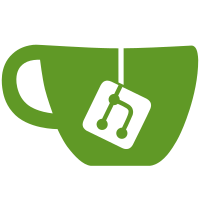
6 changed files with 258 additions and 238 deletions
@ -0,0 +1,14 @@ |
|||
using System; |
|||
using System.Collections.Generic; |
|||
using System.Linq; |
|||
using System.Threading.Tasks; |
|||
|
|||
namespace CoviDok.Api |
|||
{ |
|||
public enum Priority |
|||
{ |
|||
Low, |
|||
Normal, |
|||
High |
|||
} |
|||
} |
@ -1,16 +1,17 @@ |
|||
using System; |
|||
using System.Collections.Generic; |
|||
using System.Linq; |
|||
using System.Threading.Tasks; |
|||
|
|||
namespace CoviDok.Api.Request |
|||
{ |
|||
public class CaseCreate |
|||
{ |
|||
public string SessionId { get; set; } |
|||
public int DoctorId { get; set; } |
|||
public int ChildId { get; set; } |
|||
public DateTime StartDate { get; set; } |
|||
public string Title { get; set; } |
|||
} |
|||
} |
|||
using System; |
|||
using System.Collections.Generic; |
|||
using System.Linq; |
|||
using System.Threading.Tasks; |
|||
|
|||
namespace CoviDok.Api.Request |
|||
{ |
|||
public class CaseCreate |
|||
{ |
|||
public string SessionId { get; set; } |
|||
public int DoctorId { get; set; } |
|||
public int ChildId { get; set; } |
|||
public DateTime StartDate { get; set; } |
|||
public string Title { get; set; } |
|||
public Priority Priority {get; set;} |
|||
} |
|||
} |
|||
|
@ -1,15 +1,16 @@ |
|||
using System; |
|||
using System.Collections.Generic; |
|||
using System.Linq; |
|||
using System.Threading.Tasks; |
|||
|
|||
namespace CoviDok.Api.Request |
|||
{ |
|||
public class CaseUpdate |
|||
{ |
|||
public int CaseId { get; set; } |
|||
public string UpdateMsg { get; set; } |
|||
public List<string> Images {get;set;} |
|||
public string SessionId { get; set; } |
|||
} |
|||
} |
|||
using System; |
|||
using System.Collections.Generic; |
|||
using System.Linq; |
|||
using System.Threading.Tasks; |
|||
|
|||
namespace CoviDok.Api.Request |
|||
{ |
|||
public class CaseUpdate |
|||
{ |
|||
public int CaseId { get; set; } |
|||
public string UpdateMsg { get; set; } |
|||
public List<string> Images {get;set;} |
|||
public string SessionId { get; set; } |
|||
public Priority Priority { get; set; } |
|||
} |
|||
} |
|||
|
@ -1,166 +1,167 @@ |
|||
using CoviDok.Api.Request; |
|||
using CoviDok.BLL.Sessions; |
|||
using CoviDok.BLL.Storage; |
|||
using CoviDok.BLL.User.Handlers; |
|||
using CoviDok.Data.Model; |
|||
using CoviDok.Data.MySQL; |
|||
using CoviDok.Data.StorageProviders; |
|||
using System; |
|||
using System.Collections.Generic; |
|||
using System.Linq; |
|||
using System.Linq.Expressions; |
|||
using System.Threading.Tasks; |
|||
|
|||
namespace CoviDok.BLL.User.Managers |
|||
{ |
|||
public class CaseManager |
|||
{ |
|||
private readonly ICaseHandler handler = new MySqlCaseHandler(); |
|||
|
|||
private readonly StorageHandler storage = new StorageHandler(new MinioProvider()); |
|||
|
|||
public async Task<List<Case>> FilterCases(Session s, CaseFilter filter) |
|||
{ |
|||
// TODO: Jogosultságkezelés
|
|||
return await handler.Filter(filter); |
|||
} |
|||
|
|||
public async Task<List<Update>> GetUpdatesForCase(Session s, int id) |
|||
{ |
|||
Case c = await handler.GetCase(id); |
|||
if (c == null) throw new KeyNotFoundException(); |
|||
if (handler.IsAuthorized(s.Id, c)) |
|||
{ |
|||
return handler.GetUpdatesForCase(id); |
|||
} |
|||
else throw new UnauthorizedAccessException(); |
|||
|
|||
} |
|||
|
|||
public async Task SetCertified(Session s, int id) |
|||
{ |
|||
Case c = await handler.GetCase(id); |
|||
if (c == null) throw new KeyNotFoundException(); |
|||
if (handler.IsAuthorized(s.Id, c) && s.Type == Api.Role.Ast) |
|||
{ |
|||
Update update = new Update { |
|||
CaseId = c.Id, |
|||
SenderId = s.Id, |
|||
SenderRole = s.Type, |
|||
CreatedDate = DateTime.Now, |
|||
Content = "Case set to 'Certified'" |
|||
}; |
|||
await handler.SetCase(id, CaseStatus.Certified, update); |
|||
} |
|||
else |
|||
{ |
|||
throw new UnauthorizedAccessException(); |
|||
} |
|||
} |
|||
|
|||
public async Task SetCured(Session s, int id) |
|||
{ |
|||
Case c = await handler.GetCase(id); |
|||
if (c == null) throw new KeyNotFoundException(); |
|||
if (s.Id == c.DoctorId) |
|||
{ |
|||
Update update = new Update |
|||
{ |
|||
CaseId = c.Id, |
|||
SenderId = s.Id, |
|||
SenderRole = s.Type, |
|||
CreatedDate = DateTime.Now, |
|||
Content = "Case set to 'Cured'" |
|||
}; |
|||
await handler.SetCase(id, CaseStatus.Cured, update); |
|||
} |
|||
else |
|||
{ |
|||
throw new UnauthorizedAccessException(); |
|||
} |
|||
} |
|||
|
|||
public async Task<Update> GetUpdate(Session s, int id) |
|||
{ |
|||
// Parent, Doctor, and doctors assistants can access a case
|
|||
Update u = handler.GetUpadte(id); |
|||
Case c = await handler.GetCase(u.CaseId); |
|||
if (c == null) throw new KeyNotFoundException(); |
|||
if (handler.IsAuthorized(s.Id, c)) |
|||
{ |
|||
return u; |
|||
} |
|||
else |
|||
{ |
|||
throw new UnauthorizedAccessException(); |
|||
} |
|||
} |
|||
|
|||
|
|||
|
|||
public async Task<Case> GetCase(Session s, int id) |
|||
{ |
|||
// Parent, Doctor, and doctors assistants can access a case
|
|||
Case c = await handler.GetCase(id); |
|||
if (c == null) throw new KeyNotFoundException(); |
|||
if (handler.IsAuthorized(s.Id, c)) |
|||
{ |
|||
return c; |
|||
} |
|||
else |
|||
{ |
|||
throw new UnauthorizedAccessException(); |
|||
} |
|||
} |
|||
|
|||
public async Task<Case> CreateCase(Session s, int DoctorId, int ChildId, string Title, DateTime startDate) |
|||
{ |
|||
// TODO szülő csak saját gyereket jelenthet
|
|||
Case c = new Case { |
|||
StartDate = startDate, |
|||
ChildId = ChildId, |
|||
ParentId = s.Id, |
|||
DoctorId = DoctorId, |
|||
Title = Title, |
|||
CreatedDate = DateTime.Now, |
|||
LastModificationDate = DateTime.Now, |
|||
CaseStatus = CaseStatus.InProgress, |
|||
Assignee = s.Id |
|||
}; |
|||
return await handler.AddCase(c); |
|||
} |
|||
|
|||
public async Task UpdateCase(Session s, int id, string updateMsg, List<string> Images) |
|||
{ |
|||
Case c = await handler.GetCase(id); |
|||
if (c == null) throw new KeyNotFoundException("Case Id not found: " + id); |
|||
if (handler.IsAuthorized(s.Id, c)) |
|||
{ |
|||
foreach (string ImageId in Images) |
|||
{ |
|||
if (!await storage.ImageExists("test1", ImageId)) throw new InvalidOperationException(ImageId + " not found in storage!"); |
|||
} |
|||
if (c.CaseStatus == CaseStatus.Certified) throw new ArgumentException("Can't modify closed Case!"); |
|||
|
|||
if (s.Id == c.ParentId) c.Assignee = c.DoctorId; // Ha szülő updatel, az assignee az orvos lesz
|
|||
// TODO Ha a doki VAGY asszisztense frissít
|
|||
if (s.Id == c.DoctorId) c.Assignee = c.ParentId; // Ha doki frissít, a szülőhöz kerül
|
|||
|
|||
c.LastModificationDate = DateTime.Now; |
|||
Update update = new Update { |
|||
CaseId = c.Id, |
|||
SenderId = s.Id, |
|||
SenderRole = s.Type, |
|||
Content = updateMsg, |
|||
CreatedDate = DateTime.Now, |
|||
Images = Images |
|||
}; |
|||
await handler.UpdateCase(id, c, update); |
|||
} |
|||
else |
|||
{ |
|||
throw new UnauthorizedAccessException(); |
|||
} |
|||
} |
|||
} |
|||
} |
|||
using CoviDok.Api.Request; |
|||
using CoviDok.BLL.Sessions; |
|||
using CoviDok.BLL.Storage; |
|||
using CoviDok.BLL.User.Handlers; |
|||
using CoviDok.Data.Model; |
|||
using CoviDok.Data.MySQL; |
|||
using CoviDok.Data.StorageProviders; |
|||
using System; |
|||
using System.Collections.Generic; |
|||
using System.Linq; |
|||
using System.Linq.Expressions; |
|||
using System.Threading.Tasks; |
|||
|
|||
namespace CoviDok.BLL.User.Managers |
|||
{ |
|||
public class CaseManager |
|||
{ |
|||
private readonly ICaseHandler handler = new MySqlCaseHandler(); |
|||
|
|||
private readonly StorageHandler storage = new StorageHandler(new MinioProvider()); |
|||
|
|||
public async Task<List<Case>> FilterCases(Session s, CaseFilter filter) |
|||
{ |
|||
// TODO: Jogosultságkezelés
|
|||
return await handler.Filter(filter); |
|||
} |
|||
|
|||
public async Task<List<Update>> GetUpdatesForCase(Session s, int id) |
|||
{ |
|||
Case c = await handler.GetCase(id); |
|||
if (c == null) throw new KeyNotFoundException(); |
|||
if (handler.IsAuthorized(s.Id, c)) |
|||
{ |
|||
return handler.GetUpdatesForCase(id); |
|||
} |
|||
else throw new UnauthorizedAccessException(); |
|||
|
|||
} |
|||
|
|||
public async Task SetCertified(Session s, int id) |
|||
{ |
|||
Case c = await handler.GetCase(id); |
|||
if (c == null) throw new KeyNotFoundException(); |
|||
if (handler.IsAuthorized(s.Id, c) && s.Type == Api.Role.Ast) |
|||
{ |
|||
Update update = new Update { |
|||
CaseId = c.Id, |
|||
SenderId = s.Id, |
|||
SenderRole = s.Type, |
|||
CreatedDate = DateTime.Now, |
|||
Content = "Case set to 'Certified'" |
|||
}; |
|||
await handler.SetCase(id, CaseStatus.Certified, update); |
|||
} |
|||
else |
|||
{ |
|||
throw new UnauthorizedAccessException(); |
|||
} |
|||
} |
|||
|
|||
public async Task SetCured(Session s, int id) |
|||
{ |
|||
Case c = await handler.GetCase(id); |
|||
if (c == null) throw new KeyNotFoundException(); |
|||
if (s.Id == c.DoctorId) |
|||
{ |
|||
Update update = new Update |
|||
{ |
|||
CaseId = c.Id, |
|||
SenderId = s.Id, |
|||
SenderRole = s.Type, |
|||
CreatedDate = DateTime.Now, |
|||
Content = "Case set to 'Cured'" |
|||
}; |
|||
await handler.SetCase(id, CaseStatus.Cured, update); |
|||
} |
|||
else |
|||
{ |
|||
throw new UnauthorizedAccessException(); |
|||
} |
|||
} |
|||
|
|||
public async Task<Update> GetUpdate(Session s, int id) |
|||
{ |
|||
// Parent, Doctor, and doctors assistants can access a case
|
|||
Update u = handler.GetUpadte(id); |
|||
Case c = await handler.GetCase(u.CaseId); |
|||
if (c == null) throw new KeyNotFoundException(); |
|||
if (handler.IsAuthorized(s.Id, c)) |
|||
{ |
|||
return u; |
|||
} |
|||
else |
|||
{ |
|||
throw new UnauthorizedAccessException(); |
|||
} |
|||
} |
|||
|
|||
|
|||
|
|||
public async Task<Case> GetCase(Session s, int id) |
|||
{ |
|||
// Parent, Doctor, and doctors assistants can access a case
|
|||
Case c = await handler.GetCase(id); |
|||
if (c == null) throw new KeyNotFoundException(); |
|||
if (handler.IsAuthorized(s.Id, c)) |
|||
{ |
|||
return c; |
|||
} |
|||
else |
|||
{ |
|||
throw new UnauthorizedAccessException(); |
|||
} |
|||
} |
|||
|
|||
public async Task<Case> CreateCase(Session s, int DoctorId, int ChildId, string Title, DateTime startDate, Api.Priority priority) |
|||
{ |
|||
// TODO szülő csak saját gyereket jelenthet
|
|||
Case c = new Case { |
|||
StartDate = startDate, |
|||
ChildId = ChildId, |
|||
ParentId = s.Id, |
|||
DoctorId = DoctorId, |
|||
Title = Title, |
|||
CreatedDate = DateTime.Now, |
|||
LastModificationDate = DateTime.Now, |
|||
CaseStatus = CaseStatus.InProgress, |
|||
Assignee = s.Id, |
|||
Priority = priority |
|||
}; |
|||
return await handler.AddCase(c); |
|||
} |
|||
|
|||
public async Task UpdateCase(Session s, int id, string updateMsg, List<string> Images, Api.Priority priority) |
|||
{ |
|||
Case c = await handler.GetCase(id); |
|||
if (c == null) throw new KeyNotFoundException("Case Id not found: " + id); |
|||
if (handler.IsAuthorized(s.Id, c)) |
|||
{ |
|||
foreach (string ImageId in Images) |
|||
{ |
|||
if (!await storage.ImageExists("test1", ImageId)) throw new InvalidOperationException(ImageId + " not found in storage!"); |
|||
} |
|||
if (c.CaseStatus == CaseStatus.Certified) throw new ArgumentException("Can't modify closed Case!"); |
|||
|
|||
if (s.Id == c.ParentId) c.Assignee = c.DoctorId; // Ha szülő updatel, az assignee az orvos lesz
|
|||
// TODO Ha a doki VAGY asszisztense frissít
|
|||
if (s.Id == c.DoctorId) c.Assignee = c.ParentId; // Ha doki frissít, a szülőhöz kerül
|
|||
c.Priority = priority; |
|||
c.LastModificationDate = DateTime.Now; |
|||
Update update = new Update { |
|||
CaseId = c.Id, |
|||
SenderId = s.Id, |
|||
SenderRole = s.Type, |
|||
Content = updateMsg, |
|||
CreatedDate = DateTime.Now, |
|||
Images = Images |
|||
}; |
|||
await handler.UpdateCase(id, c, update); |
|||
} |
|||
else |
|||
{ |
|||
throw new UnauthorizedAccessException(); |
|||
} |
|||
} |
|||
} |
|||
} |
|||
|
@ -1,39 +1,42 @@ |
|||
using System; |
|||
using System.Collections.Generic; |
|||
using System.Linq; |
|||
using System.Threading.Tasks; |
|||
|
|||
namespace CoviDok.Data.Model |
|||
{ |
|||
public class Case |
|||
{ |
|||
public int Id { get; set; } |
|||
public int DoctorId { get; set; } |
|||
public int ParentId { get; set; } |
|||
public int ChildId { get; set; } |
|||
|
|||
public CaseStatus CaseStatus { get; set; } |
|||
|
|||
public ICollection<Update> Updates { get; set; } |
|||
|
|||
public int Assignee { get; set; } |
|||
|
|||
public string Title { get; set; } |
|||
public DateTime StartDate { get; set; } //amikor a tünetek kezdődtek
|
|||
public DateTime CreatedDate { get; set; } //amikor a taskot létrehozták
|
|||
public DateTime LastModificationDate { get; set; } |
|||
|
|||
public Case() |
|||
{ |
|||
Updates= new List<Update>(); |
|||
} |
|||
|
|||
} |
|||
|
|||
public enum CaseStatus |
|||
{ |
|||
InProgress, |
|||
Cured, |
|||
Certified |
|||
} |
|||
} |
|||
using CoviDok.Api; |
|||
using System; |
|||
using System.Collections.Generic; |
|||
using System.Linq; |
|||
using System.Threading.Tasks; |
|||
|
|||
namespace CoviDok.Data.Model |
|||
{ |
|||
public class Case |
|||
{ |
|||
public int Id { get; set; } |
|||
public int DoctorId { get; set; } |
|||
public int ParentId { get; set; } |
|||
public int ChildId { get; set; } |
|||
|
|||
public CaseStatus CaseStatus { get; set; } |
|||
|
|||
public Priority Priority { get; set; } |
|||
|
|||
public ICollection<Update> Updates { get; set; } |
|||
|
|||
public int Assignee { get; set; } |
|||
|
|||
public string Title { get; set; } |
|||
public DateTime StartDate { get; set; } //amikor a tünetek kezdődtek
|
|||
public DateTime CreatedDate { get; set; } //amikor a taskot létrehozták
|
|||
public DateTime LastModificationDate { get; set; } |
|||
|
|||
public Case() |
|||
{ |
|||
Updates= new List<Update>(); |
|||
} |
|||
|
|||
} |
|||
|
|||
public enum CaseStatus |
|||
{ |
|||
InProgress, |
|||
Cured, |
|||
Certified |
|||
} |
|||
} |
|||
|
Loading…
Reference in new issue