get; set; } |
|||
public FilteredCases() |
|||
{ |
|||
Cases = new List<Case>(); |
|||
} |
|||
} |
|||
} |
@ -1,50 +0,0 @@ |
|||
using Minio; |
|||
using Minio.Exceptions; |
|||
using System; |
|||
using System.Collections.Generic; |
|||
using System.IO; |
|||
using System.Linq; |
|||
using System.Threading.Tasks; |
|||
|
|||
namespace CoviDok.BLL |
|||
{ |
|||
class MinioHandler |
|||
{ |
|||
private readonly MinioClient Client = null; |
|||
|
|||
public MinioHandler(string Host, string AccessKey, string SecretKey) |
|||
{ |
|||
//Client = new MinioClient(
|
|||
//"192.168.0.160:9000",
|
|||
//"secretaccesskey",
|
|||
//"secretsecretkey");
|
|||
Client = new MinioClient(Host, AccessKey, SecretKey); |
|||
} |
|||
|
|||
public async Task<MinioResult> UploadImage(string BucketName, Stream FilePath, long size, string ObjectName) |
|||
{ |
|||
try |
|||
{ |
|||
// Make a bucket on the server, if not already present.
|
|||
bool found = await Client.BucketExistsAsync(BucketName); |
|||
if (!found) |
|||
{ |
|||
await Client.MakeBucketAsync(BucketName); |
|||
} |
|||
// Upload a file to bucket.
|
|||
await Client.PutObjectAsync(BucketName, ObjectName, FilePath, size); |
|||
return new MinioResult(true, BucketName + ":" + ObjectName); |
|||
} |
|||
catch (MinioException e) |
|||
{ |
|||
return new MinioResult(false, e.Message); |
|||
} |
|||
} |
|||
|
|||
public async Task GetImage(string ImageID, Action<Stream> callback) |
|||
{ |
|||
string[] attrs = ImageID.Split(":"); |
|||
await Client.GetObjectAsync(attrs[0], attrs[1], callback); |
|||
} |
|||
} |
|||
} |
@ -0,0 +1,53 @@ |
|||
using System; |
|||
using System.Collections.Generic; |
|||
using System.Linq; |
|||
using System.Reflection; |
|||
using System.Threading.Tasks; |
|||
|
|||
namespace CoviDok.BLL |
|||
{ |
|||
public class PropertyCopier<T> where T : class |
|||
{ |
|||
public static void Copy(T source, T dest, string[] except = null) |
|||
{ |
|||
List<string> forbidden = new List<string>(except); |
|||
PropertyInfo[] properties = source.GetType().GetProperties(); |
|||
foreach (var property in properties) |
|||
{ |
|||
if (property.Name.ToLower() != "id" && !forbidden.Contains(property.Name.ToLower())) { |
|||
var val = property.GetValue(source); |
|||
if (val != null) |
|||
{ |
|||
property.SetValue(dest, val); |
|||
} |
|||
} |
|||
} |
|||
} |
|||
} |
|||
|
|||
public class PropertyCopier<TSource, TDest> where TSource : class |
|||
where TDest : class |
|||
{ |
|||
public static void Copy(TSource source, TDest dest) |
|||
{ |
|||
var sourceProperties = source.GetType().GetProperties(); |
|||
var destProperties = dest.GetType().GetProperties(); |
|||
|
|||
foreach (var sourceProperty in sourceProperties) |
|||
{ |
|||
foreach (var destProperty in destProperties) |
|||
{ |
|||
if (sourceProperty.Name == destProperty.Name && sourceProperty.PropertyType == destProperty.PropertyType) |
|||
{ |
|||
if (sourceProperty.Name.ToLower() != "id") |
|||
{ |
|||
var val = sourceProperty.GetValue(source); |
|||
if (val != null) destProperty.SetValue(dest, val); |
|||
} |
|||
break; |
|||
} |
|||
} |
|||
} |
|||
} |
|||
} |
|||
} |
@ -1,47 +0,0 @@ |
|||
using System; |
|||
using System.Collections.Generic; |
|||
using System.Text; |
|||
using System.Text.Json; |
|||
using CoviDok.Api; |
|||
using NCuid; |
|||
|
|||
namespace CoviDok.BLL |
|||
{ |
|||
class SessionHandler |
|||
{ |
|||
private readonly ISessionProvider SessionStore; |
|||
|
|||
public SessionHandler(ISessionProvider Provider) |
|||
{ |
|||
SessionStore = Provider; |
|||
} |
|||
|
|||
public Session GetSession(string SessionID) |
|||
{ |
|||
string Candidate = SessionStore.Get(SessionID); |
|||
if (Candidate == null) return null; |
|||
Session session = JsonSerializer.Deserialize<Session>(Candidate); |
|||
session.LastAccess = DateTime.Now; |
|||
SessionStore.Set(SessionID, JsonSerializer.Serialize(session)); |
|||
return session; |
|||
} |
|||
|
|||
public string CreateSession(Role UserType, string UserID) |
|||
{ |
|||
Session session = new Session |
|||
{ |
|||
ID = UserID, |
|||
Type = UserType, |
|||
LastAccess = DateTime.Now |
|||
}; |
|||
string ID = Cuid.Generate(); |
|||
SessionStore.Set(ID, JsonSerializer.Serialize(session)); |
|||
return ID; |
|||
} |
|||
|
|||
public void DeleteSession(string SessionID) |
|||
{ |
|||
SessionStore.Del(SessionID); |
|||
} |
|||
} |
|||
} |
@ -0,0 +1,54 @@ |
|||
using System; |
|||
using System.Collections.Generic; |
|||
using System.Text; |
|||
using System.Text.Json; |
|||
using System.Threading.Tasks; |
|||
using CoviDok.Api; |
|||
using NCuid; |
|||
|
|||
namespace CoviDok.BLL.Sessions |
|||
{ |
|||
class SessionHandler |
|||
{ |
|||
private readonly ISessionProvider SessionStore; |
|||
|
|||
public SessionHandler(ISessionProvider Provider) |
|||
{ |
|||
SessionStore = Provider; |
|||
} |
|||
|
|||
public async Task<Session> GetSession(string SessionID) |
|||
{ |
|||
string Candidate = SessionStore.Get(SessionID); |
|||
if (Candidate == null) return null; |
|||
Session session = null; |
|||
await Task.Run(() => { |
|||
session = JsonSerializer.Deserialize<Session>(Candidate); |
|||
session.LastAccess = DateTime.Now; |
|||
SessionStore.Set(SessionID, JsonSerializer.Serialize(session)); |
|||
}); |
|||
return session; |
|||
} |
|||
|
|||
public async Task<string> CreateSession(Role UserType, int UserID) |
|||
{ |
|||
string ID = null; |
|||
await Task.Run(() => { |
|||
Session session = new Session |
|||
{ |
|||
ID = UserID, |
|||
Type = UserType, |
|||
LastAccess = DateTime.Now |
|||
}; |
|||
ID = Cuid.Generate(); |
|||
SessionStore.Set(ID, JsonSerializer.Serialize(session)); |
|||
}); |
|||
return ID; |
|||
} |
|||
|
|||
public void DeleteSession(string SessionID) |
|||
{ |
|||
SessionStore.Del(SessionID); |
|||
} |
|||
} |
|||
} |
@ -0,0 +1,17 @@ |
|||
using System; |
|||
using System.Collections.Generic; |
|||
using System.IO; |
|||
using System.Linq; |
|||
using System.Threading.Tasks; |
|||
|
|||
namespace CoviDok.BLL.Storage |
|||
{ |
|||
// Interface for namespaced storage
|
|||
interface IStorageProvider |
|||
{ |
|||
public Task<bool> NamespaceExists(string ns); |
|||
public Task CreateNamespace(string ns); |
|||
public Task Upload(string ns, string objectname, Stream data, long size); |
|||
public Task Download(string ns, string objectname, Action<Stream> callback); |
|||
} |
|||
} |
@ -0,0 +1,47 @@ |
|||
using System; |
|||
using System.Collections.Generic; |
|||
using System.IO; |
|||
using System.Linq; |
|||
using System.Threading.Tasks; |
|||
|
|||
namespace CoviDok.BLL.Storage |
|||
{ |
|||
class StorageHandler |
|||
{ |
|||
private readonly IStorageProvider storageProvider; |
|||
|
|||
public StorageHandler(IStorageProvider provider) |
|||
{ |
|||
//Client = new MinioClient(
|
|||
//"192.168.0.160:9000",
|
|||
//"secretaccesskey",
|
|||
//"secretsecretkey");
|
|||
storageProvider = provider; |
|||
} |
|||
|
|||
public async Task<StorageResult> UploadImage(string BucketName, Stream FilePath, long size, string ObjectName) |
|||
{ |
|||
try |
|||
{ |
|||
// Make a bucket on the server, if not already present.
|
|||
bool found = await storageProvider.NamespaceExists(BucketName); |
|||
if (!found) |
|||
{ |
|||
await storageProvider.CreateNamespace(BucketName); |
|||
} |
|||
// Upload a file to bucket.
|
|||
await storageProvider.Upload(BucketName, ObjectName, FilePath, size); |
|||
return new StorageResult(true, BucketName + ":" + ObjectName); |
|||
} |
|||
catch (Exception e) |
|||
{ |
|||
return new StorageResult(false, e.Message); |
|||
} |
|||
} |
|||
|
|||
public async Task GetImage(string bucketName, string ImageID, Action<Stream> callback) |
|||
{ |
|||
await storageProvider.Download(bucketName, ImageID, callback); |
|||
} |
|||
} |
|||
} |
@ -1,11 +1,11 @@ |
|||
namespace CoviDok.BLL |
|||
{ |
|||
public class MinioResult |
|||
public class StorageResult |
|||
{ |
|||
public readonly bool Success; |
|||
public readonly string Data; |
|||
|
|||
public MinioResult(bool Success, string Data) |
|||
public StorageResult(bool Success, string Data) |
|||
{ |
|||
this.Success = Success; |
|||
this.Data = Data; |
@ -1,78 +0,0 @@ |
|||
using CoviDok.data; |
|||
using System; |
|||
using System.Collections.Generic; |
|||
using System.Linq; |
|||
using System.Threading.Tasks; |
|||
|
|||
namespace CoviDok.BLL |
|||
{ |
|||
public class Tools |
|||
{ |
|||
|
|||
private static Random random = new Random(); |
|||
public static string RandomString(int length) |
|||
{ |
|||
const string chars = "ABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789"; |
|||
return new string(Enumerable.Repeat(chars, length) |
|||
.Select(s => s[random.Next(s.Length)]).ToArray()); |
|||
} |
|||
|
|||
static T RandomEnumValue<T>() |
|||
{ |
|||
var v = Enum.GetValues(typeof(T)); |
|||
return (T)v.GetValue(random.Next(v.Length)); |
|||
} |
|||
|
|||
public static Case MockCase(Dictionary<string, string> filters) |
|||
{ |
|||
Case c = new Case |
|||
{ |
|||
Id = RandomString(8) |
|||
}; |
|||
if (filters.ContainsKey("DoctorID")) |
|||
{ |
|||
c.DoctorID = filters["DoctorID"]; |
|||
} |
|||
else |
|||
{ |
|||
c.DoctorID = Tools.RandomString(10); |
|||
} |
|||
if (filters.ContainsKey("ParentID")) |
|||
{ |
|||
c.ParentID = filters["ParentID"]; |
|||
} |
|||
else |
|||
{ |
|||
c.ParentID = Tools.RandomString(10); |
|||
} |
|||
if (filters.ContainsKey("ChildID")) |
|||
{ |
|||
c.ChildID = filters["ChildID"]; |
|||
} |
|||
else |
|||
{ |
|||
c.ChildID = Tools.RandomString(10); |
|||
} |
|||
if (filters.ContainsKey("CaseStatus")) |
|||
{ |
|||
c.ChildID = filters["CaseStatus"]; |
|||
} |
|||
else |
|||
{ |
|||
c.ChildID = RandomEnumValue<CaseStatus>().ToString(); |
|||
} |
|||
int msgDb = random.Next(10); |
|||
for (int i = 0; i < msgDb; i++) |
|||
{ |
|||
Update u = new Update |
|||
{ |
|||
Content = RandomString(55), |
|||
Sender = RandomString(13), |
|||
Id = RandomString(8) |
|||
}; |
|||
c.Updates.Add(u); |
|||
} |
|||
return c; |
|||
} |
|||
} |
|||
} |
@ -1,11 +1,118 @@ |
|||
using System; |
|||
using CoviDok.Api; |
|||
using CoviDok.Api.Request; |
|||
using CoviDok.Api.Response; |
|||
using CoviDok.Data.MySQL; |
|||
using CoviDok.Data.Model; |
|||
using System; |
|||
using System.Collections.Generic; |
|||
using System.Linq; |
|||
using System.Text.RegularExpressions; |
|||
using System.Threading.Tasks; |
|||
|
|||
namespace CoviDok.BLL.User |
|||
{ |
|||
public class Auth |
|||
{ |
|||
private static string emailRegex = @"(@)(.+)$"; |
|||
private static string pwRegex = @"^(?=.{6,})(?=.*[0-9])(?=.*[a-z])(?=.*[A-Z])(?=.*[@#$%^&+=?!]).*$"; //8 hosszú, kis és nagybetű, különleges karakter
|
|||
private static readonly MySqlContext context = new MySqlContext(); |
|||
|
|||
public static async Task<AuthIdentity> AuthenticateUser(string Email, string Password) |
|||
{ |
|||
if (!Regex.IsMatch(Email, emailRegex, RegexOptions.IgnoreCase)) throw new FormatException(); |
|||
|
|||
List<RoleUser> users = new List<RoleUser>(); |
|||
await Task.Run(() => |
|||
{ |
|||
var us = from u in context.RoleUsers where u.Email == Email select u; |
|||
users.AddRange(us); |
|||
}); |
|||
|
|||
if (users.Count != 1) throw new KeyNotFoundException(); |
|||
RoleUser user = users[0]; |
|||
|
|||
if (user.CheckPassword(Password)) { |
|||
return new AuthIdentity |
|||
{ |
|||
FirstName = user.FirstName, |
|||
LastName = user.LastName, |
|||
Role = user.Role, |
|||
UserId = user.Id |
|||
}; |
|||
} |
|||
return null; |
|||
} |
|||
|
|||
public static bool CheckEmail(string Email) { |
|||
return context.RoleUsers.Any(e => e.Email == Email); |
|||
} |
|||
|
|||
public static GenericResponse ValidateRegistration(string Email, string Password) |
|||
{ |
|||
GenericResponse genericResponse = new GenericResponse(); |
|||
|
|||
if (CheckEmail(Email)) |
|||
{ |
|||
genericResponse.Status = Status.Error; |
|||
genericResponse.Body["reason"] = Email + " is already registered!"; |
|||
return genericResponse; |
|||
} |
|||
if (!Regex.IsMatch(Email, emailRegex, RegexOptions.IgnoreCase)) |
|||
{ |
|||
genericResponse.Status = Status.Error; |
|||
genericResponse.Body["reason"] = Email + " is not a valid email address!"; |
|||
return genericResponse; |
|||
} |
|||
if (!Regex.IsMatch(Password, pwRegex, RegexOptions.IgnoreCase)) { |
|||
genericResponse.Status = Status.Error; |
|||
genericResponse.Body["reason"] = "Password does not meet complexity requirements!"; |
|||
return genericResponse; |
|||
} |
|||
return genericResponse; |
|||
} |
|||
|
|||
public static async Task<GenericResponse> CreateUser(AuthRegistration registration) { |
|||
GenericResponse response = new GenericResponse(); |
|||
switch (registration.Role) |
|||
{ |
|||
case Role.Ast: |
|||
Assistant ast = new Assistant { |
|||
FirstName = registration.FirstName, |
|||
LastName = registration.LastName, |
|||
Email = registration.Email, |
|||
Password = RoleUser.GetHashString(registration.Password), |
|||
Role = Role.Ast |
|||
}; |
|||
context.Assistants.Add(ast); |
|||
await context.SaveChangesAsync(); |
|||
response.Body["id"] = ast.Id.ToString(); |
|||
break; |
|||
case Role.Doc: |
|||
Doctor doc = new Doctor { |
|||
FirstName = registration.FirstName, |
|||
LastName = registration.LastName, |
|||
Email = registration.Email, |
|||
Password = RoleUser.GetHashString(registration.Password), |
|||
Role = Role.Doc }; |
|||
context.Doctors.Add(doc); |
|||
await context.SaveChangesAsync(); |
|||
response.Body["id"] = doc.Id.ToString(); |
|||
break; |
|||
case Role.Par: |
|||
Parent par = new Parent |
|||
{ |
|||
FirstName = registration.FirstName, |
|||
LastName = registration.LastName, |
|||
Email = registration.Email, |
|||
Password = RoleUser.GetHashString(registration.Password), |
|||
Role = Role.Par |
|||
}; |
|||
context.Parents.Add(par); |
|||
await context.SaveChangesAsync(); |
|||
response.Body["id"] = par.Id.ToString(); |
|||
break; |
|||
} |
|||
return response; |
|||
} |
|||
} |
|||
} |
|||
|
@ -0,0 +1,16 @@ |
|||
using CoviDok.Api.Objects; |
|||
using CoviDok.BLL.Sessions; |
|||
using CoviDok.Data.Model; |
|||
using System; |
|||
using System.Collections.Generic; |
|||
using System.Linq; |
|||
using System.Threading.Tasks; |
|||
|
|||
namespace CoviDok.BLL.User.Managers |
|||
{ |
|||
interface IAssistantHandler |
|||
{ |
|||
public Task<Assistant> GetAssistant(int id); |
|||
public Task SetAssistant(int id, Assistant value); |
|||
} |
|||
} |
@ -0,0 +1,23 @@ |
|||
using CoviDok.Api.Request; |
|||
using CoviDok.BLL.User.Managers; |
|||
using CoviDok.Data.Model; |
|||
using System; |
|||
using System.Collections.Generic; |
|||
using System.Linq; |
|||
using System.Threading.Tasks; |
|||
|
|||
namespace CoviDok.BLL.User.Handlers |
|||
{ |
|||
public interface ICaseHandler |
|||
{ |
|||
public Task<List<Case>> Filter(CaseFilter filter); |
|||
public Task<Case> GetCase(int id); |
|||
|
|||
public Task<Case> AddCase(Case c); |
|||
|
|||
public Task UpdateCase(int id, Case Case, Update update); |
|||
|
|||
public Task SetCase(int id, CaseStatus status); |
|||
public bool IsAuthorized(int ID, Case c); |
|||
} |
|||
} |
@ -0,0 +1,17 @@ |
|||
using CoviDok.Api.Objects; |
|||
using CoviDok.BLL.Sessions; |
|||
using CoviDok.Data.Model; |
|||
using System; |
|||
using System.Collections.Generic; |
|||
using System.Linq; |
|||
using System.Threading.Tasks; |
|||
|
|||
namespace CoviDok.BLL.User.Managers |
|||
{ |
|||
interface IChildHandler |
|||
{ |
|||
public Task<Child> GetChild(int id); |
|||
public Task UpdateChild(int id, Child newData); |
|||
public Task<int> AddChild(Child newChild); |
|||
} |
|||
} |
@ -0,0 +1,21 @@ |
|||
using CoviDok.Api.Objects; |
|||
using CoviDok.BLL.Sessions; |
|||
using CoviDok.Data.Model; |
|||
using System; |
|||
using System.Collections.Generic; |
|||
using System.Linq; |
|||
using System.Threading.Tasks; |
|||
|
|||
namespace CoviDok.BLL.User.Managers |
|||
{ |
|||
interface IDoctorHandler |
|||
{ |
|||
public List<Doctor> GetDoctors(); |
|||
public Task<Doctor> GetDoctor(int id); |
|||
public Task UpdateDoctor(int id, Doctor value); |
|||
public List<Assistant> GetAssistants(int id); |
|||
public List<Child> GetChildren(int id); |
|||
|
|||
public bool DoctorExists(int id); |
|||
} |
|||
} |
@ -0,0 +1,19 @@ |
|||
using CoviDok.Api.Objects; |
|||
using CoviDok.BLL.Sessions; |
|||
using CoviDok.Data.Model; |
|||
using System; |
|||
using System.Collections.Generic; |
|||
using System.Linq; |
|||
using System.Threading.Tasks; |
|||
|
|||
namespace CoviDok.BLL.User.Managers |
|||
{ |
|||
interface IParentHandler |
|||
{ |
|||
public Task<Parent> GetParent(int id); |
|||
public Task UpdateParent(int id, Parent value); |
|||
public List<Child> GetChildren(int id); |
|||
|
|||
public bool ParentExists(int id); |
|||
} |
|||
} |
@ -0,0 +1,36 @@ |
|||
using CoviDok.Api.Objects; |
|||
using CoviDok.BLL.Sessions; |
|||
using CoviDok.BLL.User.Managers; |
|||
using CoviDok.Data.MySQL; |
|||
using CoviDok.Data.Model; |
|||
using Microsoft.EntityFrameworkCore; |
|||
using System; |
|||
using System.Collections.Generic; |
|||
using System.Linq; |
|||
using System.Threading.Tasks; |
|||
|
|||
namespace CoviDok.Data.MySQL |
|||
{ |
|||
public class AssistantManager |
|||
{ |
|||
private readonly IAssistantHandler handler = new MySqlAssistantHandler(); |
|||
public async Task<PublicAssistant> GetAssistant(int id) |
|||
{ |
|||
Assistant ast = await handler.GetAssistant(id); |
|||
if (ast == null) throw new KeyNotFoundException(); |
|||
return ast.ToPublic(); |
|||
} |
|||
|
|||
public async Task UpdateAssistant(Session s, int id, PublicAssistant value) |
|||
{ |
|||
if (id != value.ID) throw new FormatException(); |
|||
if (s.ID != id) throw new UnauthorizedAccessException(); |
|||
|
|||
Assistant ast = await handler.GetAssistant(id); |
|||
if (ast == null) throw new KeyNotFoundException(); |
|||
|
|||
ast.UpdateSelf(value); |
|||
await handler.SetAssistant(id, ast); |
|||
} |
|||
} |
|||
} |
@ -0,0 +1,112 @@ |
|||
using CoviDok.Api.Request; |
|||
using CoviDok.BLL.Sessions; |
|||
using CoviDok.BLL.User.Handlers; |
|||
using CoviDok.Data.Model; |
|||
using CoviDok.Data.MySQL; |
|||
using System; |
|||
using System.Collections.Generic; |
|||
using System.Linq; |
|||
using System.Linq.Expressions; |
|||
using System.Threading.Tasks; |
|||
|
|||
namespace CoviDok.BLL.User.Managers |
|||
{ |
|||
public class CaseManager |
|||
{ |
|||
private readonly ICaseHandler handler = new MySqlCaseHandler(); |
|||
|
|||
public async Task<List<Case>> FilterCases(Session s, CaseFilter filter) |
|||
{ |
|||
// TODO: Jogosultságkezelés
|
|||
return await handler.Filter(filter); |
|||
} |
|||
|
|||
public async Task SetCertified(Session s, int id) |
|||
{ |
|||
Case c = await handler.GetCase(id); |
|||
if (c == null) throw new KeyNotFoundException(); |
|||
if (handler.IsAuthorized(s.ID, c) && s.Type == Api.Role.Ast) |
|||
{ |
|||
await handler.SetCase(id, CaseStatus.Cured); |
|||
} |
|||
else |
|||
{ |
|||
throw new UnauthorizedAccessException(); |
|||
} |
|||
} |
|||
|
|||
public async Task SetCured(Session s, int id) |
|||
{ |
|||
Case c = await handler.GetCase(id); |
|||
if (c == null) throw new KeyNotFoundException(); |
|||
if (s.ID == c.DoctorID) |
|||
{ |
|||
await handler.SetCase(id, CaseStatus.Cured); |
|||
} |
|||
else |
|||
{ |
|||
throw new UnauthorizedAccessException(); |
|||
} |
|||
} |
|||
|
|||
|
|||
|
|||
public async Task<Case> GetCase(Session s, int id) |
|||
{ |
|||
// Parent, Doctor, and doctors assistants can access a case
|
|||
Case c = await handler.GetCase(id); |
|||
if (c == null) throw new KeyNotFoundException(); |
|||
if (handler.IsAuthorized(s.ID, c)) |
|||
{ |
|||
return c; |
|||
} |
|||
else |
|||
{ |
|||
throw new UnauthorizedAccessException(); |
|||
} |
|||
} |
|||
|
|||
public async Task<Case> CreateCase(Session s, int DoctorID, int ChildID, string Title, DateTime startDate) |
|||
{ |
|||
// TODO szülő csak saját gyereket jelenthet
|
|||
Case c = new Case { |
|||
StartDate = startDate, |
|||
ChildID = ChildID, |
|||
ParentID = s.ID, |
|||
DoctorID = DoctorID, |
|||
Title = Title, |
|||
CreatedDate = DateTime.Now, |
|||
LastModificationDate = DateTime.Now, |
|||
CaseStatus = CaseStatus.InProgress, |
|||
Assignee = s.ID |
|||
}; |
|||
return await handler.AddCase(c); |
|||
} |
|||
|
|||
public async Task UpdateCase(Session s, int id, string updateMsg, List<string> Images) |
|||
{ |
|||
Case c = await handler.GetCase(id); |
|||
if (c == null) throw new KeyNotFoundException("Case ID not found: " + id); |
|||
if (handler.IsAuthorized(s.ID, c)) |
|||
{ |
|||
if (c.CaseStatus == CaseStatus.Certified) throw new ArgumentException("Can't modify closed Case!"); |
|||
|
|||
if (s.ID == c.ParentID) c.Assignee = c.DoctorID; // Ha szülő updatel, az assignee az orvos lesz
|
|||
if (s.ID == c.DoctorID) c.Assignee = c.ParentID; // Ha doki frissít, a szülőhöz kerül
|
|||
|
|||
c.LastModificationDate = DateTime.Now; |
|||
Update update = new Update { |
|||
Sender = s.ID, |
|||
Content = updateMsg, |
|||
CreatedDate = DateTime.Now, |
|||
Images = Images |
|||
}; |
|||
await handler.UpdateCase(id, c, update); |
|||
} |
|||
else |
|||
{ |
|||
throw new UnauthorizedAccessException(); |
|||
} |
|||
} |
|||
} |
|||
} |
@ -0,0 +1,56 @@ |
|||
using CoviDok.Api.Objects; |
|||
using CoviDok.BLL.Sessions; |
|||
using CoviDok.BLL.User.Managers; |
|||
using CoviDok.Data.Model; |
|||
using CoviDok.Data.MySQL; |
|||
using Microsoft.EntityFrameworkCore; |
|||
using System; |
|||
using System.Collections.Generic; |
|||
using System.Linq; |
|||
using System.Threading.Tasks; |
|||
|
|||
namespace CoviDok.Data.MySQL |
|||
{ |
|||
public class ChildManager |
|||
{ |
|||
private readonly IChildHandler handler; |
|||
public async Task<PublicChild> GetChild(Session s, int id) |
|||
{ |
|||
Child child = await handler.GetChild(id); |
|||
if (child == null) throw new KeyNotFoundException(); |
|||
if (child.DoctorId == s.ID || child.ParentId == s.ID) |
|||
{ |
|||
return child.ToPublic(); |
|||
} |
|||
else { |
|||
throw new UnauthorizedAccessException(); |
|||
} |
|||
} |
|||
|
|||
public async Task UpdateChild(Session s, int id, PublicChild newData) |
|||
{ |
|||
if (id != newData.ID) throw new FormatException(); |
|||
|
|||
Child child = await handler.GetChild(id); |
|||
if (child == null) throw new KeyNotFoundException(); |
|||
if (child.ParentId == s.ID || child.DoctorId == s.ID) |
|||
{ |
|||
child.UpdateSelf(newData); |
|||
await handler.UpdateChild(id, child); |
|||
} |
|||
else |
|||
{ |
|||
throw new UnauthorizedAccessException(); |
|||
} |
|||
} |
|||
|
|||
public async Task<int> AddChild(Session s, PublicChild newChild) |
|||
{ |
|||
if (s.ID != newChild.ParentId) throw new UnauthorizedAccessException(); |
|||
Child child = new Child(); |
|||
child.UpdateSelf(newChild); |
|||
child.RegistrationDate = DateTime.Now; |
|||
return await handler.AddChild(child); |
|||
} |
|||
} |
|||
} |
@ -0,0 +1,79 @@ |
|||
using CoviDok.Api.Objects; |
|||
using CoviDok.BLL.Sessions; |
|||
using CoviDok.BLL.User.Managers; |
|||
using CoviDok.Data.MySQL; |
|||
using CoviDok.Data.Model; |
|||
using Microsoft.EntityFrameworkCore; |
|||
using System; |
|||
using System.Collections.Generic; |
|||
using System.Linq; |
|||
using System.Security.Policy; |
|||
using System.Threading.Tasks; |
|||
|
|||
namespace CoviDok.Data.MySQL |
|||
{ |
|||
public class DoctorManager |
|||
{ |
|||
private readonly IDoctorHandler handler = new MySqlDoctorHandler(); |
|||
public async Task<List<PublicDoctor>> GetDoctors() |
|||
{ |
|||
|
|||
List<PublicDoctor> ret = new List<PublicDoctor>(); |
|||
await Task.Run( () => { |
|||
var docs = handler.GetDoctors(); |
|||
foreach (Doctor doctor in docs) |
|||
{ |
|||
ret.Add(doctor.ToPublic()); |
|||
} |
|||
}); |
|||
return ret; |
|||
} |
|||
|
|||
public async Task<PublicDoctor> GetDoctor(int id) |
|||
{ |
|||
Doctor doc = await handler.GetDoctor(id); |
|||
if (doc == null) throw new KeyNotFoundException(); |
|||
return doc.ToPublic(); |
|||
} |
|||
|
|||
public async Task UpdateDoctor(Session s, int id, PublicDoctor value) |
|||
{ |
|||
if (id != value.ID) throw new FormatException(); |
|||
if (s.ID != id) throw new UnauthorizedAccessException(); |
|||
|
|||
Doctor doc = await handler.GetDoctor(id); |
|||
if (doc == null) throw new KeyNotFoundException(); |
|||
|
|||
doc.UpdateSelf(value); |
|||
await handler.UpdateDoctor(id, doc); |
|||
} |
|||
|
|||
public async Task<List<PublicAssistant>> GetAssistants(int id) |
|||
{ |
|||
if (!handler.DoctorExists(id)) throw new KeyNotFoundException(); |
|||
List<PublicAssistant> ret = new List<PublicAssistant>(); |
|||
await Task.Run(() => { |
|||
var asts = handler.GetAssistants(id); |
|||
foreach (Assistant assistant in asts) |
|||
{ |
|||
ret.Add(assistant.ToPublic()); |
|||
} |
|||
}); |
|||
return ret; |
|||
} |
|||
|
|||
public async Task<List<PublicChild>> GetChildren(int id) |
|||
{ |
|||
if (!handler.DoctorExists(id)) throw new KeyNotFoundException(); |
|||
List<PublicChild> ret = new List<PublicChild>(); |
|||
await Task.Run(() => { |
|||
var asts = handler.GetChildren(id); |
|||
foreach (Child child in asts) |
|||
{ |
|||
ret.Add(child.ToPublic()); |
|||
} |
|||
}); |
|||
return ret; |
|||
} |
|||
} |
|||
} |
@ -0,0 +1,50 @@ |
|||
using CoviDok.Api.Objects; |
|||
using CoviDok.BLL.Sessions; |
|||
using CoviDok.BLL.User.Managers; |
|||
using CoviDok.Data.Model; |
|||
using Microsoft.AspNetCore.Mvc; |
|||
using Microsoft.EntityFrameworkCore; |
|||
using System; |
|||
using System.Collections.Generic; |
|||
using System.Linq; |
|||
using System.Threading.Tasks; |
|||
|
|||
namespace CoviDok.Data.MySQL |
|||
{ |
|||
public class ParentManager |
|||
{ |
|||
private readonly IParentHandler handler = new MySqlParentHandler(); |
|||
public async Task<PublicParent> GetParent(int id) |
|||
{ |
|||
Parent parent = await handler.GetParent(id); |
|||
if (parent == null) throw new KeyNotFoundException(); |
|||
return parent.ToPublic(); |
|||
} |
|||
|
|||
public async Task UpdateParent(Session s, int id, PublicParent value) |
|||
{ |
|||
if (id != value.ID) throw new FormatException(); |
|||
if (s.ID != id) throw new UnauthorizedAccessException(); |
|||
|
|||
Parent parent = await handler.GetParent(id); |
|||
if (parent == null) throw new KeyNotFoundException(); |
|||
|
|||
parent.UpdateSelf(value); |
|||
await handler.UpdateParent(id, parent); |
|||
} |
|||
|
|||
public async Task<List<PublicChild>> GetChildren(int id) |
|||
{ |
|||
if (!handler.ParentExists(id)) throw new KeyNotFoundException(); |
|||
List<PublicChild> ret = new List<PublicChild>(); |
|||
await Task.Run(() => { |
|||
var asts = handler.GetChildren(id); |
|||
foreach (Child child in asts) |
|||
{ |
|||
ret.Add(child.ToPublic()); |
|||
} |
|||
}); |
|||
return ret; |
|||
} |
|||
} |
|||
} |
@ -0,0 +1,61 @@ |
|||
using System; |
|||
using System.Collections.Generic; |
|||
using System.Linq; |
|||
using System.Threading.Tasks; |
|||
using CoviDok.Api.Objects; |
|||
using CoviDok.BLL.Sessions; |
|||
using CoviDok.BLL.User.Managers; |
|||
using CoviDok.Data.MySQL; |
|||
using CoviDok.Data.SessionProviders; |
|||
using Microsoft.AspNetCore.Http; |
|||
using Microsoft.AspNetCore.Mvc; |
|||
|
|||
namespace CoviDok.Controllers |
|||
{ |
|||
[Route("api/[controller]")]
|
|||
[ApiController] |
|||
public class AssistantController : ControllerBase |
|||
{ |
|||
private readonly SessionHandler Handler = new SessionHandler(new RedisProvider("redis")); |
|||
|
|||
private readonly AssistantManager mgr = new AssistantManager(); |
|||
|
|||
|
|||
[HttpGet("{id}")] |
|||
public async Task<ActionResult<PublicAssistant>> GetDoctor(int id) |
|||
{ |
|||
try |
|||
{ |
|||
return await mgr.GetAssistant(id); |
|||
} |
|||
catch (KeyNotFoundException) |
|||
{ |
|||
return NotFound(); |
|||
} |
|||
} |
|||
|
|||
[HttpPut("{id}")] |
|||
public async Task<IActionResult> PutAssistant(int id, PublicAssistant ast) |
|||
{ |
|||
Session s = await Handler.GetSession(ast.SessionID); |
|||
if (s == null) return Unauthorized(); |
|||
try |
|||
{ |
|||
await mgr.UpdateAssistant(s, id, ast); |
|||
return NoContent(); |
|||
} |
|||
catch (UnauthorizedAccessException) |
|||
{ |
|||
return Unauthorized(); |
|||
} |
|||
catch (KeyNotFoundException) |
|||
{ |
|||
return NotFound(); |
|||
} |
|||
catch (FormatException) |
|||
{ |
|||
return BadRequest(); |
|||
} |
|||
} |
|||
} |
|||
} |
@ -0,0 +1,87 @@ |
|||
using System; |
|||
using System.Collections.Generic; |
|||
using System.Linq; |
|||
using System.Threading.Tasks; |
|||
using Microsoft.AspNetCore.Http; |
|||
using Microsoft.AspNetCore.Mvc; |
|||
using CoviDok.Api.Objects; |
|||
using CoviDok.Api.Request; |
|||
using CoviDok.BLL.User.Managers; |
|||
using CoviDok.BLL.Sessions; |
|||
using CoviDok.Data.SessionProviders; |
|||
using CoviDok.Data.MySQL; |
|||
|
|||
namespace CoviDok.Controllers |
|||
{ |
|||
[Route("api/[controller]")]
|
|||
[ApiController] |
|||
public class ChildController : ControllerBase |
|||
{ |
|||
private readonly SessionHandler Handler = new SessionHandler(new RedisProvider("redis")); |
|||
|
|||
private readonly ChildManager ChildManager = new ChildManager(); |
|||
|
|||
// POST: api/Child/5
|
|||
[HttpPost("{id}")] |
|||
public async Task<ActionResult<PublicChild>> GetPublicChild(int id, AuthGet get) |
|||
{ |
|||
Session s = await Handler.GetSession(get.SessionID); |
|||
if (s == null) return Unauthorized(); |
|||
|
|||
try |
|||
{ |
|||
return await ChildManager.GetChild(s, id); |
|||
} |
|||
catch (UnauthorizedAccessException) |
|||
{ |
|||
return Unauthorized(); |
|||
} |
|||
catch (KeyNotFoundException) |
|||
{ |
|||
return NotFound(); |
|||
} |
|||
} |
|||
|
|||
// PUT: api/Child/5
|
|||
// To protect from overposting attacks, enable the specific properties you want to bind to, for
|
|||
// more details, see https://go.microsoft.com/fwlink/?linkid=2123754.
|
|||
[HttpPut("{id}")] |
|||
public async Task<IActionResult> PutPublicChild(int id, PublicChild publicChild) |
|||
{ |
|||
Session s = await Handler.GetSession(publicChild.SessionID); |
|||
if (s == null) return Unauthorized(); |
|||
try { |
|||
await ChildManager.UpdateChild(s, id, publicChild); |
|||
return NoContent(); |
|||
} |
|||
catch (UnauthorizedAccessException) { |
|||
return Unauthorized(); |
|||
} |
|||
catch (KeyNotFoundException) { |
|||
return NotFound(); |
|||
} |
|||
catch (FormatException) |
|||
{ |
|||
return BadRequest(); |
|||
} |
|||
} |
|||
|
|||
// POST: api/Child
|
|||
// To protect from overposting attacks, enable the specific properties you want to bind to, for
|
|||
// more details, see https://go.microsoft.com/fwlink/?linkid=2123754.
|
|||
[HttpPost] |
|||
public async Task<ActionResult<PublicChild>> PostPublicChild(PublicChild publicChild) |
|||
{ |
|||
Session s = await Handler.GetSession(publicChild.SessionID); |
|||
if (s == null ) return Unauthorized(); |
|||
|
|||
try { |
|||
int Id = await ChildManager.AddChild(s, publicChild); |
|||
return CreatedAtAction("GetPublicChild", new { id = Id }, publicChild); |
|||
} |
|||
catch (UnauthorizedAccessException) { |
|||
return Unauthorized(); |
|||
} |
|||
} |
|||
} |
|||
} |
@ -1,57 +0,0 @@ |
|||
using System; |
|||
using System.Collections.Generic; |
|||
using System.Linq; |
|||
using System.Threading.Tasks; |
|||
using CoviDok.Api; |
|||
using CoviDok.Api.Request; |
|||
using Microsoft.AspNetCore.Http; |
|||
using Microsoft.AspNetCore.Mvc; |
|||
|
|||
namespace CoviDok.Controllers |
|||
{ |
|||
[Route("api/[controller]")]
|
|||
[ApiController] |
|||
public class DocController : ControllerBase |
|||
{ |
|||
// GET /api/Doc
|
|||
[HttpGet] |
|||
public async Task<ActionResult<GenericResponse>> GetDoctors() |
|||
{ |
|||
GenericResponse genericResponse = new GenericResponse(); |
|||
for (int i=5; i < 15; i++) |
|||
{ |
|||
string doc = "{ \"firstName\": \"Dr. Schanniquah\", \"lastName\": \"The " + i + "th\"}"; |
|||
genericResponse.Body[i.ToString()] = doc; |
|||
} |
|||
return genericResponse; |
|||
} |
|||
|
|||
// GET /api/Doc/{id}/assistants
|
|||
[HttpGet("{id}/assistants")] |
|||
public async Task<ActionResult<GenericResponse>> GetAssistantsOfDoctor(string id) |
|||
{ |
|||
GenericResponse genericResponse = new GenericResponse(); |
|||
genericResponse.Body["doctorID"] = id; |
|||
for (int i = 5; i < 15; i++) |
|||
{ |
|||
string doc = "{ \"firstName\": \"Belisarius\", \"lastName\": \"The " + i + "th Cawl\"}"; |
|||
genericResponse.Body[i.ToString()] = doc; |
|||
} |
|||
return genericResponse; |
|||
} |
|||
|
|||
// GET /api/Doc/{id}/children
|
|||
[HttpGet("{id}/children")] |
|||
public async Task<ActionResult<GenericResponse>> GetChildrenOfDoctor(string id) |
|||
{ |
|||
GenericResponse genericResponse = new GenericResponse(); |
|||
genericResponse.Body["doctorID"] = id; |
|||
for (int i = 5; i < 15; i++) |
|||
{ |
|||
string doc = "{ \"firstName\": \"Belisarius\", \"lastName\": \"The " + i + "th Cawl\"}"; |
|||
genericResponse.Body[i.ToString()] = doc; |
|||
} |
|||
return genericResponse; |
|||
} |
|||
} |
|||
} |
@ -0,0 +1,100 @@ |
|||
using System; |
|||
using System.Collections.Generic; |
|||
using System.Linq; |
|||
using System.Threading.Tasks; |
|||
using CoviDok.Api; |
|||
using CoviDok.Api.Objects; |
|||
using CoviDok.Api.Request; |
|||
using CoviDok.BLL.Sessions; |
|||
using CoviDok.BLL.User.Managers; |
|||
using CoviDok.Data.MySQL; |
|||
using CoviDok.Data.SessionProviders; |
|||
using Microsoft.AspNetCore.Http; |
|||
using Microsoft.AspNetCore.Mvc; |
|||
|
|||
namespace CoviDok.Controllers |
|||
{ |
|||
[Route("api/[controller]")]
|
|||
[ApiController] |
|||
public class DoctorController : ControllerBase |
|||
{ |
|||
private readonly SessionHandler Handler = new SessionHandler(new RedisProvider("redis")); |
|||
|
|||
private readonly DoctorManager doctorHandler = new DoctorManager(); |
|||
// GET /api/Doc
|
|||
[HttpGet] |
|||
public async Task<ActionResult<ICollection<PublicDoctor>>> GetDoctors() |
|||
{ |
|||
return await doctorHandler.GetDoctors(); |
|||
} |
|||
|
|||
[HttpGet("{id}")] |
|||
public async Task<ActionResult<PublicDoctor>> GetDoctor(int id) |
|||
{ |
|||
try |
|||
{ |
|||
return await doctorHandler.GetDoctor(id); |
|||
} |
|||
catch (KeyNotFoundException) |
|||
{ |
|||
return NotFound(); |
|||
} |
|||
} |
|||
|
|||
[HttpPut("{id}")] |
|||
public async Task<IActionResult> PutDoctor(int id, PublicDoctor doctor) { |
|||
Session s = await Handler.GetSession(doctor.SessionID); |
|||
if (s == null) return Unauthorized(); |
|||
try |
|||
{ |
|||
await doctorHandler.UpdateDoctor(s, id, doctor); |
|||
return NoContent(); |
|||
} |
|||
catch (UnauthorizedAccessException) |
|||
{ |
|||
return Unauthorized(); |
|||
} |
|||
catch (KeyNotFoundException) |
|||
{ |
|||
return NotFound(); |
|||
} |
|||
catch (FormatException) |
|||
{ |
|||
return BadRequest(); |
|||
} |
|||
} |
|||
|
|||
// GET /api/Doc/{id}/assistants
|
|||
[HttpGet("{id}/assistants")] |
|||
public async Task<ActionResult<ICollection<PublicAssistant>>> GetAssistantsOfDoctor(int id) |
|||
{ |
|||
try |
|||
{ |
|||
return await doctorHandler.GetAssistants(id); |
|||
} |
|||
catch (KeyNotFoundException) |
|||
{ |
|||
return NotFound(); |
|||
} |
|||
} |
|||
|
|||
// GET /api/Doc/{id}/children
|
|||
[HttpPost("{id}/children")] |
|||
public async Task<ActionResult<ICollection<PublicChild>>> GetChildrenOfDoctor(int id, AuthGet get) |
|||
{ |
|||
Session s = await Handler.GetSession(get.SessionID); |
|||
if (s == null) return Unauthorized(); |
|||
try |
|||
{ |
|||
return await doctorHandler.GetChildren(id); |
|||
} |
|||
catch (KeyNotFoundException) |
|||
{ |
|||
return NotFound(); |
|||
} |
|||
catch (UnauthorizedAccessException) { |
|||
return Unauthorized(); |
|||
} |
|||
} |
|||
} |
|||
} |
@ -1,123 +0,0 @@ |
|||
using System; |
|||
using System.Collections.Generic; |
|||
using System.Linq; |
|||
using System.Threading.Tasks; |
|||
using Microsoft.AspNetCore.Http; |
|||
using Microsoft.AspNetCore.Mvc; |
|||
using Microsoft.EntityFrameworkCore; |
|||
using CoviDok.data; |
|||
|
|||
namespace CoviDok.Controllers |
|||
{ |
|||
[Route("api/[controller]")]
|
|||
[ApiController] |
|||
public class DoctorsController : ControllerBase |
|||
{ |
|||
private readonly MySQLContext _context; |
|||
|
|||
public DoctorsController(MySQLContext context) |
|||
{ |
|||
_context = context; |
|||
} |
|||
|
|||
// GET: api/Doctors
|
|||
[HttpGet] |
|||
public async Task<ActionResult<IEnumerable<Doctor>>> GetDoctors() |
|||
{ |
|||
return await _context.Doctors.ToListAsync(); |
|||
} |
|||
|
|||
// GET: api/Doctors/5
|
|||
[HttpGet("{id}")] |
|||
public async Task<ActionResult<Doctor>> GetDoctor(string id) |
|||
{ |
|||
var doctor = await _context.Doctors.FindAsync(id); |
|||
|
|||
if (doctor == null) |
|||
{ |
|||
return NotFound(); |
|||
} |
|||
|
|||
return doctor; |
|||
} |
|||
|
|||
// PUT: api/Doctors/5
|
|||
// To protect from overposting attacks, enable the specific properties you want to bind to, for
|
|||
// more details, see https://go.microsoft.com/fwlink/?linkid=2123754.
|
|||
[HttpPut("{id}")] |
|||
public async Task<IActionResult> PutDoctor(string id, Doctor doctor) |
|||
{ |
|||
if (id != doctor.Id) |
|||
{ |
|||
return BadRequest(); |
|||
} |
|||
|
|||
_context.Entry(doctor).State = EntityState.Modified; |
|||
|
|||
try |
|||
{ |
|||
await _context.SaveChangesAsync(); |
|||
} |
|||
catch (DbUpdateConcurrencyException) |
|||
{ |
|||
if (!DoctorExists(id)) |
|||
{ |
|||
return NotFound(); |
|||
} |
|||
else |
|||
{ |
|||
throw; |
|||
} |
|||
} |
|||
|
|||
return NoContent(); |
|||
} |
|||
|
|||
// POST: api/Doctors
|
|||
// To protect from overposting attacks, enable the specific properties you want to bind to, for
|
|||
// more details, see https://go.microsoft.com/fwlink/?linkid=2123754.
|
|||
[HttpPost] |
|||
public async Task<ActionResult<Doctor>> PostDoctor(Doctor doctor) |
|||
{ |
|||
_context.Doctors.Add(doctor); |
|||
try |
|||
{ |
|||
await _context.SaveChangesAsync(); |
|||
} |
|||
catch (DbUpdateException) |
|||
{ |
|||
if (DoctorExists(doctor.Id)) |
|||
{ |
|||
return Conflict(); |
|||
} |
|||
else |
|||
{ |
|||
throw; |
|||
} |
|||
} |
|||
|
|||
return CreatedAtAction("GetDoctor", new { id = doctor.Id }, doctor); |
|||
} |
|||
|
|||
// DELETE: api/Doctors/5
|
|||
[HttpDelete("{id}")] |
|||
public async Task<ActionResult<Doctor>> DeleteDoctor(string id) |
|||
{ |
|||
var doctor = await _context.Doctors.FindAsync(id); |
|||
if (doctor == null) |
|||
{ |
|||
return NotFound(); |
|||
} |
|||
|
|||
_context.Doctors.Remove(doctor); |
|||
await _context.SaveChangesAsync(); |
|||
|
|||
return doctor; |
|||
} |
|||
|
|||
private bool DoctorExists(string id) |
|||
{ |
|||
return _context.Doctors.Any(e => e.Id == id); |
|||
} |
|||
} |
|||
} |
@ -0,0 +1,79 @@ |
|||
using System; |
|||
using System.Collections.Generic; |
|||
using System.Linq; |
|||
using System.Threading.Tasks; |
|||
using CoviDok.Api.Objects; |
|||
using CoviDok.Api.Request; |
|||
using CoviDok.BLL.Sessions; |
|||
using CoviDok.BLL.User.Managers; |
|||
using CoviDok.Data.MySQL; |
|||
using CoviDok.Data.SessionProviders; |
|||
using Microsoft.AspNetCore.Http; |
|||
using Microsoft.AspNetCore.Mvc; |
|||
|
|||
namespace CoviDok.Controllers |
|||
{ |
|||
[Route("api/[controller]")]
|
|||
[ApiController] |
|||
public class ParentController : ControllerBase |
|||
{ |
|||
private readonly SessionHandler sessionHandler = new SessionHandler(new RedisProvider("redis")); |
|||
|
|||
private readonly ParentManager parentManager = new ParentManager(); |
|||
|
|||
[HttpPost("{id}")] |
|||
public async Task<ActionResult<PublicParent>> GetParent(int id, AuthGet get) |
|||
{ |
|||
Session s = await sessionHandler.GetSession(get.SessionID); |
|||
if (s == null) return Unauthorized(); |
|||
try { |
|||
return await parentManager.GetParent(id); |
|||
} |
|||
catch (KeyNotFoundException) |
|||
{ |
|||
return NotFound(); |
|||
} |
|||
} |
|||
|
|||
[HttpPut("{id}")] |
|||
public async Task<IActionResult> PutParent(int id, PublicParent parent) |
|||
{ |
|||
Session s = await sessionHandler.GetSession(parent.SessionID); |
|||
if (s == null) return Unauthorized(); |
|||
try { |
|||
await parentManager.UpdateParent(s, id, parent); |
|||
return NoContent(); |
|||
} |
|||
catch (UnauthorizedAccessException) |
|||
{ |
|||
return Unauthorized(); |
|||
} |
|||
catch (KeyNotFoundException) |
|||
{ |
|||
return NotFound(); |
|||
} |
|||
catch (FormatException) |
|||
{ |
|||
return BadRequest(); |
|||
} |
|||
} |
|||
[HttpPost("{id}/children")] |
|||
public async Task<ActionResult<List<PublicChild>>> GetChildrenOfParent(int id, AuthGet get) |
|||
{ |
|||
Session s = await sessionHandler.GetSession(get.SessionID); |
|||
if (s == null) return Unauthorized(); |
|||
try |
|||
{ |
|||
return await parentManager.GetChildren(id); |
|||
} |
|||
catch (KeyNotFoundException) |
|||
{ |
|||
return NotFound(); |
|||
} |
|||
catch (UnauthorizedAccessException) |
|||
{ |
|||
return Unauthorized(); |
|||
} |
|||
} |
|||
} |
|||
} |
@ -0,0 +1,22 @@ |
|||
using CoviDok.Api.Objects; |
|||
using System; |
|||
using System.Collections.Generic; |
|||
using System.Linq; |
|||
using System.Threading.Tasks; |
|||
|
|||
namespace CoviDok.Data.Model |
|||
{ |
|||
public class Assistant : RoleUser |
|||
{ |
|||
public int DoctorId { get; set; } |
|||
|
|||
public void UpdateSelf(PublicAssistant assistant) |
|||
{ |
|||
|
|||
} |
|||
public PublicAssistant ToPublic() |
|||
{ |
|||
throw new NotImplementedException(); |
|||
} |
|||
} |
|||
} |
@ -0,0 +1,40 @@ |
|||
using CoviDok.Api.Objects; |
|||
using System; |
|||
using System.Collections.Generic; |
|||
using System.Linq; |
|||
using System.Threading.Tasks; |
|||
|
|||
namespace CoviDok.Data.Model |
|||
{ |
|||
public class Child : User |
|||
{ |
|||
public int DoctorId { get; set; } |
|||
public int ParentId { get; set; } |
|||
//public ICollection<Case> MedicalHistory { get; set; } = new List<Case>();
|
|||
public string SSN { get; set; } |
|||
|
|||
public PublicChild ToPublic() |
|||
{ |
|||
return new PublicChild |
|||
{ |
|||
FirstName = FirstName, |
|||
LastName = LastName, |
|||
DoctorId = DoctorId, |
|||
ParentId = ParentId, |
|||
SSN = SSN, |
|||
BirthDate = BirthDate, |
|||
PictureId = PictureId |
|||
}; |
|||
} |
|||
public void UpdateSelf(PublicChild newVal) |
|||
{ |
|||
FirstName = newVal.FirstName; |
|||
LastName = newVal.LastName; |
|||
DoctorId = newVal.DoctorId; |
|||
ParentId = newVal.ParentId; |
|||
SSN = newVal.SSN; |
|||
BirthDate = newVal.BirthDate; |
|||
PictureId = newVal.PictureId; |
|||
} |
|||
} |
|||
} |
@ -0,0 +1,24 @@ |
|||
using CoviDok.Api.Objects; |
|||
using System; |
|||
using System.Collections.Generic; |
|||
using System.Linq; |
|||
using System.Threading.Tasks; |
|||
|
|||
namespace CoviDok.Data.Model |
|||
{ |
|||
public class Doctor : RoleUser |
|||
{ |
|||
public ICollection<Child> Children { get; set; } = new List<Child>(); |
|||
|
|||
public ICollection<Assistant> Assistants { get; set; } = new List<Assistant>(); |
|||
|
|||
public void UpdateSelf(PublicDoctor doctor) { |
|||
|
|||
} |
|||
public PublicDoctor ToPublic() |
|||
{ |
|||
throw new NotImplementedException(); |
|||
} |
|||
|
|||
} |
|||
} |
@ -0,0 +1,22 @@ |
|||
using CoviDok.Api.Objects; |
|||
using System; |
|||
using System.Collections.Generic; |
|||
using System.Linq; |
|||
using System.Threading.Tasks; |
|||
|
|||
namespace CoviDok.Data.Model |
|||
{ |
|||
public class Parent : RoleUser |
|||
{ |
|||
public ICollection<Child> Children { get; set; } = new List<Child>(); |
|||
|
|||
public void UpdateSelf(PublicParent doctor) |
|||
{ |
|||
|
|||
} |
|||
public PublicParent ToPublic() |
|||
{ |
|||
throw new NotImplementedException(); |
|||
} |
|||
} |
|||
} |
@ -0,0 +1,23 @@ |
|||
using System; |
|||
using System.Collections.Generic; |
|||
using System.Security.Cryptography; |
|||
using System.Text; |
|||
|
|||
namespace CoviDok.Data.Model |
|||
{ |
|||
public class User |
|||
{ |
|||
public int Id { get; set; } |
|||
public string FirstName { get; set; } |
|||
public string LastName { get; set; } |
|||
|
|||
public DateTime BirthDate { get; set; } |
|||
|
|||
public DateTime RegistrationDate { get; set; } |
|||
public string PictureId { get; set; } |
|||
|
|||
|
|||
} |
|||
|
|||
|
|||
} |
@ -0,0 +1,30 @@ |
|||
using CoviDok.Api.Objects; |
|||
using CoviDok.BLL; |
|||
using CoviDok.BLL.User.Managers; |
|||
using CoviDok.Data.Model; |
|||
using Microsoft.EntityFrameworkCore; |
|||
using System; |
|||
using System.Collections.Generic; |
|||
using System.Linq; |
|||
using System.Threading.Tasks; |
|||
|
|||
namespace CoviDok.Data.MySQL |
|||
{ |
|||
public class MySqlAssistantHandler : IAssistantHandler |
|||
{ |
|||
private readonly MySqlContext context = new MySqlContext(); |
|||
public async Task<Assistant> GetAssistant(int id) |
|||
{ |
|||
return await context.Assistants.FindAsync(id); |
|||
} |
|||
|
|||
public async Task SetAssistant(int id, Assistant value) |
|||
{ |
|||
Assistant ast = new Assistant { Id = id }; |
|||
context.Attach(ast); |
|||
context.Entry(ast).State = EntityState.Modified; |
|||
PropertyCopier<Assistant>.Copy(value, ast); |
|||
await context.SaveChangesAsync(); |
|||
} |
|||
} |
|||
} |
@ -0,0 +1,89 @@ |
|||
using CoviDok.Api.Request; |
|||
using CoviDok.BLL; |
|||
using CoviDok.BLL.User.Handlers; |
|||
using CoviDok.BLL.User.Managers; |
|||
using CoviDok.Data.Model; |
|||
using System; |
|||
using System.Collections.Generic; |
|||
using System.Linq; |
|||
using System.Reflection; |
|||
using System.Threading.Tasks; |
|||
|
|||
namespace CoviDok.Data.MySQL |
|||
{ |
|||
public class MySqlCaseHandler : ICaseHandler |
|||
{ |
|||
private readonly MySqlContext context = new MySqlContext(); |
|||
public async Task<Case> AddCase(Case c) |
|||
{ |
|||
context.Cases.Add(c); |
|||
await context.SaveChangesAsync(); |
|||
return c; |
|||
} |
|||
|
|||
public async Task SetCase(int id, CaseStatus status) |
|||
{ |
|||
Case c = await context.Cases.FindAsync(id); |
|||
c.CaseStatus = status; |
|||
await context.SaveChangesAsync(); |
|||
} |
|||
|
|||
public async Task<List<Case>> Filter(CaseFilter filter) |
|||
{ |
|||
List<Case> ret = new List<Case>(); |
|||
|
|||
await Task.Run(() => { |
|||
var query = from c in context.Cases select c; |
|||
if (filter.ChildID != int.MinValue) |
|||
{ |
|||
query = query.Where(c => c.ChildID == filter.ChildID); |
|||
} |
|||
if (filter.DoctorID != int.MinValue) |
|||
{ |
|||
query = query.Where(c => c.DoctorID == filter.DoctorID); |
|||
} |
|||
if (filter.ParentID != int.MinValue) |
|||
{ |
|||
query = query.Where(c => c.ParentID == filter.ParentID); |
|||
} |
|||
if (filter.Assignee != int.MinValue) |
|||
{ |
|||
query = query.Where(c => c.Assignee == filter.Assignee); |
|||
} |
|||
if (filter.Title != null) |
|||
{ |
|||
query = query.Where(c => c.Title.Contains(filter.Title)); |
|||
} |
|||
ret = query.ToList(); |
|||
}); |
|||
return ret; |
|||
} |
|||
|
|||
public async Task<Case> GetCase(int id) |
|||
{ |
|||
return await context.Cases.FindAsync(id); |
|||
} |
|||
|
|||
public async Task UpdateCase(int id, Case Case, Update update) |
|||
{ |
|||
Case c = new Case { Id = id }; |
|||
context.Attach(c); |
|||
context.Entry(c).State = Microsoft.EntityFrameworkCore.EntityState.Modified; |
|||
string[] forbidden = { "Updates" }; |
|||
PropertyCopier<Case>.Copy(Case, c, forbidden); |
|||
context.Updates.Add(update); |
|||
update.CreatedDate = DateTime.Now; |
|||
c.Updates.Add(update); |
|||
await context.SaveChangesAsync(); |
|||
} |
|||
|
|||
public bool IsAuthorized(int ID, Case c) |
|||
{ |
|||
if (ID == c.DoctorID || ID == c.ParentID) return true; |
|||
// Ha van olyan Asszisztens, akinek;
|
|||
// - a dokija egyezik az ügy dokijával
|
|||
// - azonosítója a bejelentezett user azonosítója
|
|||
return (context.Assistants.Any(a => a.Id == ID && a.DoctorId == c.DoctorID)); |
|||
} |
|||
} |
|||
} |
@ -0,0 +1,35 @@ |
|||
using CoviDok.BLL; |
|||
using CoviDok.BLL.User.Managers; |
|||
using CoviDok.Data.Model; |
|||
using System; |
|||
using System.Collections.Generic; |
|||
using System.Linq; |
|||
using System.Threading.Tasks; |
|||
|
|||
namespace CoviDok.Data.MySQL |
|||
{ |
|||
public class MySqlChildHandler : IChildHandler |
|||
{ |
|||
private readonly MySqlContext context = new MySqlContext(); |
|||
public async Task<int> AddChild(Child child) |
|||
{ |
|||
context.Children.Add(child); |
|||
await context.SaveChangesAsync(); |
|||
return child.Id; |
|||
} |
|||
|
|||
public async Task<Child> GetChild(int id) |
|||
{ |
|||
return await context.Children.FindAsync(id); |
|||
} |
|||
|
|||
public async Task UpdateChild(int id, Child newData) |
|||
{ |
|||
Child child = new Child { Id = id }; |
|||
context.Attach(child); |
|||
context.Entry(child).State = Microsoft.EntityFrameworkCore.EntityState.Modified; |
|||
PropertyCopier<Child>.Copy(newData, child); |
|||
await context.SaveChangesAsync(); |
|||
} |
|||
} |
|||
} |
@ -1,23 +1,25 @@ |
|||
using Microsoft.EntityFrameworkCore; |
|||
using CoviDok.Data.Model; |
|||
using Microsoft.EntityFrameworkCore; |
|||
using System; |
|||
using System.Collections.Generic; |
|||
using System.Linq; |
|||
using System.Security.Cryptography.X509Certificates; |
|||
using System.Threading.Tasks; |
|||
|
|||
namespace CoviDok.data |
|||
namespace CoviDok.Data.MySQL |
|||
{ |
|||
public class MySQLContext : DbContext |
|||
public class MySqlContext : DbContext |
|||
{ |
|||
public DbSet<Case> Cases { get; set; } |
|||
public DbSet<Child> Children { get; set; } |
|||
public DbSet<Assistant> Assistants { get; set; } |
|||
public DbSet<Doctor> Doctors { get; set; } |
|||
public DbSet<Image> Images { get; set; } |
|||
public DbSet<Parent> Parents { get; set; } |
|||
public DbSet<Child> Children { get; set; } |
|||
public DbSet<Case> Cases { get; set; } |
|||
public DbSet<Update> Updates { get; set; } |
|||
|
|||
public DbSet<RoleUser> RoleUsers { get; set; } |
|||
protected override void OnConfiguring(DbContextOptionsBuilder optionsBuilder) |
|||
{ |
|||
optionsBuilder.UseMySQL("server=192.168.0.157;database=covidok;user=covidok;password=covidok"); |
|||
optionsBuilder.UseMySQL("server=mysql;database=covidok;user=covidok;password=covidok"); |
|||
} |
|||
} |
|||
} |
@ -0,0 +1,50 @@ |
|||
using CoviDok.Api.Objects; |
|||
using CoviDok.BLL; |
|||
using CoviDok.BLL.User.Managers; |
|||
using CoviDok.Data.Model; |
|||
using System; |
|||
using System.Collections.Generic; |
|||
using System.Linq; |
|||
using System.Threading.Tasks; |
|||
|
|||
namespace CoviDok.Data.MySQL |
|||
{ |
|||
public class MySqlDoctorHandler : IDoctorHandler |
|||
{ |
|||
private readonly MySqlContext context = new MySqlContext(); |
|||
|
|||
public bool DoctorExists(int id) |
|||
{ |
|||
return context.Doctors.Any(e => e.Id == id); |
|||
} |
|||
|
|||
public List<Assistant> GetAssistants(int id) |
|||
{ |
|||
return (from a in context.Assistants where a.DoctorId == id select a).ToList(); |
|||
} |
|||
|
|||
public List<Child> GetChildren(int id) |
|||
{ |
|||
return (from a in context.Children where a.DoctorId == id select a).ToList(); |
|||
} |
|||
|
|||
public async Task<Doctor> GetDoctor(int id) |
|||
{ |
|||
return await context.Doctors.FindAsync(id); |
|||
} |
|||
|
|||
public List<Doctor> GetDoctors() |
|||
{ |
|||
return context.Doctors.ToList(); |
|||
} |
|||
|
|||
public async Task UpdateDoctor(int id, Doctor value) |
|||
{ |
|||
Doctor doctor = new Doctor { Id = id }; |
|||
context.Attach(doctor); |
|||
context.Entry(doctor).State = Microsoft.EntityFrameworkCore.EntityState.Modified; |
|||
PropertyCopier<Doctor>.Copy(value, doctor); |
|||
await context.SaveChangesAsync(); |
|||
} |
|||
} |
|||
} |
@ -0,0 +1,39 @@ |
|||
using CoviDok.BLL; |
|||
using CoviDok.BLL.User.Managers; |
|||
using CoviDok.Data.Model; |
|||
using System; |
|||
using System.Collections.Generic; |
|||
using System.Linq; |
|||
using System.Linq.Expressions; |
|||
using System.Threading.Tasks; |
|||
|
|||
namespace CoviDok.Data.MySQL |
|||
{ |
|||
public class MySqlParentHandler : IParentHandler |
|||
{ |
|||
private readonly MySqlContext context = new MySqlContext(); |
|||
public List<Child> GetChildren(int id) |
|||
{ |
|||
return (from a in context.Children where a.DoctorId == id select a).ToList(); |
|||
} |
|||
|
|||
public async Task<Parent> GetParent(int id) |
|||
{ |
|||
return await context.Parents.FindAsync(id); |
|||
} |
|||
|
|||
public bool ParentExists(int id) |
|||
{ |
|||
return context.Parents.Any(e => e.Id == id); |
|||
} |
|||
|
|||
public async Task UpdateParent(int id, Parent value) |
|||
{ |
|||
Parent Parent = new Parent { Id = id }; |
|||
context.Attach(Parent); |
|||
context.Entry(Parent).State = Microsoft.EntityFrameworkCore.EntityState.Modified; |
|||
PropertyCopier<Parent>.Copy(value, Parent); |
|||
await context.SaveChangesAsync(); |
|||
} |
|||
} |
|||
} |
@ -1,14 +1,15 @@ |
|||
using System; |
|||
using CoviDok.BLL.Sessions; |
|||
using System; |
|||
using System.Collections.Generic; |
|||
using System.Text; |
|||
|
|||
namespace CoviDok.BLL |
|||
namespace CoviDok.Data.SessionProviders |
|||
{ |
|||
// Class is not thread safe, should only be used for testing
|
|||
class DummyProvider : ISessionProvider |
|||
class DummySessionProvider : ISessionProvider |
|||
{ |
|||
private readonly Dictionary<string, string> dict; |
|||
public DummyProvider() |
|||
public DummySessionProvider() |
|||
{ |
|||
dict = new Dictionary<string, string>(); |
|||
} |
@ -1,9 +1,10 @@ |
|||
using StackExchange.Redis; |
|||
using CoviDok.BLL.Sessions; |
|||
using StackExchange.Redis; |
|||
using System; |
|||
using System.Collections.Generic; |
|||
using System.Text; |
|||
|
|||
namespace CoviDok.BLL |
|||
namespace CoviDok.Data.SessionProviders |
|||
{ |
|||
class RedisProvider : ISessionProvider |
|||
{ |
@ -0,0 +1,39 @@ |
|||
using CoviDok.BLL.Storage; |
|||
using System; |
|||
using System.Collections.Generic; |
|||
using System.IO; |
|||
using System.Linq; |
|||
using System.Threading.Tasks; |
|||
using Minio; |
|||
|
|||
namespace CoviDok.Data.StorageProviders |
|||
{ |
|||
public class MinioProvider : IStorageProvider |
|||
{ |
|||
private readonly MinioClient Client = null; |
|||
public MinioProvider(string Host, string AccessKey, string SecretKey) |
|||
{ |
|||
Client = new MinioClient(Host, AccessKey, SecretKey); |
|||
} |
|||
|
|||
public async Task CreateNamespace(string ns) |
|||
{ |
|||
await Client.MakeBucketAsync(ns); |
|||
} |
|||
|
|||
public async Task Download(string ns, string objectname, Action<Stream> callback) |
|||
{ |
|||
await Client.GetObjectAsync(ns, objectname, callback); |
|||
} |
|||
|
|||
public async Task<bool> NamespaceExists(string ns) |
|||
{ |
|||
return await Client.BucketExistsAsync(ns); |
|||
} |
|||
|
|||
public async Task Upload(string ns, string objectname, Stream data, long size) |
|||
{ |
|||
await Client.PutObjectAsync(ns, objectname, data, size); |
|||
} |
|||
} |
|||
} |
@ -1,17 +0,0 @@ |
|||
using System; |
|||
using System.Collections.Generic; |
|||
using System.Linq; |
|||
using System.Threading.Tasks; |
|||
|
|||
namespace CoviDok.data |
|||
{ |
|||
public class Child |
|||
{ |
|||
public string Id { get; set; } |
|||
public string FirstName { get; set; } |
|||
public string LastName { get; set; } |
|||
public string DoctorId { get; set; } |
|||
public string ParentId { get; set; } |
|||
public ICollection<Case> MedicalHistory { get; } = new List<Case>(); |
|||
} |
|||
} |
@ -1,26 +0,0 @@ |
|||
using System; |
|||
using System.Collections.Generic; |
|||
using System.Linq; |
|||
using System.Threading.Tasks; |
|||
|
|||
namespace CoviDok.data |
|||
{ |
|||
public class Doctor |
|||
{ |
|||
public string Id { get; set; } |
|||
|
|||
public string Email { get; set; } |
|||
|
|||
public string Password { get; set; } |
|||
|
|||
public string FirstName { get; set; } |
|||
|
|||
public string LastName { get; set; } |
|||
|
|||
public DateTime RegistrationDate { get; set; } |
|||
|
|||
public ICollection<Child> Children { get; } = new List<Child>(); |
|||
|
|||
public ICollection<Assistant> Assistants { get; } = new List<Assistant>(); |
|||
} |
|||
} |
@ -1,14 +0,0 @@ |
|||
using System; |
|||
using System.Collections.Generic; |
|||
using System.Linq; |
|||
using System.Threading.Tasks; |
|||
|
|||
namespace CoviDok.data |
|||
{ |
|||
//Store Image ID, get actual content from MinIO backend.
|
|||
public class Image |
|||
{ |
|||
public string Id { get; set; } |
|||
|
|||
} |
|||
} |
@ -1,24 +0,0 @@ |
|||
using System; |
|||
using System.Collections.Generic; |
|||
using System.Linq; |
|||
using System.Threading.Tasks; |
|||
|
|||
namespace CoviDok.data |
|||
{ |
|||
public class Parent |
|||
{ |
|||
public string Id { get; set; } |
|||
|
|||
public string Email { get; set; } |
|||
|
|||
public string Password { get; set; } |
|||
|
|||
public string FirstName { get; set; } |
|||
|
|||
public string LastName { get; set; } |
|||
|
|||
public DateTime RegistrationDate { get; set; } |
|||
|
|||
public ICollection<Child> Children { get; } = new List<Child>(); |
|||
} |
|||
} |
Loading…
Reference in new issue