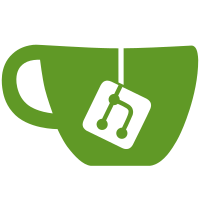
8 changed files with 272 additions and 3 deletions
@ -0,0 +1,14 @@ |
|||||
|
using CoviDok.data; |
||||
|
using System; |
||||
|
using System.Collections.Generic; |
||||
|
using System.Linq; |
||||
|
using System.Threading.Tasks; |
||||
|
|
||||
|
namespace CoviDok.Api.Request |
||||
|
{ |
||||
|
public class CaseFilter |
||||
|
{ |
||||
|
public string SessionID { get; set; } |
||||
|
public Dictionary<string, string> Filters = new Dictionary<string, string>(); |
||||
|
} |
||||
|
} |
@ -0,0 +1,14 @@ |
|||||
|
using System; |
||||
|
using System.Collections.Generic; |
||||
|
using System.Linq; |
||||
|
using System.Threading.Tasks; |
||||
|
|
||||
|
namespace CoviDok.Api.Request |
||||
|
{ |
||||
|
public class CaseUpdate |
||||
|
{ |
||||
|
public string CaseID { get; set; } |
||||
|
public string UpdateMsg { get; set; } |
||||
|
public string SessionID { get; set; } |
||||
|
} |
||||
|
} |
@ -0,0 +1,13 @@ |
|||||
|
using CoviDok.data; |
||||
|
using System; |
||||
|
using System.Collections.Generic; |
||||
|
using System.Linq; |
||||
|
using System.Threading.Tasks; |
||||
|
|
||||
|
namespace CoviDok.Api.Response |
||||
|
{ |
||||
|
public class FilteredCases |
||||
|
{ |
||||
|
public ICollection<Case> Cases = new List<Case>(); |
||||
|
} |
||||
|
} |
@ -0,0 +1,78 @@ |
|||||
|
using CoviDok.data; |
||||
|
using System; |
||||
|
using System.Collections.Generic; |
||||
|
using System.Linq; |
||||
|
using System.Threading.Tasks; |
||||
|
|
||||
|
namespace CoviDok.BLL |
||||
|
{ |
||||
|
public class Tools |
||||
|
{ |
||||
|
|
||||
|
private static Random random = new Random(); |
||||
|
public static string RandomString(int length) |
||||
|
{ |
||||
|
const string chars = "ABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789"; |
||||
|
return new string(Enumerable.Repeat(chars, length) |
||||
|
.Select(s => s[random.Next(s.Length)]).ToArray()); |
||||
|
} |
||||
|
|
||||
|
static T RandomEnumValue<T>() |
||||
|
{ |
||||
|
var v = Enum.GetValues(typeof(T)); |
||||
|
return (T)v.GetValue(random.Next(v.Length)); |
||||
|
} |
||||
|
|
||||
|
public static Case MockCase(Dictionary<string, string> filters) |
||||
|
{ |
||||
|
Case c = new Case |
||||
|
{ |
||||
|
Id = RandomString(8) |
||||
|
}; |
||||
|
if (filters.ContainsKey("DoctorID")) |
||||
|
{ |
||||
|
c.DoctorID = filters["DoctorID"]; |
||||
|
} |
||||
|
else |
||||
|
{ |
||||
|
c.DoctorID = Tools.RandomString(10); |
||||
|
} |
||||
|
if (filters.ContainsKey("ParentID")) |
||||
|
{ |
||||
|
c.ParentID = filters["ParentID"]; |
||||
|
} |
||||
|
else |
||||
|
{ |
||||
|
c.ParentID = Tools.RandomString(10); |
||||
|
} |
||||
|
if (filters.ContainsKey("ChildID")) |
||||
|
{ |
||||
|
c.ChildID = filters["ChildID"]; |
||||
|
} |
||||
|
else |
||||
|
{ |
||||
|
c.ChildID = Tools.RandomString(10); |
||||
|
} |
||||
|
if (filters.ContainsKey("CaseStatus")) |
||||
|
{ |
||||
|
c.ChildID = filters["CaseStatus"]; |
||||
|
} |
||||
|
else |
||||
|
{ |
||||
|
c.ChildID = RandomEnumValue<CaseStatus>().ToString(); |
||||
|
} |
||||
|
int msgDb = random.Next(10); |
||||
|
for (int i = 0; i < msgDb; i++) |
||||
|
{ |
||||
|
Update u = new Update |
||||
|
{ |
||||
|
Content = RandomString(55), |
||||
|
Sender = RandomString(13), |
||||
|
Id = RandomString(8) |
||||
|
}; |
||||
|
c.updates.Add(u); |
||||
|
} |
||||
|
return c; |
||||
|
} |
||||
|
} |
||||
|
} |
@ -0,0 +1,84 @@ |
|||||
|
using System; |
||||
|
using System.Collections; |
||||
|
using System.Collections.Generic; |
||||
|
using System.Linq; |
||||
|
using System.Threading.Tasks; |
||||
|
using CoviDok.Api; |
||||
|
using CoviDok.Api.Request; |
||||
|
using CoviDok.Api.Response; |
||||
|
using CoviDok.BLL; |
||||
|
using CoviDok.data; |
||||
|
using Microsoft.AspNetCore.Http; |
||||
|
using Microsoft.AspNetCore.Mvc; |
||||
|
|
||||
|
namespace CoviDok.Controllers |
||||
|
{ |
||||
|
[Route("api/[controller]")]
|
||||
|
[ApiController] |
||||
|
public class CaseController : ControllerBase |
||||
|
{ |
||||
|
// POST /api/Case/{id}
|
||||
|
[HttpGet("{id}")] |
||||
|
public async Task<ActionResult<Case>> GetCase(string id, AuthGet auth) |
||||
|
{ |
||||
|
if (auth.SessionID != "a") return Unauthorized(); |
||||
|
|
||||
|
List<Update> updates = new List<Update>(); |
||||
|
for (int i = 0; i < 10; i++) |
||||
|
{ |
||||
|
Update u = new Update |
||||
|
{ |
||||
|
Content = i.ToString(), |
||||
|
Sender = "Dr. A B", |
||||
|
Id = i.ToString() |
||||
|
}; |
||||
|
updates.Add(u); |
||||
|
} |
||||
|
Case c = new Case |
||||
|
{ |
||||
|
Id = "asasadf", |
||||
|
DoctorID = "hehegbvdesv", |
||||
|
ParentID = "qgwenhjegh", |
||||
|
ChildID = "egwbenbeb", |
||||
|
CaseStatus = CaseStatus.InProgress |
||||
|
}; |
||||
|
|
||||
|
return c; |
||||
|
} |
||||
|
|
||||
|
// POST /api/Case/{id}/update
|
||||
|
|
||||
|
[HttpPut("{id}/update")] |
||||
|
public async Task<IActionResult> PostUpdate(string id, CaseUpdate data) |
||||
|
{ |
||||
|
if (data.SessionID != "a") return Unauthorized(); |
||||
|
|
||||
|
return Ok(); |
||||
|
} |
||||
|
|
||||
|
// POST /api/Case/{id}/close
|
||||
|
[HttpPost("{i}/close")] |
||||
|
public async Task<IActionResult> PostClose(string id, CaseUpdate data) |
||||
|
{ |
||||
|
if (data.SessionID != "a") return Unauthorized(); |
||||
|
// if not doctor: unauthorized
|
||||
|
return Ok(); |
||||
|
} |
||||
|
|
||||
|
// POST /api/Case/filter
|
||||
|
public async Task<ActionResult<FilteredCases>> Filter(CaseFilter filters) |
||||
|
{ |
||||
|
if (filters.SessionID != "a") return Unauthorized(); |
||||
|
|
||||
|
FilteredCases cases = new FilteredCases(); |
||||
|
for (int i = 0; i < 10; i++) |
||||
|
{ |
||||
|
cases.Cases.Add(Tools.MockCase(filters.Filters)); |
||||
|
} |
||||
|
|
||||
|
return cases; |
||||
|
|
||||
|
} |
||||
|
|
||||
|
} |
||||
|
} |
@ -0,0 +1,66 @@ |
|||||
|
using System; |
||||
|
using System.Collections.Generic; |
||||
|
using System.Linq; |
||||
|
using System.Threading.Tasks; |
||||
|
using CoviDok.Api; |
||||
|
using CoviDok.Api.Request; |
||||
|
using Microsoft.AspNetCore.Http; |
||||
|
using Microsoft.AspNetCore.Mvc; |
||||
|
|
||||
|
namespace CoviDok.Controllers |
||||
|
{ |
||||
|
[Route("api/[controller]")]
|
||||
|
[ApiController] |
||||
|
public class DocController : ControllerBase |
||||
|
{ |
||||
|
// GET /api/Doc
|
||||
|
[HttpGet] |
||||
|
public async Task<ActionResult<GenericResponse>> GetDoctors() |
||||
|
{ |
||||
|
GenericResponse genericResponse = new GenericResponse |
||||
|
{ |
||||
|
Status = Status.Success |
||||
|
}; |
||||
|
for (int i=5; i < 15; i++) |
||||
|
{ |
||||
|
string doc = "{ \"FirstName\": \"Dr. Schanniquah\", \"LastName\": \"The " + i + "th\"}"; |
||||
|
genericResponse.Body[i.ToString()] = doc; |
||||
|
} |
||||
|
return genericResponse; |
||||
|
} |
||||
|
|
||||
|
// GET /api/Doc/{id}/assistants
|
||||
|
[HttpGet("{id}/assistants")] |
||||
|
public async Task<ActionResult<GenericResponse>> GetAssistantsOfDoctor(string id) |
||||
|
{ |
||||
|
GenericResponse genericResponse = new GenericResponse |
||||
|
{ |
||||
|
Status = Status.Success |
||||
|
}; |
||||
|
genericResponse.Body["DoctorID"] = id; |
||||
|
for (int i = 5; i < 15; i++) |
||||
|
{ |
||||
|
string doc = "{ \"FirstName\": \"Belisarius\", \"LastName\": \"The " + i + "th Cawl\"}"; |
||||
|
genericResponse.Body[i.ToString()] = doc; |
||||
|
} |
||||
|
return genericResponse; |
||||
|
} |
||||
|
|
||||
|
// GET /api/Doc/{id}/children
|
||||
|
[HttpGet("{id}/children")] |
||||
|
public async Task<ActionResult<GenericResponse>> GetChildrenOfDoctor(string id) |
||||
|
{ |
||||
|
GenericResponse genericResponse = new GenericResponse |
||||
|
{ |
||||
|
Status = Status.Success |
||||
|
}; |
||||
|
genericResponse.Body["DoctorID"] = id; |
||||
|
for (int i = 5; i < 15; i++) |
||||
|
{ |
||||
|
string doc = "{ \"FirstName\": \"Belisarius\", \"LastName\": \"The " + i + "th Cawl\"}"; |
||||
|
genericResponse.Body[i.ToString()] = doc; |
||||
|
} |
||||
|
return genericResponse; |
||||
|
} |
||||
|
} |
||||
|
} |
Loading…
Reference in new issue